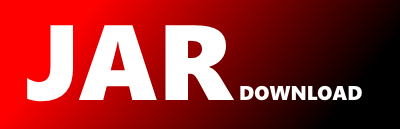
riv.ehr.patientsummary._1.ADXP Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlValue;
/**
* Java class for ADXP complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ADXP">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="code" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="codeSystem" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="codeSystemVersion" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="language" type="{urn:riv:ehr:patientsummary:1}Code" />
* <attribute name="type" type="{urn:riv:ehr:patientsummary:1}AddressPartType" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ADXP", propOrder = {
"content"
})
@XmlSeeAlso({
ADXPSTTYP.class,
ADXPDAL.class,
ADXPUNIT.class,
ADXPUNID.class,
ADXPADL.class,
ADXPCEN.class,
ADXPDINST.class,
ADXPSAL.class,
ADXPCTY.class,
ADXPPOB.class,
ADXPBNN.class,
ADXPBNR.class,
ADXPBNS.class,
ADXPCNT.class,
ADXPDINSTA.class,
ADXPINT.class,
ADXPDINSTQ.class,
ADXPCPA.class,
ADXPPRE.class,
ADXPDMODID.class,
ADXPAL.class,
ADXPDMOD.class,
ADXPSTR.class,
ADXPBR.class,
ADXPSTB.class,
ADXPSTA.class,
ADXPCAR.class,
ADXPZIP.class,
ADXPDIR.class
})
public class ADXP {
@XmlValue
protected String content;
@XmlAttribute(name = "code")
protected String code;
@XmlAttribute(name = "codeSystem")
protected String codeSystem;
@XmlAttribute(name = "codeSystemVersion")
protected String codeSystemVersion;
@XmlAttribute(name = "language")
protected String language;
@XmlAttribute(name = "type")
protected AddressPartType type;
/**
* Gets the value of the content property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContent() {
return content;
}
/**
* Sets the value of the content property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContent(String value) {
this.content = value;
}
/**
* Gets the value of the code property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCode(String value) {
this.code = value;
}
/**
* Gets the value of the codeSystem property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeSystem() {
return codeSystem;
}
/**
* Sets the value of the codeSystem property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeSystem(String value) {
this.codeSystem = value;
}
/**
* Gets the value of the codeSystemVersion property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeSystemVersion() {
return codeSystemVersion;
}
/**
* Sets the value of the codeSystemVersion property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeSystemVersion(String value) {
this.codeSystemVersion = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link AddressPartType }
*
*/
public AddressPartType getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link AddressPartType }
*
*/
public void setType(AddressPartType value) {
this.type = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy