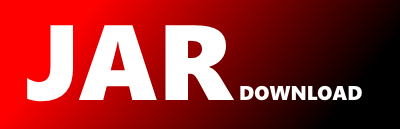
riv.ehr.patientsummary._1.ATTESTATIONINFO Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* Any attestations for a Record Component. The proof field
* should be a cryptographic hash of the RECORD_COMPONENT it
* attests, but it is recognised that in many implementations
* the components will change to point to new components that
* revise them. In that case, the proof should sign the
* unchanging parts of the component only.
*
*
* Java class for ATTESTATION_INFO complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ATTESTATION_INFO">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="attested_view" type="{urn:riv:ehr:patientsummary:1}ED" minOccurs="0"/>
* <element name="proof" type="{urn:riv:ehr:patientsummary:1}ED" minOccurs="0"/>
* <element name="reason_for_attestation" type="{urn:riv:ehr:patientsummary:1}CD"/>
* <element name="time" type="{urn:riv:ehr:patientsummary:1}TS"/>
* <element name="target_rc_id" type="{urn:riv:ehr:patientsummary:1}II" maxOccurs="unbounded"/>
* <element name="attester" type="{urn:riv:ehr:patientsummary:1}FUNCTIONAL_ROLE"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ATTESTATION_INFO", propOrder = {
"attestedView",
"proof",
"reasonForAttestation",
"time",
"targetRcId",
"attester"
})
public class ATTESTATIONINFO {
@XmlElement(name = "attested_view")
protected ED attestedView;
protected ED proof;
@XmlElement(name = "reason_for_attestation", required = true)
protected CD reasonForAttestation;
@XmlElement(required = true)
protected TS time;
@XmlElement(name = "target_rc_id", required = true)
protected List targetRcId;
@XmlElement(required = true)
protected FUNCTIONALROLE attester;
/**
* Gets the value of the attestedView property.
*
* @return
* possible object is
* {@link ED }
*
*/
public ED getAttestedView() {
return attestedView;
}
/**
* Sets the value of the attestedView property.
*
* @param value
* allowed object is
* {@link ED }
*
*/
public void setAttestedView(ED value) {
this.attestedView = value;
}
/**
* Gets the value of the proof property.
*
* @return
* possible object is
* {@link ED }
*
*/
public ED getProof() {
return proof;
}
/**
* Sets the value of the proof property.
*
* @param value
* allowed object is
* {@link ED }
*
*/
public void setProof(ED value) {
this.proof = value;
}
/**
* Gets the value of the reasonForAttestation property.
*
* @return
* possible object is
* {@link CD }
*
*/
public CD getReasonForAttestation() {
return reasonForAttestation;
}
/**
* Sets the value of the reasonForAttestation property.
*
* @param value
* allowed object is
* {@link CD }
*
*/
public void setReasonForAttestation(CD value) {
this.reasonForAttestation = value;
}
/**
* Gets the value of the time property.
*
* @return
* possible object is
* {@link TS }
*
*/
public TS getTime() {
return time;
}
/**
* Sets the value of the time property.
*
* @param value
* allowed object is
* {@link TS }
*
*/
public void setTime(TS value) {
this.time = value;
}
/**
* Gets the value of the targetRcId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the targetRcId property.
*
*
* For example, to add a new item, do as follows:
*
* getTargetRcId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link II }
*
*
*/
public List getTargetRcId() {
if (targetRcId == null) {
targetRcId = new ArrayList();
}
return this.targetRcId;
}
/**
* Gets the value of the attester property.
*
* @return
* possible object is
* {@link FUNCTIONALROLE }
*
*/
public FUNCTIONALROLE getAttester() {
return attester;
}
/**
* Sets the value of the attester property.
*
* @param value
* allowed object is
* {@link FUNCTIONALROLE }
*
*/
public void setAttester(FUNCTIONALROLE value) {
this.attester = value;
}
}