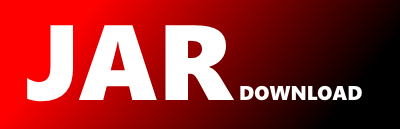
se.laz.casual.jca.CasualManagedConnectionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of casual-jca Show documentation
Show all versions of casual-jca Show documentation
Casual JCA - Resource Adapter for Casual middleware.
/*
* Copyright (c) 2017 - 2018, The casual project. All rights reserved.
*
* This software is licensed under the MIT license, https://opensource.org/licenses/MIT
*/
package se.laz.casual.jca;
import se.laz.casual.network.ProtocolVersion;
import jakarta.resource.ResourceException;
import jakarta.resource.spi.CommException;
import jakarta.resource.spi.ConnectionManager;
import jakarta.resource.spi.ConnectionRequestInfo;
import jakarta.resource.spi.ManagedConnection;
import jakarta.resource.spi.ManagedConnectionFactory;
import jakarta.resource.spi.ResourceAdapter;
import jakarta.resource.spi.ResourceAdapterAssociation;
import jakarta.resource.spi.ValidatingManagedConnectionFactory;
import javax.security.auth.Subject;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.logging.Logger;
import java.util.stream.Collectors;
/**
* CasualManagedConnectionFactory
*
* @version $Revision: $
*/
//Non serialisable or transient for ResourceAdapter and PrintWriter - this is as shown in Iron Jacamar so ignoring.
@SuppressWarnings("squid:S1948")
public class CasualManagedConnectionFactory implements ManagedConnectionFactory, ResourceAdapterAssociation, ValidatingManagedConnectionFactory
{
private static final long serialVersionUID = 1L;
private static Logger log = Logger.getLogger(CasualManagedConnectionFactory.class.getName());
private CasualManagedConnectionProducer casualManagedConnectionProducer;
private ResourceAdapter ra;
private PrintWriter logwriter;
private String hostName;
private Integer portNumber;
private Long casualProtocolVersion = 1000L;
private String networkConnectionPoolName;
private Integer networkConnectionPoolSize;
private final int resourceId = CasualResourceManager.getInstance().getNextId();
public CasualManagedConnectionFactory()
{
this.casualManagedConnectionProducer = CasualManagedConnection::new;
}
public String getHostName()
{
return hostName;
}
public void setHostName(String hostName)
{
this.hostName = hostName;
}
public Integer getPortNumber()
{
return portNumber;
}
public void setPortNumber(Integer portNumber)
{
this.portNumber = portNumber;
}
public ProtocolVersion getCasualProtocolVersion()
{
return ProtocolVersion.unmarshall(casualProtocolVersion);
}
public CasualManagedConnectionFactory setCasualProtocolVersion(Long casualProtocolVersion)
{
Objects.requireNonNull(casualProtocolVersion, "casual protocol version can not be null!");
this.casualProtocolVersion = casualProtocolVersion;
return this;
}
public String getNetworkConnectionPoolName()
{
return networkConnectionPoolName;
}
public void setNetworkConnectionPoolName(String networkConnectionPoolName)
{
this.networkConnectionPoolName = networkConnectionPoolName;
}
public Integer getNetworkConnectionPoolSize()
{
return networkConnectionPoolSize;
}
public void setNetworkConnectionPoolSize(Integer networkConnectionPoolSize)
{
this.networkConnectionPoolSize = networkConnectionPoolSize;
}
@Override
public Object createConnectionFactory(ConnectionManager cxManager) throws ResourceException
{
log.finest("createConnectionFactory()");
return new CasualConnectionFactoryImpl(this, cxManager);
}
@Override
public Object createConnectionFactory() throws ResourceException
{
throw new ResourceException("This resource adapter doesn't support non-managed environments");
}
@Override
public ManagedConnection createManagedConnection(Subject subject,
ConnectionRequestInfo cxRequestInfo) throws ResourceException
{
try
{
CasualManagedConnection managedConnection = casualManagedConnectionProducer.createManagedConnection(this);
log.finest(() -> "Created a new managed connection: " + managedConnection);
return managedConnection;
}
catch(Exception e)
{
StringWriter writer = new StringWriter();
PrintWriter printWriter = new PrintWriter( writer );
e.printStackTrace(printWriter);
printWriter.flush();
log.warning(() -> "createManagedConnection failed: " + writer);
throw new CommException(e);
}
}
@Override
@SuppressWarnings({"rawtypes","unchecked"})
public ManagedConnection matchManagedConnections(Set connectionSet,
Subject subject, ConnectionRequestInfo cxRequestInfo) throws ResourceException
{
log.finest("matchManagedConnections()");
return (ManagedConnection)connectionSet.stream()
.filter(CasualManagedConnection.class::isInstance)
.findFirst( )
.orElse( null );
}
@Override
@SuppressWarnings({"rawtypes","unchecked"})
public Set getInvalidConnections(Set connectionSet) throws ResourceException
{
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy