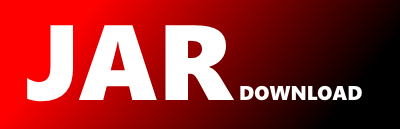
se.l4.silo.Silo Maven / Gradle / Ivy
package se.l4.silo;
import java.io.Closeable;
import java.util.function.Supplier;
import se.l4.silo.binary.BinaryEntity;
import se.l4.silo.structured.StructuredEntity;
/**
* Silo storage, provides access to all entities stored, transactions and
* resource management. An instance of this class contains {@link Entity entities}
* that can be used to store and retrieve data.
*
* Transactions
*
* Silo provides transaction support. In normal usage every write operation is
* a tiny transaction, so a store operation will internally be mapped against a
* transaction. Transactions in Silo are implemented so that they become
* readable when they are committed, so any changes made in a transaction
* are not visible either within our outside the transaction.
*
*
Using {@link #newTransaction()}
*
* When {@link #newTransaction()} is called a transaction is activated for the
* current thread. Transactions must either be committed or
* rolled back when they are used.
*
*
* Example:
*
* Transaction tx = silo.newTransaction();
* try {
* // Perform operations as usual here
* entity.store("test id", object);
*
* tx.commit();
* } catch(Throwable t) {
* tx.rollback();
* }
*
*
* Using {@link #inTransaction(Runnable)} and {@link #inTransaction(Supplier)}
*
* An alternative way of using transactions is provided via {@link #inTransaction(Runnable)}
* and {@link #inTransaction(Supplier)}. These will take care of committing
* and rolling back a transaction.
*
*
* Examples:
*
* silo.inTransaction(() -> {
* entity.store("test id", object);
* });
*
*
* @author Andreas Holstenson
*
*/
public interface Silo
extends Closeable
{
/**
* Create a new instance of the specified interface for usage with the
* storage.
*
* @param siloInterface
* @return
*/
T create(Class siloInterface);
/**
* Check if the given entity is available.
*
* @param entityName
* @return
*/
boolean hasEntity(String entityName);
/**
* Get an entity of the given type.
*
* @param entityName
* @param type
*/
T entity(String entityName, Class type);
/**
* Get access to the binary entity with the given name.
*
* @param entityName
* @return
*/
BinaryEntity binary(String entityName);
/**
* Get an entity used for storing structured data with the given name.
*
* @param entityName
* @return
*/
StructuredEntity structured(String entityName);
/**
* Create a new transaction for the current thread.
*
* @return
*/
Transaction newTransaction();
/**
* Acquire a new resource lock for the current thread.
*
* @return
*/
ResourceHandle acquireResourceHandle();
/**
* Run the given {@link Supplier} in a transaction.
*
* @param supplier
* @return
*/
default T inTransaction(Supplier supplier)
{
StorageTransactionException firstException = null;
for(int i=0, n=5; i