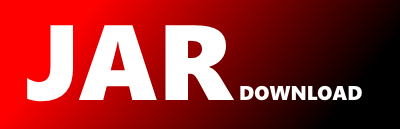
sorald.processor.ThreadLocalWithInitial Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sorald Show documentation
Show all versions of sorald Show documentation
An automatic repair system for static code analysis warnings from Sonar Java.
package sorald.processor;
import java.util.List;
import java.util.function.Supplier;
import sorald.annotations.ProcessorAnnotation;
import spoon.reflect.code.CtExpression;
import spoon.reflect.code.CtInvocation;
import spoon.reflect.code.CtLambda;
import spoon.reflect.code.CtNewClass;
import spoon.reflect.code.CtReturn;
import spoon.reflect.code.CtStatement;
import spoon.reflect.code.CtTypeAccess;
import spoon.reflect.declaration.CtClass;
import spoon.reflect.reference.CtExecutableReference;
import spoon.reflect.reference.CtTypeReference;
@ProcessorAnnotation(key = "S4065", description = "\"ThreadLocal.withInitial\" should be preferred")
public class ThreadLocalWithInitial extends SoraldAbstractProcessor> {
@Override
protected void repairInternal(CtNewClass> newClass) {
CtClass> innerClass = newClass.getAnonymousClass();
CtExecutableReference> initialValueMethod = findInitialValueMethod(innerClass);
CtLambda> lambda = createSupplier(initialValueMethod);
CtInvocation> invocation = createInitialMethod(newClass, lambda);
invocation.setArguments(List.of(lambda));
newClass.replace(invocation);
}
private CtInvocation> createInitialMethod(CtNewClass> threadLocal, CtLambda> lambda) {
CtTypeAccess
© 2015 - 2025 Weber Informatics LLC | Privacy Policy