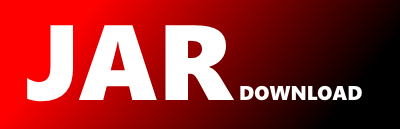
se.michaelthelin.spotify.model_objects.specification.Error Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotify-web-api-java Show documentation
Show all versions of spotify-web-api-java Show documentation
A Java client for Spotify's Web API
package se.michaelthelin.spotify.model_objects.specification;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.gson.JsonObject;
import se.michaelthelin.spotify.model_objects.AbstractModelObject;
/**
* Retrieve information about
* Error objects by building instances from this class.
*/
@JsonDeserialize(builder = Error.Builder.class)
public class Error extends AbstractModelObject {
private final Integer status;
private final String message;
private Error(final Builder builder) {
super(builder);
this.status = builder.status;
this.message = builder.message;
}
/**
* Get the HTTP status code
* of the {@link Error} object.
*
* @return The HTTP status code.
*/
public Integer getStatus() {
return status;
}
/**
* Get the error message (description of the cause) of the {@link Error} object.
*
* @return A short description of the cause of the error.
*/
public String getMessage() {
return message;
}
@Override
public String toString() {
return "Error(status=" + status + ", message=" + message + ")";
}
@Override
public Builder builder() {
return new Builder();
}
/**
* Builder class for building {@link Error} instances.
*/
public static final class Builder extends AbstractModelObject.Builder {
private Integer status;
private String message;
/**
* The error HTTP status
* code setter.
*
* @param status The
* HTTP status code.
* @return A {@link Error.Builder}.
*/
public Builder setStatus(Integer status) {
this.status = status;
return this;
}
/**
* The error message setter.
*
* @param message A short description of the cause of the error.
* @return A {@link Error.Builder}.
*/
public Builder setMessage(String message) {
this.message = message;
return this;
}
@Override
public Error build() {
return new Error(this);
}
}
/**
* JsonUtil class for building {@link Error} instances.
*/
public static final class JsonUtil extends AbstractModelObject.JsonUtil {
public Error createModelObject(JsonObject jsonObject) {
if (jsonObject == null || jsonObject.isJsonNull()) {
return null;
}
return new Error.Builder()
.setStatus(
hasAndNotNull(jsonObject, "status")
? jsonObject.get("status").getAsInt()
: null)
.setMessage(
hasAndNotNull(jsonObject, "message")
? jsonObject.get("message").getAsString()
: null)
.build();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy