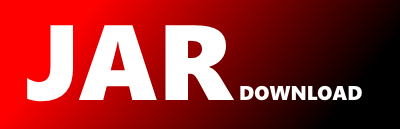
se.michaelthelin.spotify.requests.data.personalization.simplified.GetUsersTopTracksRequest Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package se.michaelthelin.spotify.requests.data.personalization.simplified;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import org.apache.hc.core5.http.ParseException;
import se.michaelthelin.spotify.exceptions.SpotifyWebApiException;
import se.michaelthelin.spotify.model_objects.specification.Paging;
import se.michaelthelin.spotify.model_objects.specification.Track;
import se.michaelthelin.spotify.requests.data.AbstractDataPagingRequest;
import se.michaelthelin.spotify.requests.data.AbstractDataRequest;
import java.io.IOException;
/**
* Get the current user’s top tracks based on calculated affinity.
*
* Affinity is a measure of the expected preference a user has for a particular track or artist. It is based on user
* behavior, including play history, but does not include actions made while in incognito mode.
* Light or infrequent users of Spotify may not have sufficient play history to generate a full affinity data set.
*
* As a user’s behavior is likely to shift over time, this preference data is available over three time spans. See
* {@link Builder#time_range(String)} for more information.
*
* For each time range, the top 50 tracks and artists are available for each user. In the future, it is likely that this
* restriction will be relaxed. This data is typically updated once each day for each user.
*/
@JsonDeserialize(builder = GetUsersTopTracksRequest.Builder.class)
public class GetUsersTopTracksRequest extends AbstractDataRequest> {
/**
* The private {@link GetUsersTopTracksRequest} constructor.
*
* @param builder A {@link GetUsersTopTracksRequest.Builder}.
*/
private GetUsersTopTracksRequest(final Builder builder) {
super(builder);
}
/**
* Get an user's top tracks.
*
* @return An user's top tracks.
* @throws IOException In case of networking issues.
* @throws SpotifyWebApiException The Web API returned an error further specified in this exception's root cause.
*/
public Paging