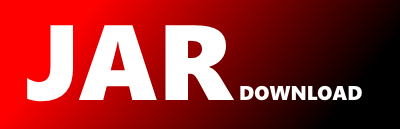
sorald.processor.EqualsOnAtomicClassProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sorald Show documentation
Show all versions of sorald Show documentation
An automatic repair system for static code analysis warnings from Sonar Java.
package sorald.processor;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicLong;
import sorald.annotations.ProcessorAnnotation;
import spoon.reflect.code.BinaryOperatorKind;
import spoon.reflect.code.CtBinaryOperator;
import spoon.reflect.code.CtExpression;
import spoon.reflect.code.CtInvocation;
import spoon.reflect.declaration.CtMethod;
import spoon.reflect.declaration.CtType;
import spoon.reflect.reference.CtExecutableReference;
@ProcessorAnnotation(
key = "S2204",
description = "\".equals()\" should not be used to test the values of \"Atomic\" classes")
public class EqualsOnAtomicClassProcessor extends SoraldAbstractProcessor {
@Override
protected void repairInternal(CtInvocation element) {
CtType atomicClass;
if (isAtomicInteger(element.getTarget())) {
atomicClass = getFactory().Class().get(AtomicInteger.class);
} else if (isAtomicLong(element.getTarget())) {
atomicClass = getFactory().Class().get(AtomicLong.class);
} else {
atomicClass = getFactory().Class().get(AtomicBoolean.class);
}
CtMethod ctMethodToBeCalled = (CtMethod) atomicClass.getMethodsByName("get").get(0);
CtExecutableReference ctExecutableReferenceToMethodToBeCalled =
getFactory().Executable().createReference(ctMethodToBeCalled);
CtInvocation leftInvocation =
getFactory()
.Code()
.createInvocation(
element.getTarget(), ctExecutableReferenceToMethodToBeCalled);
CtInvocation rightInvocation =
getFactory()
.Code()
.createInvocation(
(CtExpression) element.getArguments().get(0),
ctExecutableReferenceToMethodToBeCalled);
CtBinaryOperator newCtBinaryOperator =
getFactory()
.Code()
.createBinaryOperator(
leftInvocation, rightInvocation, BinaryOperatorKind.EQ);
element.replace(newCtBinaryOperator);
}
private boolean isAtomicClassRef(CtExpression ctExpression) {
return isAtomicInteger(ctExpression)
|| isAtomicLong(ctExpression)
|| isAtomicBoolean(ctExpression);
}
private boolean isAtomicInteger(CtExpression ctExpression) {
return ctExpression
.getType()
.getQualifiedName()
.equals("java.util.concurrent.atomic.AtomicInteger");
}
private boolean isAtomicLong(CtExpression ctExpression) {
return ctExpression
.getType()
.getQualifiedName()
.equals("java.util.concurrent.atomic.AtomicLong");
}
private boolean isAtomicBoolean(CtExpression ctExpression) {
return ctExpression
.getType()
.getQualifiedName()
.equals("java.util.concurrent.atomic.AtomicBoolean");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy