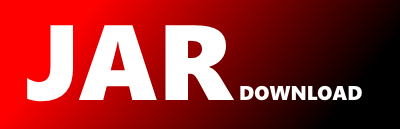
riv.ehr.patientsummary._1.ED Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for ED complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ED">
* <complexContent>
* <extension base="{urn:riv:ehr:patientsummary:1}ANY">
* <sequence>
* <element name="data" type="{http://www.w3.org/2001/XMLSchema}base64Binary" minOccurs="0"/>
* <element name="xml" type="{http://www.w3.org/2001/XMLSchema}anyType" minOccurs="0"/>
* <element name="reference" type="{urn:riv:ehr:patientsummary:1}TEL.URL" minOccurs="0"/>
* <element name="integrityCheck" type="{http://www.w3.org/2001/XMLSchema}base64Binary" minOccurs="0"/>
* <element name="thumbnail" type="{urn:riv:ehr:patientsummary:1}ED" minOccurs="0"/>
* <element name="description" type="{urn:riv:ehr:patientsummary:1}ST" minOccurs="0"/>
* <element name="translation" type="{urn:riv:ehr:patientsummary:1}ED" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="value" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="mediaType" type="{http://www.w3.org/2001/XMLSchema}string" default="text/plain" />
* <attribute name="charset" type="{urn:riv:ehr:patientsummary:1}Code" />
* <attribute name="language" type="{urn:riv:ehr:patientsummary:1}Code" />
* <attribute name="compression" type="{urn:riv:ehr:patientsummary:1}Compression" />
* <attribute name="integrityCheckAlgorithm" type="{urn:riv:ehr:patientsummary:1}IntegrityCheckAlgorithm" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ED", propOrder = {
"data",
"xml",
"reference",
"integrityCheck",
"thumbnail",
"description",
"translation"
})
@XmlSeeAlso({
EDSTRUCTUREDTITLE.class,
EDIMAGE.class,
EDSTRUCTUREDTEXT.class,
EDSIGNATURE.class,
EDTEXT.class,
EDDOC.class
})
public class ED
extends ANY
{
protected byte[] data;
protected Object xml;
protected TELURL reference;
protected byte[] integrityCheck;
protected ED thumbnail;
protected ST description;
protected List translation;
@XmlAttribute(name = "value")
protected String value;
@XmlAttribute(name = "mediaType")
protected String mediaType;
@XmlAttribute(name = "charset")
protected String charset;
@XmlAttribute(name = "language")
protected String language;
@XmlAttribute(name = "compression")
protected Compression compression;
@XmlAttribute(name = "integrityCheckAlgorithm")
protected IntegrityCheckAlgorithm integrityCheckAlgorithm;
/**
* Gets the value of the data property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getData() {
return data;
}
/**
* Sets the value of the data property.
*
* @param value
* allowed object is
* byte[]
*/
public void setData(byte[] value) {
this.data = value;
}
/**
* Gets the value of the xml property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getXml() {
return xml;
}
/**
* Sets the value of the xml property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setXml(Object value) {
this.xml = value;
}
/**
* Gets the value of the reference property.
*
* @return
* possible object is
* {@link TELURL }
*
*/
public TELURL getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is
* {@link TELURL }
*
*/
public void setReference(TELURL value) {
this.reference = value;
}
/**
* Gets the value of the integrityCheck property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getIntegrityCheck() {
return integrityCheck;
}
/**
* Sets the value of the integrityCheck property.
*
* @param value
* allowed object is
* byte[]
*/
public void setIntegrityCheck(byte[] value) {
this.integrityCheck = value;
}
/**
* Gets the value of the thumbnail property.
*
* @return
* possible object is
* {@link ED }
*
*/
public ED getThumbnail() {
return thumbnail;
}
/**
* Sets the value of the thumbnail property.
*
* @param value
* allowed object is
* {@link ED }
*
*/
public void setThumbnail(ED value) {
this.thumbnail = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link ST }
*
*/
public ST getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link ST }
*
*/
public void setDescription(ST value) {
this.description = value;
}
/**
* Gets the value of the translation property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the translation property.
*
*
* For example, to add a new item, do as follows:
*
* getTranslation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ED }
*
*
*/
public List getTranslation() {
if (translation == null) {
translation = new ArrayList();
}
return this.translation;
}
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
/**
* Gets the value of the mediaType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMediaType() {
if (mediaType == null) {
return "text/plain";
} else {
return mediaType;
}
}
/**
* Sets the value of the mediaType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMediaType(String value) {
this.mediaType = value;
}
/**
* Gets the value of the charset property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCharset() {
return charset;
}
/**
* Sets the value of the charset property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCharset(String value) {
this.charset = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the compression property.
*
* @return
* possible object is
* {@link Compression }
*
*/
public Compression getCompression() {
return compression;
}
/**
* Sets the value of the compression property.
*
* @param value
* allowed object is
* {@link Compression }
*
*/
public void setCompression(Compression value) {
this.compression = value;
}
/**
* Gets the value of the integrityCheckAlgorithm property.
*
* @return
* possible object is
* {@link IntegrityCheckAlgorithm }
*
*/
public IntegrityCheckAlgorithm getIntegrityCheckAlgorithm() {
return integrityCheckAlgorithm;
}
/**
* Sets the value of the integrityCheckAlgorithm property.
*
* @param value
* allowed object is
* {@link IntegrityCheckAlgorithm }
*
*/
public void setIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
this.integrityCheckAlgorithm = value;
}
}