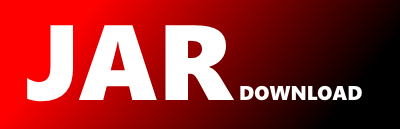
se.kth.iss.ug2.UgObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of standalone-ugclient Show documentation
Show all versions of standalone-ugclient Show documentation
A stand-alone Maven UgClient package separated from the UG server.
/*
* MIT License
*
* Copyright (c) 2017 Kungliga Tekniska högskolan
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package se.kth.iss.ug2;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* Basic class for representing a user or a group in UG. Contains all attributes
* that are supposed to be fetched for the UG class if they are set. Might also
* contain extra attributes.
*/
public class UgObject {
/**
* System wide definitions of valid UG classes.
*/
public static final String GROUP = "group";
public static final String USER = "user";
/**
* pseudoattribute for dealing with a users groupmemberships. Might be implemented
* as pseudoattributes in ug instead, since knowing a users group memberships
* is a very normal and common question.
*/
public static final String USERGROUPPSEUDOATTRIBUTE = "groupnames";
private final String kthid;
private final String ugClass;
private final Map ugAttributes = new HashMap<>();
private String lookupAttributeValue;
private String password;
/*
*This variable is for determining if this entry is qualified for propagation
*to the destination. It is false if the entry either is deleted/nonexisting
*in ug or it does not match the criterias for selective propagtion.
*/
private boolean notQualifiedForPropagation = true;
/**
* Default constructor for a UG object.
*
* @param ugClass should be either UgObject.USER or UgObject.GROUP
* @param kthid the kthid of the object.
* @param attributes the attributes.
* @param schema UG schema for attribute validation and singel value/multivalue knowledge.
* @throws Ug2Exception on errors.
*/
public UgObject(String ugClass, String kthid, Map> attributes, Ug2Schema schema)
throws Ug2Exception {
this.ugClass = ugClass;
this.kthid = kthid;
this.notQualifiedForPropagation = false;
for (Map.Entry> entry : attributes.entrySet()) {
if (!entry.getKey().equals(USERGROUPPSEUDOATTRIBUTE)) {
ugAttributes.put(entry.getKey(), new UgAttribute(entry.getKey(), entry.getValue(),
schema.multival(ugClass, entry.getKey())));
} else {
ugAttributes.put(entry.getKey(), new UgAttribute(entry.getKey(), entry.getValue(), true));
}
}
}
/**
* Constructor for a UG object that is created as a result of a lookup
*
* @param ugClass should be either UgObject.USER or UgObject.GROUP
* @param kthid the kthid of the object.
* @param attributes the attributes.
* @param schema UG schema for attribute validation and singel value/multivalue knowledge
* @param lookupAttributeValue value of attribute that was used to lookup this object
* @throws Ug2Exception on errors.
*/
public UgObject(String ugClass, String kthid, Map> attributes, Ug2Schema schema,
String lookupAttributeValue) throws Ug2Exception {
this.ugClass = ugClass;
this.kthid = kthid;
this.lookupAttributeValue = lookupAttributeValue;
this.notQualifiedForPropagation = false;
for (Map.Entry> entry : attributes.entrySet()) {
if (!entry.getKey().equals(USERGROUPPSEUDOATTRIBUTE)) {
ugAttributes.put(entry.getKey(), new UgAttribute(entry.getKey(), entry.getValue(),
schema.multival(ugClass, entry.getKey())));
} else {
ugAttributes.put(entry.getKey(), new UgAttribute(entry.getKey(), entry.getValue(), true));
}
}
}
/**
* Constructor for a UG object that is created locally to simulate a deleted object
*
* @param ugClass as defined in {@link UgObject}
* @param kthid the kthid of the object.
*/
public UgObject(String ugClass, String kthid) {
this.ugClass = ugClass;
this.notQualifiedForPropagation = true;
this.kthid = kthid;
}
/**
* Constructor for a UG object that is deleted in UG
*
* @param ugClass as defined in {@link UgObject}
* @param kthid the kthid of the object.
* @param lookupAttributeValue value of attribute that was used to lookup this object
*/
public UgObject(String ugClass, String kthid, String lookupAttributeValue) {
this.ugClass = ugClass;
this.kthid = kthid;
this.notQualifiedForPropagation = true;
this.lookupAttributeValue = lookupAttributeValue;
}
/**
* @return the attribute value used when this object was looked up
*/
public String getLookupAttributeValue() {
return lookupAttributeValue;
}
/**
* @param name the name of the attribute.
* @return the UG Attribute values
*/
public UgAttribute getUgAttribute(String name) {
return ugAttributes.get(name);
}
/**
* @return the kthid
*/
public String getKthid() {
return kthid;
}
/*
* Must not print the decrypted password!!!
*/
@Override
public String toString() {
return "class: " + ugClass + " kthid: " + kthid + " ugAttributes: " + ugAttributes;
}
/**
* Checks if this UG object contains the specified UG attribute.
*
* @param name the name of the attribute.
* @return true if object has given attribute.
*/
public boolean hasAttribute(String name) {
return ugAttributes.containsKey(name);
}
/**
* True if this UG object is deleted in UG or the object is not in the propagation set.
*
* @return true if deleted
*/
public boolean isDeleted() {
return this.notQualifiedForPropagation;
}
/**
* Get the UG class of this object.
*
* @return UgObject.USER or UgObject.GROUP
*/
public String getUgClass() {
return ugClass;
}
/**
* @return list of all multivalued attributes.
*/
public List getMultiValueAttributes() {
return ugAttributes.keySet().stream().filter(attributeName -> ugAttributes.get(attributeName).isMultiVal())
.collect(Collectors.toCollection(LinkedList::new));
}
/**
* True if ugClass is UgObject.USER
*
* @param ugClass the class to check.
* @return True if ugClass is UgObject.USER
*/
public static boolean isGroup(String ugClass) {
return ugClass.equals(GROUP);
}
/**
* True if ugClass is UgObject.GROUP
*
* @param ugClass the class to check.
* @return True if ugClass is UgObject.GROUP
*/
public static boolean isUser(String ugClass) {
return ugClass.equals(USER);
}
/**
* Check if this is a group.
*
* @return true if this is a UG group
*/
public boolean isGroup() {
return isGroup(ugClass);
}
/**
* Check if this is a user.
*
* @return true if this is a UG user
*/
public boolean isUser() {
return isUser(ugClass);
}
/**
* Used for setting the decrypted password.
*
* @param password the decrypted password
*/
public void setPassword(String password) {
this.password = password;
}
/**
* Retrieve the decrypted password.
*
* @return decrypted password
*/
public String getPassword() {
return password;
}
/**
* Passwords are a special UG attribute and this is only true if the
* password has been set since the last time this UG user was synced or when
* the user is created.
*
* @return true if the object includes new password data.
*/
public boolean hasPassword() {
return password != null;
}
public void setDeleted() {
notQualifiedForPropagation = true;
}
public static boolean isValidKthid(String kthid, String ugClass) throws Ug2Exception {
if (kthid.length() != 8) {
return false;
}
if (isUser(ugClass)) {
return kthid.startsWith("u1");
}
if (isGroup(ugClass)) {
return kthid.startsWith("u2");
}
throw new Ug2Exception("UG class is neither user nor group:" + ugClass);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy