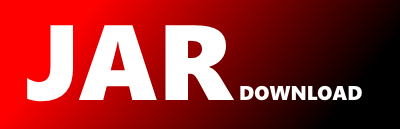
se.michaelthelin.spotify.model_objects.special.SearchResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotify-web-api-java Show documentation
Show all versions of spotify-web-api-java Show documentation
A Java client for Spotify's Web API
package se.michaelthelin.spotify.model_objects.special;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.gson.JsonObject;
import se.michaelthelin.spotify.model_objects.AbstractModelObject;
import se.michaelthelin.spotify.model_objects.specification.*;
import se.michaelthelin.spotify.requests.data.personalization.interfaces.IArtistTrackModelObject;
import se.michaelthelin.spotify.requests.data.search.SearchItemRequest;
import se.michaelthelin.spotify.requests.data.search.interfaces.ISearchModelObject;
/**
* Retrieve the searched-for items by building instances from this class. This objects contains
* for every type specified by the {@code type} parameter in the {@link SearchItemRequest}
* the searched-for items wrapped in a {@link Paging} object.
*/
@JsonDeserialize(builder = SearchResult.Builder.class)
public class SearchResult extends AbstractModelObject implements IArtistTrackModelObject, ISearchModelObject {
private final Paging albums;
private final Paging artists;
private final Paging episodes;
private final Paging playlists;
private final Paging shows;
private final Paging
© 2015 - 2024 Weber Informatics LLC | Privacy Policy