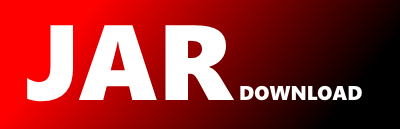
se.l4.vibe.probes.SamplerProbes Maven / Gradle / Ivy
package se.l4.vibe.probes;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.TimeUnit;
import se.l4.vibe.probes.Sampler.Entry;
/**
* General probes that work with {@link Sampler sampler}.
*
* @author Andreas Holstenson
*
*/
public class SamplerProbes
{
private SamplerProbes()
{
}
/**
* Create a probe for the specified sampler that will keep track of values
* over the specified time.
*
* @param series
* @param duration
* @param unit
* @param operation
* @return
*/
public static Probe
© 2015 - 2025 Weber Informatics LLC | Privacy Policy