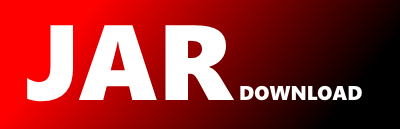
riv.ehr.patientsummary._1.ENTRY Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* Represents the information acquired for a single clinical
* activity or recording. The Entry may be nilled if it
* corrects a previous erroneous version.
*
*
* Java class for ENTRY complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ENTRY">
* <complexContent>
* <extension base="{urn:riv:ehr:patientsummary:1}CONTENT">
* <sequence>
* <element name="act_id" type="{urn:riv:ehr:patientsummary:1}ST" minOccurs="0"/>
* <element name="act_status" type="{urn:riv:ehr:patientsummary:1}CS" minOccurs="0"/>
* <element name="subject_of_information_category" type="{urn:riv:ehr:patientsummary:1}CS" minOccurs="0"/>
* <element name="uncertainty_expressed" type="{urn:riv:ehr:patientsummary:1}BL"/>
* <element name="items" type="{urn:riv:ehr:patientsummary:1}ITEM" maxOccurs="unbounded" minOccurs="0"/>
* <element name="info_provider" type="{urn:riv:ehr:patientsummary:1}FUNCTIONAL_ROLE" minOccurs="0"/>
* <element name="other_participations" type="{urn:riv:ehr:patientsummary:1}FUNCTIONAL_ROLE" maxOccurs="unbounded" minOccurs="0"/>
* <element name="subject_of_information" type="{urn:riv:ehr:patientsummary:1}RELATED_PARTY" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ENTRY", propOrder = {
"actId",
"actStatus",
"subjectOfInformationCategory",
"uncertaintyExpressed",
"items",
"infoProvider",
"otherParticipations",
"subjectOfInformation"
})
public class ENTRY
extends CONTENT
{
@XmlElement(name = "act_id")
protected ST actId;
@XmlElement(name = "act_status")
protected CS actStatus;
@XmlElement(name = "subject_of_information_category")
protected CS subjectOfInformationCategory;
@XmlElement(name = "uncertainty_expressed", required = true)
protected BL uncertaintyExpressed;
@XmlElement(nillable = true)
protected List- items;
@XmlElement(name = "info_provider")
protected FUNCTIONALROLE infoProvider;
@XmlElement(name = "other_participations")
protected List
otherParticipations;
@XmlElement(name = "subject_of_information")
protected RELATEDPARTY subjectOfInformation;
/**
* Gets the value of the actId property.
*
* @return
* possible object is
* {@link ST }
*
*/
public ST getActId() {
return actId;
}
/**
* Sets the value of the actId property.
*
* @param value
* allowed object is
* {@link ST }
*
*/
public void setActId(ST value) {
this.actId = value;
}
/**
* Gets the value of the actStatus property.
*
* @return
* possible object is
* {@link CS }
*
*/
public CS getActStatus() {
return actStatus;
}
/**
* Sets the value of the actStatus property.
*
* @param value
* allowed object is
* {@link CS }
*
*/
public void setActStatus(CS value) {
this.actStatus = value;
}
/**
* Gets the value of the subjectOfInformationCategory property.
*
* @return
* possible object is
* {@link CS }
*
*/
public CS getSubjectOfInformationCategory() {
return subjectOfInformationCategory;
}
/**
* Sets the value of the subjectOfInformationCategory property.
*
* @param value
* allowed object is
* {@link CS }
*
*/
public void setSubjectOfInformationCategory(CS value) {
this.subjectOfInformationCategory = value;
}
/**
* Gets the value of the uncertaintyExpressed property.
*
* @return
* possible object is
* {@link BL }
*
*/
public BL getUncertaintyExpressed() {
return uncertaintyExpressed;
}
/**
* Sets the value of the uncertaintyExpressed property.
*
* @param value
* allowed object is
* {@link BL }
*
*/
public void setUncertaintyExpressed(BL value) {
this.uncertaintyExpressed = value;
}
/**
* Gets the value of the items property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the items property.
*
*
* For example, to add a new item, do as follows:
*
* getItems().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ITEM }
*
*
*/
public List- getItems() {
if (items == null) {
items = new ArrayList
- ();
}
return this.items;
}
/**
* Gets the value of the infoProvider property.
*
* @return
* possible object is
* {@link FUNCTIONALROLE }
*
*/
public FUNCTIONALROLE getInfoProvider() {
return infoProvider;
}
/**
* Sets the value of the infoProvider property.
*
* @param value
* allowed object is
* {@link FUNCTIONALROLE }
*
*/
public void setInfoProvider(FUNCTIONALROLE value) {
this.infoProvider = value;
}
/**
* Gets the value of the otherParticipations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the otherParticipations property.
*
*
* For example, to add a new item, do as follows:
*
* getOtherParticipations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FUNCTIONALROLE }
*
*
*/
public List getOtherParticipations() {
if (otherParticipations == null) {
otherParticipations = new ArrayList();
}
return this.otherParticipations;
}
/**
* Gets the value of the subjectOfInformation property.
*
* @return
* possible object is
* {@link RELATEDPARTY }
*
*/
public RELATEDPARTY getSubjectOfInformation() {
return subjectOfInformation;
}
/**
* Sets the value of the subjectOfInformation property.
*
* @param value
* allowed object is
* {@link RELATEDPARTY }
*
*/
public void setSubjectOfInformation(RELATEDPARTY value) {
this.subjectOfInformation = value;
}
}