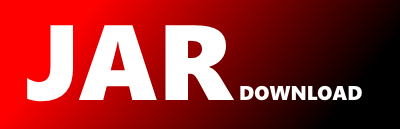
se.natusoft.doc.markdown.util.SourcePaths Maven / Gradle / Ivy
/*
*
* PROJECT
* Name
* MarkdownDoc Maven Plugin
*
* Code Version
* 1.2.4
*
* Description
* A maven plugin for generating documentation from markdown.
*
* COPYRIGHTS
* Copyright (C) 2012 by Natusoft AB All rights reserved.
*
* LICENSE
* Apache 2.0 (Open Source)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* AUTHORS
* Tommy Svensson ([email protected])
* Changes:
* 2012-11-21: Created!
*
*/
package se.natusoft.doc.markdown.util;
import se.natusoft.doc.markdown.util.SourcePath;
import java.io.File;
import java.util.LinkedList;
import java.util.List;
import java.util.StringTokenizer;
/**
* This handles a comma separated set of SourcePaths.
*/
public class SourcePaths {
//
// Private Members
//
/** All the source paths. */
List sourcePaths = new LinkedList();
//
// Constructors
//
/**
* Creates a new SourcePaths.
*
* @param sourcePaths The comma separated path specifications to parse.
*/
public SourcePaths(String sourcePaths) {
StringTokenizer pathTokenizer = new StringTokenizer(sourcePaths, ",");
while (pathTokenizer.hasMoreTokens()) {
this.sourcePaths.add(new SourcePath(pathTokenizer.nextToken().trim()));
}
}
/**
* Creates a new SourcePaths.
*
* @param projRoot The root dir that all source paths are relative to.
* @param sourcePaths The comma separated path specifications to parse.
*/
public SourcePaths(File projRoot, String sourcePaths) {
StringTokenizer pathTokenizer = new StringTokenizer(sourcePaths, ",");
while (pathTokenizer.hasMoreTokens()) {
String path = pathTokenizer.nextToken().trim();
if (path.startsWith(projRoot.getAbsolutePath())) {
int projRootLength = projRoot.getAbsolutePath().length();
path = path.substring(projRootLength);
}
this.sourcePaths.add(new SourcePath(projRoot, path));
}
}
//
// Methods
//
/**
* Returns all specified source paths.
*/
public List getSourcePaths() {
return this.sourcePaths;
}
/**
* Returns all files from all source paths (in the order they were specified).
*/
public List getSourceFiles() {
List all = new LinkedList();
for (SourcePath sourcePath : this.sourcePaths) {
for (File file : sourcePath.getSourceFiles()) {
all.add(file);
}
}
return all;
}
/**
* @return true if there are source files.
*/
public boolean hasSourceFiles() {
return !getSourceFiles().isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy