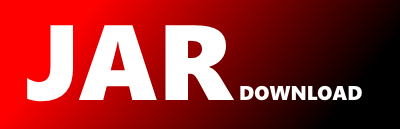
se.michaelthelin.spotify.model_objects.special.SnapshotResult Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package se.michaelthelin.spotify.model_objects.special;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.gson.JsonObject;
import se.michaelthelin.spotify.model_objects.AbstractModelObject;
/**
* Retrieve information about Snapshot Result objects by building instances from this class. These objects contain a
* playlist snapshot ID, which is created after adding or removing tracks from a playlist.
*
*
* Spotify: Working With Playlists
*/
@JsonDeserialize(builder = SnapshotResult.Builder.class)
public class SnapshotResult extends AbstractModelObject {
private final String snapshotId;
private SnapshotResult(final Builder builder) {
super(builder);
this.snapshotId = builder.snapshotId;
}
/**
* Get the snapshot ID.
*
* @return The snapshot ID.
*/
public String getSnapshotId() {
return snapshotId;
}
@Override
public String toString() {
return "SnapshotResult(snapshotId=" + snapshotId + ")";
}
@Override
public Builder builder() {
return new Builder();
}
/**
* Builder class for building {@link SnapshotResult} instances.
*/
public static final class Builder extends AbstractModelObject.Builder {
public String snapshotId;
public Builder setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
@Override
public SnapshotResult build() {
return new SnapshotResult(this);
}
}
/**
* JsonUtil class for building {@link SnapshotResult} instances.
*/
public static final class JsonUtil extends AbstractModelObject.JsonUtil {
public SnapshotResult createModelObject(JsonObject jsonObject) {
if (jsonObject == null || jsonObject.isJsonNull()) {
return null;
}
return new SnapshotResult.Builder()
.setSnapshotId(
hasAndNotNull(jsonObject, "snapshot_id")
? jsonObject.get("snapshot_id").getAsString()
: null)
.build();
}
}
}