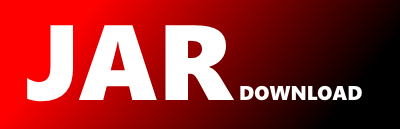
se.l4.vibe.influxdb.InfluxDBBackend Maven / Gradle / Ivy
package se.l4.vibe.influxdb;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ThreadFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import okhttp3.HttpUrl;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import se.l4.vibe.backend.VibeBackend;
import se.l4.vibe.event.EventListener;
import se.l4.vibe.event.EventSeverity;
import se.l4.vibe.event.Events;
import se.l4.vibe.influxdb.internal.DataPoint;
import se.l4.vibe.influxdb.internal.DataQueue;
import se.l4.vibe.mapping.KeyValueMappable;
import se.l4.vibe.mapping.KeyValueReceiver;
import se.l4.vibe.probes.Probe;
import se.l4.vibe.probes.SampleListener;
import se.l4.vibe.probes.SampledProbe;
import se.l4.vibe.probes.Sampler;
import se.l4.vibe.timer.Timer;
import se.l4.vibe.timer.TimerListener;
/**
* {@link VibeBackend Backend} that sends data to InfluxDB.
*
* @author Andreas Holstenson
*
*/
public class InfluxDBBackend
implements VibeBackend
{
private static final Logger logger = LoggerFactory.getLogger(InfluxDBBackend.class);
private static final MediaType MEDIA_TYPE = MediaType.parse("text/plain");
private final String url;
private final String auth;
private final Map tags;
private final OkHttpClient client;
private final DataQueue queue;
private final ScheduledExecutorService executor;
private InfluxDBBackend(String url, String username, String password, String db, Map tags)
{
this.tags = tags;
client = new OkHttpClient();
this.url = HttpUrl.parse(url).newBuilder()
.addPathSegment("write")
.addQueryParameter("db", db)
.addQueryParameter("precision", "ms")
.build()
.toString();
if(username != null)
{
auth = "Basic " + Base64.getMimeEncoder().encodeToString((username + ':' + password).getBytes(StandardCharsets.UTF_8));
}
else
{
auth = null;
}
executor = Executors.newScheduledThreadPool(1, new ThreadFactory()
{
@Override
public Thread newThread(Runnable r)
{
Thread thread = new Thread(r);
thread.setName("InfluxDB[" + url + "]");
return thread;
}
});
queue = new DataQueue(this::send, executor);
}
private void send(String data)
{
Request.Builder builder = new Request.Builder()
.url(url)
.post(RequestBody.create(MEDIA_TYPE, data));
if(auth != null)
{
builder.addHeader("Authorization", auth);
}
Request request = builder.build();
try
{
Response response = client.newCall(request)
.execute();
response.body().close();
if(response.code() < 200 || response.code() >= 300)
{
logger.warn("Unable to store values; Got response code " + response.code());
throw new RuntimeException("Failed sending");
}
}
catch(IOException e)
{
logger.warn("Unable to store values; " + e.getMessage(), e);
throw new RuntimeException("Failed sending; " + e.getMessage(), e);
}
}
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void export(String path, Sampler> sampler)
{
((Sampler) sampler).addListener(new SampleQueuer(path));
}
@Override
public void export(String path, Probe> probe)
{
}
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void export(String path, Events> events)
{
((Events) events).addListener(new EventQueuer(path));
}
@Override
public void export(String path, Timer timer)
{
timer.addListener(new TimerQueuer(path));
}
@Override
public void close()
{
queue.close();
executor.shutdown();
}
private class SampleQueuer
implements SampleListener
© 2015 - 2025 Weber Informatics LLC | Privacy Policy