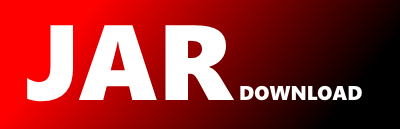
se.kth.iss.ug2.UgWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of standalone-ugclient Show documentation
Show all versions of standalone-ugclient Show documentation
A stand-alone Maven UgClient package separated from the UG server.
/*
* MIT License
*
* Copyright (c) 2017 Kungliga Tekniska högskolan
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package se.kth.iss.ug2;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* This interface is for wrapping ug2clients in order to get an Iterable with
* prefetching of a suitable number of ugobjects in each turn. There are also
* a few conveniece methods.
*/
public interface UgWrapper extends AutoCloseable {
/**
* Used for iterating over a large amount of kthids, getting the ug
* data for each of them and turning them into ugobjects
*
* @param ugClass as defined in UgObject
* @param keyAttribute the key for the lookup, "kthid" in most cases.
* @param keys a list of kthids
* @param attributeNames the names of the attributes to fetch.
* @return an iterable of ugobjects.
*/
public Iterable getData(String ugClass, String keyAttribute, List keys, Set attributeNames);
/**
* Used for iterating over a large amount of kthids, getting the ug
* data for each of them and turning them into ugobjects
*
* @param ugClass as defined in UgObject
* @param keyAttribute the key for the lookup, "kthid" in most cases.
* @param keys an array of kthids
* @param attributeNames the names of the attributes to fetch.
* @return an iterable of ugobjects.
*/
public Iterable getData(String ugClass, String keyAttribute, String[] keys, Set attributeNames);
/**
* Get the ug data for one ug object.
*
* @param ugClass as defined in UgObject
* @param keyAttribute the key for the lookup, "kthid" in most cases.
* @param key a kthid
* @param attributeNames the names of the attributes to fetch.
* @return an ugobject.
*/
public UgObject getData(String ugClass, String keyAttribute, String key, Set attributeNames);
/**
* Get a single attribute for a specific object.
*
* @param ugClass the class of the object
* @param keyAttribute which attribute to use for identifying the object
* @param key the value used to identify the object
* @param attributeName the attribute to get
* @return the wanted attribute value.
*/
public UgAttribute getAttribute(String ugClass, String keyAttribute, String key, String attributeName);
/**
* returns the current ug version
*
* @return current ug version
* @throws Ug2Exception on errors.
*/
public long currentVersion() throws Ug2Exception;
/**
* Gets all existing kthids in the given UG class.
*
* @param ugClass as defined in UgObject
* @return array of all kthids.
* @throws Ug2Exception on errors.
*/
public String[] getAllKthids(String ugClass)
throws Ug2Exception;
/**
* Gets all direct and indirect members of all the groups matching the
* SQL wildcard expressions in groupnamepatterns.
*
* @param groupNamePatterns A collection of group name patterns as SQL wildcard patterns
* @return kthids of all direct and indirect members
* @throws Ug2Exception on errors.
*/
public Set getAllUserMembers(Collection groupNamePatterns)
throws Ug2Exception;
/**
* Gets all groups and their group memmbers matching the
* SQL wildcard expressions in groupnamepatterns.
*
* @param groupNamePatterns A collection of group name patterns as SQL wildcard patterns
* @return kthids of all groups with matching names and their group members
* @throws Ug2Exception on errors.
*/
public Set getAllGroups(Collection groupNamePatterns)
throws Ug2Exception;
/**
* Gets all the changed UG objects since version and put their kthids in the
* result hash map.
*
* @param version the start version
* @param result used for returning a map of ugclass and a set of kthids that has been modified since verison..
* @return current ug version
* @throws Ug2Exception on errors.
*/
public long getChangedKthids(long version,
Map> result) throws Ug2Exception;
/**
* Check that we have a working connection. Reconnect if necessary.
*
* @throws Ug2Exception on errors.
*/
void checkConnection() throws Ug2Exception;
/**
* reconnect to the UG server.
*
* @throws Ug2Exception on errors.
*/
public void reconnect() throws Ug2Exception;
/**
* Wrapper for setData() to use when changing singleval attributes.
*
* @param className "user" or "group"
* @param key attribute to use as key to identify object.
* @param object value for key
* @param attribute attribute to set
* @param value single value to set attribute to
* @throws Ug2Exception on errors.
*/
public void setData(String className, String key, String object, String attribute, String value)
throws Ug2Exception;
/**
* Wrapper for setData() that uses Collection.
*
* @param className "user" or "group"
* @param key attribute to use as key to identify object.
* @param object value for key
* @param attribute attribute to set
* @param values Collection of values to set attribute to
* @throws Ug2Exception on errors.
*/
public void setData(String className, String key, String object, String attribute, Collection values)
throws Ug2Exception;
/**
* Wrapper for terminateSession.
*/
public void terminateSession();
public Ug2Client getUgclient();
/**
* Fetches kthids for direct members of any of the specified groups.
*
* @param groups kthids for the groups
* @return set of kthids which are members of these groups.
* @throws Ug2Exception on errors.
*/
public Set getMembers(Collection groups) throws Ug2Exception;
/**
* Sets the property that the propagator should create events for users when
* their membership changes.
*
* @param getUsersOnMembershipChanges true if partial propagator
*/
public void setGetUsersOnMembershipChanges(boolean getUsersOnMembershipChanges);
/**
* Get all indirect memberships of a ug object.
*
* @param ugobject to get indirect memberships for
* @return set of all group names
* @throws Ug2Exception on errors.
*/
public Set getAllIndirectMemberships(UgObject ugobject) throws Ug2Exception;
/**
* Fills the supplied {@link Map} with the kthids of the objects that has
* had any of the attributes specified in a supplied {@link Map} of
* attributes changed since a specified UG version. Both maps uses UG class as key.
*
* @param ugVersion the UG version to start checking from.
* @param changedKthidsMap gets filled with changed kthids mapped by UG class.
* @param watchedAttributes attributes mapped by UG class.
* @return current UG version if more results are potentially available and
* the method should be called again, -1 otherwise.
* @throws Ug2Exception if an error occurs while retrieving change log
* entries.
*/
public long getChangedKthids(long ugVersion, Map> changedKthidsMap, Map> watchedAttributes) throws Ug2Exception;
/**
* Get the distinct set of groups matching the given SQL wildcard patterns.
*
* @param patterns the SQL wildcard patterns matching the u1names of the groups.
* @param attributes list of attributes to fetch for groups.
* @return all groups having a u1name matching one of the wildcard patterns.
* @throws Ug2Exception on errors.
*/
public List getGroupsMatching(Collection patterns, List attributes) throws Ug2Exception;
/**
* Gets a list of Ug2Users complete with requested attribute data.
*
* @param kthids the kthids of the Ug2Users to retrieve
* @param attributeNames the attribute names who's value is required
* @return the list of Ug2Users
* @throws Ug2Exception if data can't be retrieved.
*/
public List getUserData(List kthids, List attributeNames) throws Ug2Exception;
/**
* Gets an Ug2User complete with requested attribute data.
*
* @param kthid the kthid of the Ug2User to retrieve
* @param attributeNames the attribute names who's value is required
* @return the Ug2User
* @throws Ug2Exception if data can't be retrieved.
*/
public Ug2User getUserData(String kthid, List attributeNames) throws Ug2Exception;
/**
* Get the social security number (personnummer) of a user.
* @param kthid the kthid of the user.
* @return the social security number or null if user was not found.
* @throws Ug2Exception on errors.
*/
public String getPnr(String kthid) throws Ug2Exception;
/**
* Gets a list of Ug2Groups complete with requested attribute data.
*
* @param kthids the kthids of the Ug2Groups to retrieve
* @param attributeNames the attribute names who's value is required
* @return the list of Ug2Users
* @throws Ug2Exception if data can't be retrieved.
*/
public List getGroupData(List kthids, List attributeNames) throws Ug2Exception;
public void setPartitionSize(int partitionSize);
public List getUsers() throws Ug2Exception;
/**
* Get all users in UG
*
* @return the list of users
* @throws Ug2Exception if the list can't be retrieved
*/
List getAllUsers() throws Ug2Exception;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy