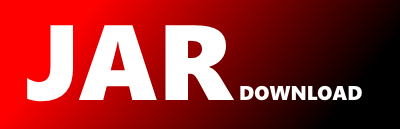
se.l4.silo.FetchResult Maven / Gradle / Ivy
The newest version!
package se.l4.silo;
import java.util.Collection;
import java.util.Iterator;
import java.util.Optional;
import java.util.Spliterator;
import java.util.function.Function;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import com.google.common.collect.Iterators;
import se.l4.silo.results.EmptyFetchResult;
import se.l4.silo.results.FetchResultSpliterator;
import se.l4.silo.results.IteratorFetchResult;
import se.l4.silo.results.TransformingFetchResult;
/**
* Result of a fetch request, contains the results and information about the
* parameters used for fetching.
*
* @author Andreas Holstenson
*
* @param
*/
public interface FetchResult
extends AutoCloseable, Iterable
{
/**
* Get the number of returned results.
*
* @return
*/
long getSize();
/**
* Get the offset used to fetch these results.
*
* @return
*/
long getOffset();
/**
* Get the limit used to fetch these results.
*
* @return
*/
long getLimit();
/**
* Get the total number of results available.
*
* @return
*/
long getTotal();
/**
* Check if these results are empty.
*
* @return
*/
boolean isEmpty();
/**
* Get the first entry is this result.
*
* @return
*/
default Optional first()
{
Iterator it = iterator();
if(it.hasNext())
{
return Optional.of(it.next());
}
return Optional.empty();
}
@Override
void close();
/**
* Transform this fetch result using the given function.
*
* @param func
* @return
*/
default FetchResult transform(Function func)
{
return new TransformingFetchResult(this, func);
}
@Override
default Spliterator spliterator()
{
return new FetchResultSpliterator(this);
}
/**
* Get a {@link Stream} for this result, see {@link Collection#stream()}
* for details. The returned stream will close this result when it itself
* is closed.
*
* @return
*/
default Stream stream()
{
Stream stream = StreamSupport.stream(spliterator(), false);
stream.onClose(this::close);
return stream;
}
/**
* Get a {@link Stream} for this result for parallel operations,
* see {@link Collection#parallelStream()} for details.
* The returned stream will close this result when it itself is closed.
*
* @return
*/
default Stream parallelStream()
{
return StreamSupport.stream(spliterator(), true);
}
/**
* Get an empty fetch result.
*
* @return
*/
@SuppressWarnings("unchecked")
static FetchResult empty()
{
return (FetchResult) EmptyFetchResult.INSTANCE;
}
/**
* Get a {@link FetchResult} representing the fetch of a single item.
*
* @param data
* @return
*/
static FetchResult single(N data)
{
return new IteratorFetchResult<>(Iterators.singletonIterator(data), 1, 0, -1, 1);
}
/**
* Get a {@link FetchResult} for the given collection. This will assume
* that the offset and limit used to fetch this was zero.
*
* @param collection
* @return
*/
static FetchResult forCollection(Collection collection)
{
return new IteratorFetchResult<>(collection, 0, 0, collection.size());
}
/**
* Get a {@link FetchResult} for the given collection. This will assume
* that the offset and limit used to fetch this was zero.
*
* @param collection
* @return
*/
static FetchResult forCollection(Collection collection, int offset, int limit, int hits)
{
if(hits < collection.size())
{
throw new IllegalArgumentException("hits must be larger than or equal to collection size (" + hits + " < " + collection.size() + ")");
}
return new IteratorFetchResult<>(collection, offset, limit, hits);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy