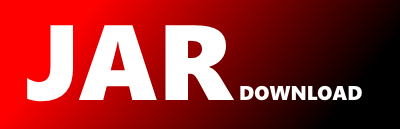
se.kth.iss.ug2.Ug2ObjectHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of standalone-ugclient Show documentation
Show all versions of standalone-ugclient Show documentation
A stand-alone Maven UgClient package separated from the UG server.
/*
* MIT License
*
* Copyright (c) 2017 Kungliga Tekniska högskolan
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package se.kth.iss.ug2;
public class Ug2ObjectHandle implements Comparable {
private final String kthid;
private final String ug2class;
private final boolean fuzzy;
public Ug2ObjectHandle(Ug2ObjectHandle ref) {
this.ug2class = ref.ug2class();
this.kthid = ref.kthid();
fuzzy = ref.fuzzy();
}
public Ug2ObjectHandle(String ug2class, String kthid) {
this.kthid = kthid;
this.ug2class = ug2class;
fuzzy = false;
}
public Ug2ObjectHandle(String ug2class, String kthid, boolean fuzzy) {
this.kthid = kthid;
this.ug2class = ug2class;
this.fuzzy = fuzzy;
}
public Ug2ObjectHandle(String value)
throws Ug2Exception {
String[] valueParts = value.split(":", 2);
if (valueParts.length != 2)
throw new Ug2Exception("Value is not an object reference: " + value, Ug2Protocol.STATUS_ILLEGALDATA);
this.ug2class = valueParts[0];
this.kthid = valueParts[1];
this.fuzzy = false;
}
/**
* @return The KTHID component of the object handle.
*/
public String kthid() {
return kthid;
}
/**
* @return The Ug2 Class component of the object handle.
*/
public String ug2class() {
return ug2class;
}
/**
* @return True if this ObjectHandle not necessary contains class/kthid but
* rather some other unique identifiers.
*/
public boolean fuzzy() {
return fuzzy;
}
public String toUg2Value() {
return ug2class + ":" + kthid;
}
/**
* @return True if the cmp principal instance contains the same principal
* data as this principal instance.
*/
@Override
public boolean equals(Object o) {
if(!(o instanceof Ug2ObjectHandle)) {
return false;
}
Ug2ObjectHandle cmp = (Ug2ObjectHandle) o;
return ug2class.equals(cmp.ug2class()) && kthid.equals(cmp.kthid());
}
@Override
public int hashCode() {
return ug2class.hashCode() ^ kthid.hashCode();
}
@Override
public int compareTo(Ug2ObjectHandle o) {
int retval = kthid.compareTo(o.kthid());
if (retval == 0)
retval = ug2class.compareTo(o.ug2class());
return retval;
}
/**
* @return A formatted String representing the value of this principal.
*/
@Override
public String toString() {
return ug2class + "/" + kthid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy