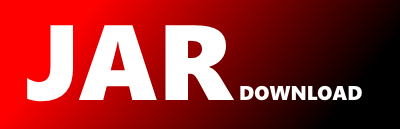
riv.ehr.patientsummary._1.COMPOSITION Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* Key aggregation class representing the set of Record
* Components authored and committed during a single clinical
* encounter. Note the values for territory will indicate
* the legal framework under which the data was committed.
* The session_time should be a restriction on the data types
* of low and high to make them TS, but this restriction is
* not permissible in XML Schema and thus the IVL properties
* are simply copied here with the appropriate types.
*
*
* Java class for COMPOSITION complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="COMPOSITION">
* <complexContent>
* <extension base="{urn:riv:ehr:patientsummary:1}RECORD_COMPONENT">
* <sequence>
* <element name="contribution_id" type="{urn:riv:ehr:patientsummary:1}II" minOccurs="0"/>
* <element name="session_time" type="{urn:riv:ehr:patientsummary:1}IVL_TS" minOccurs="0"/>
* <element name="territory" type="{urn:riv:ehr:patientsummary:1}CS" minOccurs="0"/>
* <element name="attestations" type="{urn:riv:ehr:patientsummary:1}ATTESTATION_INFO" maxOccurs="unbounded" minOccurs="0"/>
* <element name="other_participations" type="{urn:riv:ehr:patientsummary:1}FUNCTIONAL_ROLE" maxOccurs="unbounded" minOccurs="0"/>
* <element name="committal" type="{urn:riv:ehr:patientsummary:1}AUDIT_INFO"/>
* <element name="composer" type="{urn:riv:ehr:patientsummary:1}FUNCTIONAL_ROLE" minOccurs="0"/>
* <element name="content" type="{urn:riv:ehr:patientsummary:1}CONTENT" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "COMPOSITION", propOrder = {
"contributionId",
"sessionTime",
"territory",
"attestations",
"otherParticipations",
"committal",
"composer",
"content"
})
public class COMPOSITION
extends RECORDCOMPONENT
{
@XmlElement(name = "contribution_id")
protected II contributionId;
@XmlElement(name = "session_time")
protected IVLTS sessionTime;
protected CS territory;
protected List attestations;
@XmlElement(name = "other_participations")
protected List otherParticipations;
@XmlElement(required = true)
protected AUDITINFO committal;
protected FUNCTIONALROLE composer;
protected List content;
/**
* Gets the value of the contributionId property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getContributionId() {
return contributionId;
}
/**
* Sets the value of the contributionId property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setContributionId(II value) {
this.contributionId = value;
}
/**
* Gets the value of the sessionTime property.
*
* @return
* possible object is
* {@link IVLTS }
*
*/
public IVLTS getSessionTime() {
return sessionTime;
}
/**
* Sets the value of the sessionTime property.
*
* @param value
* allowed object is
* {@link IVLTS }
*
*/
public void setSessionTime(IVLTS value) {
this.sessionTime = value;
}
/**
* Gets the value of the territory property.
*
* @return
* possible object is
* {@link CS }
*
*/
public CS getTerritory() {
return territory;
}
/**
* Sets the value of the territory property.
*
* @param value
* allowed object is
* {@link CS }
*
*/
public void setTerritory(CS value) {
this.territory = value;
}
/**
* Gets the value of the attestations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the attestations property.
*
*
* For example, to add a new item, do as follows:
*
* getAttestations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ATTESTATIONINFO }
*
*
*/
public List getAttestations() {
if (attestations == null) {
attestations = new ArrayList();
}
return this.attestations;
}
/**
* Gets the value of the otherParticipations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the otherParticipations property.
*
*
* For example, to add a new item, do as follows:
*
* getOtherParticipations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FUNCTIONALROLE }
*
*
*/
public List getOtherParticipations() {
if (otherParticipations == null) {
otherParticipations = new ArrayList();
}
return this.otherParticipations;
}
/**
* Gets the value of the committal property.
*
* @return
* possible object is
* {@link AUDITINFO }
*
*/
public AUDITINFO getCommittal() {
return committal;
}
/**
* Sets the value of the committal property.
*
* @param value
* allowed object is
* {@link AUDITINFO }
*
*/
public void setCommittal(AUDITINFO value) {
this.committal = value;
}
/**
* Gets the value of the composer property.
*
* @return
* possible object is
* {@link FUNCTIONALROLE }
*
*/
public FUNCTIONALROLE getComposer() {
return composer;
}
/**
* Sets the value of the composer property.
*
* @param value
* allowed object is
* {@link FUNCTIONALROLE }
*
*/
public void setComposer(FUNCTIONALROLE value) {
this.composer = value;
}
/**
* Gets the value of the content property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the content property.
*
*
* For example, to add a new item, do as follows:
*
* getContent().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CONTENT }
*
*
*/
public List getContent() {
if (content == null) {
content = new ArrayList();
}
return this.content;
}
}