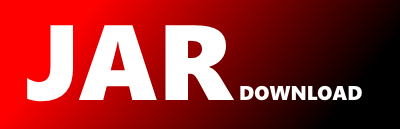
riv.ehr.patientsummary._1.AUDITINFO Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* The committal and revision data for a Record Component.
* Note that the invariant is incorrectly stated as
* constraining "attribute_version_status.coding_scheme_name"
* in the printed standard.
*
*
* Java class for AUDIT_INFO complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AUDIT_INFO">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="committer" type="{urn:riv:ehr:patientsummary:1}II"/>
* <element name="ehr_system" type="{urn:riv:ehr:patientsummary:1}II"/>
* <element name="previous_version" type="{urn:riv:ehr:patientsummary:1}II" minOccurs="0"/>
* <element name="reason_for_revision" type="{urn:riv:ehr:patientsummary:1}CD" minOccurs="0"/>
* <element name="time_committed" type="{urn:riv:ehr:patientsummary:1}TS"/>
* <element name="version_set_id" type="{urn:riv:ehr:patientsummary:1}II" minOccurs="0"/>
* <element name="version_status" type="{urn:riv:ehr:patientsummary:1}CS" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AUDIT_INFO", propOrder = {
"committer",
"ehrSystem",
"previousVersion",
"reasonForRevision",
"timeCommitted",
"versionSetId",
"versionStatus"
})
public class AUDITINFO {
@XmlElement(required = true)
protected II committer;
@XmlElement(name = "ehr_system", required = true)
protected II ehrSystem;
@XmlElement(name = "previous_version")
protected II previousVersion;
@XmlElement(name = "reason_for_revision")
protected CD reasonForRevision;
@XmlElement(name = "time_committed", required = true)
protected TS timeCommitted;
@XmlElement(name = "version_set_id")
protected II versionSetId;
@XmlElement(name = "version_status")
protected CS versionStatus;
/**
* Gets the value of the committer property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getCommitter() {
return committer;
}
/**
* Sets the value of the committer property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setCommitter(II value) {
this.committer = value;
}
/**
* Gets the value of the ehrSystem property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getEhrSystem() {
return ehrSystem;
}
/**
* Sets the value of the ehrSystem property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setEhrSystem(II value) {
this.ehrSystem = value;
}
/**
* Gets the value of the previousVersion property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getPreviousVersion() {
return previousVersion;
}
/**
* Sets the value of the previousVersion property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setPreviousVersion(II value) {
this.previousVersion = value;
}
/**
* Gets the value of the reasonForRevision property.
*
* @return
* possible object is
* {@link CD }
*
*/
public CD getReasonForRevision() {
return reasonForRevision;
}
/**
* Sets the value of the reasonForRevision property.
*
* @param value
* allowed object is
* {@link CD }
*
*/
public void setReasonForRevision(CD value) {
this.reasonForRevision = value;
}
/**
* Gets the value of the timeCommitted property.
*
* @return
* possible object is
* {@link TS }
*
*/
public TS getTimeCommitted() {
return timeCommitted;
}
/**
* Sets the value of the timeCommitted property.
*
* @param value
* allowed object is
* {@link TS }
*
*/
public void setTimeCommitted(TS value) {
this.timeCommitted = value;
}
/**
* Gets the value of the versionSetId property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getVersionSetId() {
return versionSetId;
}
/**
* Sets the value of the versionSetId property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setVersionSetId(II value) {
this.versionSetId = value;
}
/**
* Gets the value of the versionStatus property.
*
* @return
* possible object is
* {@link CS }
*
*/
public CS getVersionStatus() {
return versionStatus;
}
/**
* Sets the value of the versionStatus property.
*
* @param value
* allowed object is
* {@link CS }
*
*/
public void setVersionStatus(CS value) {
this.versionStatus = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy