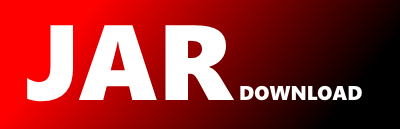
riv.ehr.patientsummary._1.ObjectFactory Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the riv.ehr.patientsummary._1 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _StrucDocTitleSup_QNAME = new QName("urn:riv:ehr:patientsummary:1", "sup");
private final static QName _StrucDocTitleLinkHtml_QNAME = new QName("urn:riv:ehr:patientsummary:1", "linkHtml");
private final static QName _StrucDocTitleBr_QNAME = new QName("urn:riv:ehr:patientsummary:1", "br");
private final static QName _StrucDocTitleContent_QNAME = new QName("urn:riv:ehr:patientsummary:1", "content");
private final static QName _StrucDocTitleSub_QNAME = new QName("urn:riv:ehr:patientsummary:1", "sub");
private final static QName _StrucDocTitleFootnote_QNAME = new QName("urn:riv:ehr:patientsummary:1", "footnote");
private final static QName _StrucDocTitleFootnoteRef_QNAME = new QName("urn:riv:ehr:patientsummary:1", "footnoteRef");
private final static QName _StrucDocTCellList_QNAME = new QName("urn:riv:ehr:patientsummary:1", "list");
private final static QName _StrucDocTCellTable_QNAME = new QName("urn:riv:ehr:patientsummary:1", "table");
private final static QName _StrucDocTCellRenderMultiMedia_QNAME = new QName("urn:riv:ehr:patientsummary:1", "renderMultiMedia");
private final static QName _StrucDocTCellParagraph_QNAME = new QName("urn:riv:ehr:patientsummary:1", "paragraph");
private final static QName _StrucDocTRowTd_QNAME = new QName("urn:riv:ehr:patientsummary:1", "td");
private final static QName _StrucDocTRowTh_QNAME = new QName("urn:riv:ehr:patientsummary:1", "th");
private final static QName _StrucDocCaptionedCaption_QNAME = new QName("urn:riv:ehr:patientsummary:1", "caption");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: riv.ehr.patientsummary._1
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link UVPTSBIRTH }
*
*/
public UVPTSBIRTH createUVPTSBIRTH() {
return new UVPTSBIRTH();
}
/**
* Create an instance of {@link NPPDTELPHONE }
*
*/
public NPPDTELPHONE createNPPDTELPHONE() {
return new NPPDTELPHONE();
}
/**
* Create an instance of {@link URGHIGHRTO }
*
*/
public URGHIGHRTO createURGHIGHRTO() {
return new URGHIGHRTO();
}
/**
* Create an instance of {@link DSETBL }
*
*/
public DSETBL createDSETBL() {
return new DSETBL();
}
/**
* Create an instance of {@link ED }
*
*/
public ED createED() {
return new ED();
}
/**
* Create an instance of {@link URGLOWMO }
*
*/
public URGLOWMO createURGLOWMO() {
return new URGLOWMO();
}
/**
* Create an instance of {@link HISTTSDATETIME }
*
*/
public HISTTSDATETIME createHISTTSDATETIME() {
return new HISTTSDATETIME();
}
/**
* Create an instance of {@link NPPDTSDATETIME }
*
*/
public NPPDTSDATETIME createNPPDTSDATETIME() {
return new NPPDTSDATETIME();
}
/**
* Create an instance of {@link BAGENTN }
*
*/
public BAGENTN createBAGENTN() {
return new BAGENTN();
}
/**
* Create an instance of {@link LISTEDSIGNATURE }
*
*/
public LISTEDSIGNATURE createLISTEDSIGNATURE() {
return new LISTEDSIGNATURE();
}
/**
* Create an instance of {@link QSPTSDATEFULL }
*
*/
public QSPTSDATEFULL createQSPTSDATEFULL() {
return new QSPTSDATEFULL();
}
/**
* Create an instance of {@link URGLOWREAL }
*
*/
public URGLOWREAL createURGLOWREAL() {
return new URGLOWREAL();
}
/**
* Create an instance of {@link QSPMO }
*
*/
public QSPMO createQSPMO() {
return new QSPMO();
}
/**
* Create an instance of {@link QSPINT }
*
*/
public QSPINT createQSPINT() {
return new QSPINT();
}
/**
* Create an instance of {@link StrucDocBr }
*
*/
public StrucDocBr createStrucDocBr() {
return new StrucDocBr();
}
/**
* Create an instance of {@link URGPQ }
*
*/
public URGPQ createURGPQ() {
return new URGPQ();
}
/**
* Create an instance of {@link UVPEDIMAGE }
*
*/
public UVPEDIMAGE createUVPEDIMAGE() {
return new UVPEDIMAGE();
}
/**
* Create an instance of {@link QSDPQ }
*
*/
public QSDPQ createQSDPQ() {
return new QSDPQ();
}
/**
* Create an instance of {@link HISTEDSTRUCTUREDTITLE }
*
*/
public HISTEDSTRUCTUREDTITLE createHISTEDSTRUCTUREDTITLE() {
return new HISTEDSTRUCTUREDTITLE();
}
/**
* Create an instance of {@link QSUTSDATETIME }
*
*/
public QSUTSDATETIME createQSUTSDATETIME() {
return new QSUTSDATETIME();
}
/**
* Create an instance of {@link PQTIME }
*
*/
public PQTIME createPQTIME() {
return new PQTIME();
}
/**
* Create an instance of {@link NPPDPQTIME }
*
*/
public NPPDPQTIME createNPPDPQTIME() {
return new NPPDPQTIME();
}
/**
* Create an instance of {@link QSUTSDATEFULL }
*
*/
public QSUTSDATEFULL createQSUTSDATEFULL() {
return new QSUTSDATEFULL();
}
/**
* Create an instance of {@link URGHIGHINT }
*
*/
public URGHIGHINT createURGHIGHINT() {
return new URGHIGHINT();
}
/**
* Create an instance of {@link StrucDocTRowGroup }
*
*/
public StrucDocTRowGroup createStrucDocTRowGroup() {
return new StrucDocTRowGroup();
}
/**
* Create an instance of {@link DSETAD }
*
*/
public DSETAD createDSETAD() {
return new DSETAD();
}
/**
* Create an instance of {@link NPPDMO }
*
*/
public NPPDMO createNPPDMO() {
return new NPPDMO();
}
/**
* Create an instance of {@link DSETTELPHONE }
*
*/
public DSETTELPHONE createDSETTELPHONE() {
return new DSETTELPHONE();
}
/**
* Create an instance of {@link ELEMENT }
*
*/
public ELEMENT createELEMENT() {
return new ELEMENT();
}
/**
* Create an instance of {@link EN }
*
*/
public EN createEN() {
return new EN();
}
/**
* Create an instance of {@link IVLLOWPQ }
*
*/
public IVLLOWPQ createIVLLOWPQ() {
return new IVLLOWPQ();
}
/**
* Create an instance of {@link QSUINT }
*
*/
public QSUINT createQSUINT() {
return new QSUINT();
}
/**
* Create an instance of {@link StrucDocTitleFootnote }
*
*/
public StrucDocTitleFootnote createStrucDocTitleFootnote() {
return new StrucDocTitleFootnote();
}
/**
* Create an instance of {@link BAGPQTIME }
*
*/
public BAGPQTIME createBAGPQTIME() {
return new BAGPQTIME();
}
/**
* Create an instance of {@link URGLOWTS }
*
*/
public URGLOWTS createURGLOWTS() {
return new URGLOWTS();
}
/**
* Create an instance of {@link TSDATETIME }
*
*/
public TSDATETIME createTSDATETIME() {
return new TSDATETIME();
}
/**
* Create an instance of {@link NPPDCDCV }
*
*/
public NPPDCDCV createNPPDCDCV() {
return new NPPDCDCV();
}
/**
* Create an instance of {@link HISTREAL }
*
*/
public HISTREAL createHISTREAL() {
return new HISTREAL();
}
/**
* Create an instance of {@link UVPTELPHONE }
*
*/
public UVPTELPHONE createUVPTELPHONE() {
return new UVPTELPHONE();
}
/**
* Create an instance of {@link UVPSC }
*
*/
public UVPSC createUVPSC() {
return new UVPSC();
}
/**
* Create an instance of {@link TSDATETIMEFULL }
*
*/
public TSDATETIMEFULL createTSDATETIMEFULL() {
return new TSDATETIMEFULL();
}
/**
* Create an instance of {@link BAGINTPOS }
*
*/
public BAGINTPOS createBAGINTPOS() {
return new BAGINTPOS();
}
/**
* Create an instance of {@link NPPDINT }
*
*/
public NPPDINT createNPPDINT() {
return new NPPDINT();
}
/**
* Create an instance of {@link ADXPSTTYP }
*
*/
public ADXPSTTYP createADXPSTTYP() {
return new ADXPSTTYP();
}
/**
* Create an instance of {@link NPPDREAL }
*
*/
public NPPDREAL createNPPDREAL() {
return new NPPDREAL();
}
/**
* Create an instance of {@link SLISTREAL }
*
*/
public SLISTREAL createSLISTREAL() {
return new SLISTREAL();
}
/**
* Create an instance of {@link ADXPDAL }
*
*/
public ADXPDAL createADXPDAL() {
return new ADXPDAL();
}
/**
* Create an instance of {@link QSPPQTIME }
*
*/
public QSPPQTIME createQSPPQTIME() {
return new QSPPQTIME();
}
/**
* Create an instance of {@link UVPST }
*
*/
public UVPST createUVPST() {
return new UVPST();
}
/**
* Create an instance of {@link BAGEDDOCREF }
*
*/
public BAGEDDOCREF createBAGEDDOCREF() {
return new BAGEDDOCREF();
}
/**
* Create an instance of {@link QSUINTNONNEG }
*
*/
public QSUINTNONNEG createQSUINTNONNEG() {
return new QSUINTNONNEG();
}
/**
* Create an instance of {@link IVLLOWMO }
*
*/
public IVLLOWMO createIVLLOWMO() {
return new IVLLOWMO();
}
/**
* Create an instance of {@link EIVLTS }
*
*/
public EIVLTS createEIVLTS() {
return new EIVLTS();
}
/**
* Create an instance of {@link INT }
*
*/
public INT createINT() {
return new INT();
}
/**
* Create an instance of {@link IVLWIDTHTSDATETIMEFULL }
*
*/
public IVLWIDTHTSDATETIMEFULL createIVLWIDTHTSDATETIMEFULL() {
return new IVLWIDTHTSDATETIMEFULL();
}
/**
* Create an instance of {@link IVLCO }
*
*/
public IVLCO createIVLCO() {
return new IVLCO();
}
/**
* Create an instance of {@link IVLWIDTHTS }
*
*/
public IVLWIDTHTS createIVLWIDTHTS() {
return new IVLWIDTHTS();
}
/**
* Create an instance of {@link BAGAD }
*
*/
public BAGAD createBAGAD() {
return new BAGAD();
}
/**
* Create an instance of {@link SLISTMO }
*
*/
public SLISTMO createSLISTMO() {
return new SLISTMO();
}
/**
* Create an instance of {@link BAGEDTEXT }
*
*/
public BAGEDTEXT createBAGEDTEXT() {
return new BAGEDTEXT();
}
/**
* Create an instance of {@link IVLWIDTHRTO }
*
*/
public IVLWIDTHRTO createIVLWIDTHRTO() {
return new IVLWIDTHRTO();
}
/**
* Create an instance of {@link BAGEDSTRUCTUREDTITLE }
*
*/
public BAGEDSTRUCTUREDTITLE createBAGEDSTRUCTUREDTITLE() {
return new BAGEDSTRUCTUREDTITLE();
}
/**
* Create an instance of {@link ADXPUNIT }
*
*/
public ADXPUNIT createADXPUNIT() {
return new ADXPUNIT();
}
/**
* Create an instance of {@link DSETCO }
*
*/
public DSETCO createDSETCO() {
return new DSETCO();
}
/**
* Create an instance of {@link QSITSDATEFULL }
*
*/
public QSITSDATEFULL createQSITSDATEFULL() {
return new QSITSDATEFULL();
}
/**
* Create an instance of {@link DSETCD }
*
*/
public DSETCD createDSETCD() {
return new DSETCD();
}
/**
* Create an instance of {@link HISTTSBIRTH }
*
*/
public HISTTSBIRTH createHISTTSBIRTH() {
return new HISTTSBIRTH();
}
/**
* Create an instance of {@link DSETTSDATETIME }
*
*/
public DSETTSDATETIME createDSETTSDATETIME() {
return new DSETTSDATETIME();
}
/**
* Create an instance of {@link LISTTEL }
*
*/
public LISTTEL createLISTTEL() {
return new LISTTEL();
}
/**
* Create an instance of {@link UVPTS }
*
*/
public UVPTS createUVPTS() {
return new UVPTS();
}
/**
* Create an instance of {@link URGINT }
*
*/
public URGINT createURGINT() {
return new URGINT();
}
/**
* Create an instance of {@link QSITSBIRTH }
*
*/
public QSITSBIRTH createQSITSBIRTH() {
return new QSITSBIRTH();
}
/**
* Create an instance of {@link QSPPQ }
*
*/
public QSPPQ createQSPPQ() {
return new QSPPQ();
}
/**
* Create an instance of {@link PERSON }
*
*/
public PERSON createPERSON() {
return new PERSON();
}
/**
* Create an instance of {@link ADXPUNID }
*
*/
public ADXPUNID createADXPUNID() {
return new ADXPUNID();
}
/**
* Create an instance of {@link SCNT }
*
*/
public SCNT createSCNT() {
return new SCNT();
}
/**
* Create an instance of {@link DSETTSDATEFULL }
*
*/
public DSETTSDATEFULL createDSETTSDATEFULL() {
return new DSETTSDATEFULL();
}
/**
* Create an instance of {@link QSDMO }
*
*/
public QSDMO createQSDMO() {
return new QSDMO();
}
/**
* Create an instance of {@link DSETCS }
*
*/
public DSETCS createDSETCS() {
return new DSETCS();
}
/**
* Create an instance of {@link ENTN }
*
*/
public ENTN createENTN() {
return new ENTN();
}
/**
* Create an instance of {@link URGHIGHCO }
*
*/
public URGHIGHCO createURGHIGHCO() {
return new URGHIGHCO();
}
/**
* Create an instance of {@link ADXPADL }
*
*/
public ADXPADL createADXPADL() {
return new ADXPADL();
}
/**
* Create an instance of {@link QSUTS }
*
*/
public QSUTS createQSUTS() {
return new QSUTS();
}
/**
* Create an instance of {@link UVPEDSTRUCTUREDTEXT }
*
*/
public UVPEDSTRUCTUREDTEXT createUVPEDSTRUCTUREDTEXT() {
return new UVPEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link EIVLTSBIRTH }
*
*/
public EIVLTSBIRTH createEIVLTSBIRTH() {
return new EIVLTSBIRTH();
}
/**
* Create an instance of {@link BAGENPN }
*
*/
public BAGENPN createBAGENPN() {
return new BAGENPN();
}
/**
* Create an instance of {@link QSSTSDATE }
*
*/
public QSSTSDATE createQSSTSDATE() {
return new QSSTSDATE();
}
/**
* Create an instance of {@link ADXPCEN }
*
*/
public ADXPCEN createADXPCEN() {
return new ADXPCEN();
}
/**
* Create an instance of {@link LISTEDTEXT }
*
*/
public LISTEDTEXT createLISTEDTEXT() {
return new LISTEDTEXT();
}
/**
* Create an instance of {@link TELURL }
*
*/
public TELURL createTELURL() {
return new TELURL();
}
/**
* Create an instance of {@link HISTEDIMAGE }
*
*/
public HISTEDIMAGE createHISTEDIMAGE() {
return new HISTEDIMAGE();
}
/**
* Create an instance of {@link DSETTELPERSON }
*
*/
public DSETTELPERSON createDSETTELPERSON() {
return new DSETTELPERSON();
}
/**
* Create an instance of {@link HISTPQTIME }
*
*/
public HISTPQTIME createHISTPQTIME() {
return new HISTPQTIME();
}
/**
* Create an instance of {@link HISTSCNT }
*
*/
public HISTSCNT createHISTSCNT() {
return new HISTSCNT();
}
/**
* Create an instance of {@link NPPDTSDATETIMEFULL }
*
*/
public NPPDTSDATETIMEFULL createNPPDTSDATETIMEFULL() {
return new NPPDTSDATETIMEFULL();
}
/**
* Create an instance of {@link XReference }
*
*/
public XReference createXReference() {
return new XReference();
}
/**
* Create an instance of {@link LISTCDCV }
*
*/
public LISTCDCV createLISTCDCV() {
return new LISTCDCV();
}
/**
* Create an instance of {@link TSBIRTH }
*
*/
public TSBIRTH createTSBIRTH() {
return new TSBIRTH();
}
/**
* Create an instance of {@link URGHIGHTSBIRTH }
*
*/
public URGHIGHTSBIRTH createURGHIGHTSBIRTH() {
return new URGHIGHTSBIRTH();
}
/**
* Create an instance of {@link NPPDSC }
*
*/
public NPPDSC createNPPDSC() {
return new NPPDSC();
}
/**
* Create an instance of {@link NPPDEDDOCREF }
*
*/
public NPPDEDDOCREF createNPPDEDDOCREF() {
return new NPPDEDDOCREF();
}
/**
* Create an instance of {@link URGTS }
*
*/
public URGTS createURGTS() {
return new URGTS();
}
/**
* Create an instance of {@link UVPMO }
*
*/
public UVPMO createUVPMO() {
return new UVPMO();
}
/**
* Create an instance of {@link QSIRTO }
*
*/
public QSIRTO createQSIRTO() {
return new QSIRTO();
}
/**
* Create an instance of {@link IVLHIGHTSDATETIMEFULL }
*
*/
public IVLHIGHTSDATETIMEFULL createIVLHIGHTSDATETIMEFULL() {
return new IVLHIGHTSDATETIMEFULL();
}
/**
* Create an instance of {@link ADXPDINST }
*
*/
public ADXPDINST createADXPDINST() {
return new ADXPDINST();
}
/**
* Create an instance of {@link EDSTRUCTUREDTITLE }
*
*/
public EDSTRUCTUREDTITLE createEDSTRUCTUREDTITLE() {
return new EDSTRUCTUREDTITLE();
}
/**
* Create an instance of {@link NPPDEDDOCINLINE }
*
*/
public NPPDEDDOCINLINE createNPPDEDDOCINLINE() {
return new NPPDEDDOCINLINE();
}
/**
* Create an instance of {@link URGLOWPQ }
*
*/
public URGLOWPQ createURGLOWPQ() {
return new URGLOWPQ();
}
/**
* Create an instance of {@link URGLOWTSBIRTH }
*
*/
public URGLOWTSBIRTH createURGLOWTSBIRTH() {
return new URGLOWTSBIRTH();
}
/**
* Create an instance of {@link AD }
*
*/
public AD createAD() {
return new AD();
}
/**
* Create an instance of {@link QSUREAL }
*
*/
public QSUREAL createQSUREAL() {
return new QSUREAL();
}
/**
* Create an instance of {@link SLISTPQTIME }
*
*/
public SLISTPQTIME createSLISTPQTIME() {
return new SLISTPQTIME();
}
/**
* Create an instance of {@link ADXPSAL }
*
*/
public ADXPSAL createADXPSAL() {
return new ADXPSAL();
}
/**
* Create an instance of {@link HISTTELPERSON }
*
*/
public HISTTELPERSON createHISTTELPERSON() {
return new HISTTELPERSON();
}
/**
* Create an instance of {@link HISTEDDOCINLINE }
*
*/
public HISTEDDOCINLINE createHISTEDDOCINLINE() {
return new HISTEDDOCINLINE();
}
/**
* Create an instance of {@link HISTEDDOCREF }
*
*/
public HISTEDDOCREF createHISTEDDOCREF() {
return new HISTEDDOCREF();
}
/**
* Create an instance of {@link NPPDSTNT }
*
*/
public NPPDSTNT createNPPDSTNT() {
return new NPPDSTNT();
}
/**
* Create an instance of {@link QSUPQTIME }
*
*/
public QSUPQTIME createQSUPQTIME() {
return new QSUPQTIME();
}
/**
* Create an instance of {@link IVLPQTIME }
*
*/
public IVLPQTIME createIVLPQTIME() {
return new IVLPQTIME();
}
/**
* Create an instance of {@link SLISTINTPOS }
*
*/
public SLISTINTPOS createSLISTINTPOS() {
return new SLISTINTPOS();
}
/**
* Create an instance of {@link BAGRTO }
*
*/
public BAGRTO createBAGRTO() {
return new BAGRTO();
}
/**
* Create an instance of {@link NPPDPQ }
*
*/
public NPPDPQ createNPPDPQ() {
return new NPPDPQ();
}
/**
* Create an instance of {@link StrucDocFootnoteRef }
*
*/
public StrucDocFootnoteRef createStrucDocFootnoteRef() {
return new StrucDocFootnoteRef();
}
/**
* Create an instance of {@link IVLWIDTHINT }
*
*/
public IVLWIDTHINT createIVLWIDTHINT() {
return new IVLWIDTHINT();
}
/**
* Create an instance of {@link BAGINTNONNEG }
*
*/
public BAGINTNONNEG createBAGINTNONNEG() {
return new BAGINTNONNEG();
}
/**
* Create an instance of {@link BAGTSDATETIMEFULL }
*
*/
public BAGTSDATETIMEFULL createBAGTSDATETIMEFULL() {
return new BAGTSDATETIMEFULL();
}
/**
* Create an instance of {@link ENPN }
*
*/
public ENPN createENPN() {
return new ENPN();
}
/**
* Create an instance of {@link IVLINTNONNEG }
*
*/
public IVLINTNONNEG createIVLINTNONNEG() {
return new IVLINTNONNEG();
}
/**
* Create an instance of {@link QSSTS }
*
*/
public QSSTS createQSSTS() {
return new QSSTS();
}
/**
* Create an instance of {@link QSDTSDATEFULL }
*
*/
public QSDTSDATEFULL createQSDTSDATEFULL() {
return new QSDTSDATEFULL();
}
/**
* Create an instance of {@link DSETPQTIME }
*
*/
public DSETPQTIME createDSETPQTIME() {
return new DSETPQTIME();
}
/**
* Create an instance of {@link LINK }
*
*/
public LINK createLINK() {
return new LINK();
}
/**
* Create an instance of {@link IVLWIDTHTSDATETIME }
*
*/
public IVLWIDTHTSDATETIME createIVLWIDTHTSDATETIME() {
return new IVLWIDTHTSDATETIME();
}
/**
* Create an instance of {@link DSETEDTEXT }
*
*/
public DSETEDTEXT createDSETEDTEXT() {
return new DSETEDTEXT();
}
/**
* Create an instance of {@link BAGTELPERSON }
*
*/
public BAGTELPERSON createBAGTELPERSON() {
return new BAGTELPERSON();
}
/**
* Create an instance of {@link QSURTO }
*
*/
public QSURTO createQSURTO() {
return new QSURTO();
}
/**
* Create an instance of {@link BL }
*
*/
public BL createBL() {
return new BL();
}
/**
* Create an instance of {@link URGRTO }
*
*/
public URGRTO createURGRTO() {
return new URGRTO();
}
/**
* Create an instance of {@link BAGENON }
*
*/
public BAGENON createBAGENON() {
return new BAGENON();
}
/**
* Create an instance of {@link EDDOCINLINE }
*
*/
public EDDOCINLINE createEDDOCINLINE() {
return new EDDOCINLINE();
}
/**
* Create an instance of {@link DSETEDSTRUCTUREDTITLE }
*
*/
public DSETEDSTRUCTUREDTITLE createDSETEDSTRUCTUREDTITLE() {
return new DSETEDSTRUCTUREDTITLE();
}
/**
* Create an instance of {@link CS }
*
*/
public CS createCS() {
return new CS();
}
/**
* Create an instance of {@link URGLOWTSDATEFULL }
*
*/
public URGLOWTSDATEFULL createURGLOWTSDATEFULL() {
return new URGLOWTSDATEFULL();
}
/**
* Create an instance of {@link ENON }
*
*/
public ENON createENON() {
return new ENON();
}
/**
* Create an instance of {@link SLISTPQ }
*
*/
public SLISTPQ createSLISTPQ() {
return new SLISTPQ();
}
/**
* Create an instance of {@link HEALTHCAREPROFESSIONALROLE }
*
*/
public HEALTHCAREPROFESSIONALROLE createHEALTHCAREPROFESSIONALROLE() {
return new HEALTHCAREPROFESSIONALROLE();
}
/**
* Create an instance of {@link StrucDocCaptioned }
*
*/
public StrucDocCaptioned createStrucDocCaptioned() {
return new StrucDocCaptioned();
}
/**
* Create an instance of {@link URGLOWINTPOS }
*
*/
public URGLOWINTPOS createURGLOWINTPOS() {
return new URGLOWINTPOS();
}
/**
* Create an instance of {@link PIVLTSBIRTH }
*
*/
public PIVLTSBIRTH createPIVLTSBIRTH() {
return new PIVLTSBIRTH();
}
/**
* Create an instance of {@link IVLHIGHINTPOS }
*
*/
public IVLHIGHINTPOS createIVLHIGHINTPOS() {
return new IVLHIGHINTPOS();
}
/**
* Create an instance of {@link UVPPQTIME }
*
*/
public UVPPQTIME createUVPPQTIME() {
return new UVPPQTIME();
}
/**
* Create an instance of {@link BAGTELURL }
*
*/
public BAGTELURL createBAGTELURL() {
return new BAGTELURL();
}
/**
* Create an instance of {@link QSUPQ }
*
*/
public QSUPQ createQSUPQ() {
return new QSUPQ();
}
/**
* Create an instance of {@link StrucDocParagraph }
*
*/
public StrucDocParagraph createStrucDocParagraph() {
return new StrucDocParagraph();
}
/**
* Create an instance of {@link QSITSDATETIME }
*
*/
public QSITSDATETIME createQSITSDATETIME() {
return new QSITSDATETIME();
}
/**
* Create an instance of {@link QSIINT }
*
*/
public QSIINT createQSIINT() {
return new QSIINT();
}
/**
* Create an instance of {@link CD }
*
*/
public CD createCD() {
return new CD();
}
/**
* Create an instance of {@link QSPTS }
*
*/
public QSPTS createQSPTS() {
return new QSPTS();
}
/**
* Create an instance of {@link LISTSCNT }
*
*/
public LISTSCNT createLISTSCNT() {
return new LISTSCNT();
}
/**
* Create an instance of {@link UVPPQ }
*
*/
public UVPPQ createUVPPQ() {
return new UVPPQ();
}
/**
* Create an instance of {@link CO }
*
*/
public CO createCO() {
return new CO();
}
/**
* Create an instance of {@link QSSRTO }
*
*/
public QSSRTO createQSSRTO() {
return new QSSRTO();
}
/**
* Create an instance of {@link QSIPQTIME }
*
*/
public QSIPQTIME createQSIPQTIME() {
return new QSIPQTIME();
}
/**
* Create an instance of {@link IVLHIGHINTNONNEG }
*
*/
public IVLHIGHINTNONNEG createIVLHIGHINTNONNEG() {
return new IVLHIGHINTNONNEG();
}
/**
* Create an instance of {@link QSSINTNONNEG }
*
*/
public QSSINTNONNEG createQSSINTNONNEG() {
return new QSSINTNONNEG();
}
/**
* Create an instance of {@link StrucDocTable }
*
*/
public StrucDocTable createStrucDocTable() {
return new StrucDocTable();
}
/**
* Create an instance of {@link URGPQTIME }
*
*/
public URGPQTIME createURGPQTIME() {
return new URGPQTIME();
}
/**
* Create an instance of {@link ATTESTATIONINFO }
*
*/
public ATTESTATIONINFO createATTESTATIONINFO() {
return new ATTESTATIONINFO();
}
/**
* Create an instance of {@link FOLDER }
*
*/
public FOLDER createFOLDER() {
return new FOLDER();
}
/**
* Create an instance of {@link LISTAD }
*
*/
public LISTAD createLISTAD() {
return new LISTAD();
}
/**
* Create an instance of {@link UVPINTPOS }
*
*/
public UVPINTPOS createUVPINTPOS() {
return new UVPINTPOS();
}
/**
* Create an instance of {@link HISTEDSIGNATURE }
*
*/
public HISTEDSIGNATURE createHISTEDSIGNATURE() {
return new HISTEDSIGNATURE();
}
/**
* Create an instance of {@link HISTEDTEXT }
*
*/
public HISTEDTEXT createHISTEDTEXT() {
return new HISTEDTEXT();
}
/**
* Create an instance of {@link DSETEDDOCREF }
*
*/
public DSETEDDOCREF createDSETEDDOCREF() {
return new DSETEDDOCREF();
}
/**
* Create an instance of {@link QSICO }
*
*/
public QSICO createQSICO() {
return new QSICO();
}
/**
* Create an instance of {@link LISTTELEMAIL }
*
*/
public LISTTELEMAIL createLISTTELEMAIL() {
return new LISTTELEMAIL();
}
/**
* Create an instance of {@link HISTPQ }
*
*/
public HISTPQ createHISTPQ() {
return new HISTPQ();
}
/**
* Create an instance of {@link StrucDocFootnote }
*
*/
public StrucDocFootnote createStrucDocFootnote() {
return new StrucDocFootnote();
}
/**
* Create an instance of {@link UVPINT }
*
*/
public UVPINT createUVPINT() {
return new UVPINT();
}
/**
* Create an instance of {@link DSETINTPOS }
*
*/
public DSETINTPOS createDSETINTPOS() {
return new DSETINTPOS();
}
/**
* Create an instance of {@link EDIMAGE }
*
*/
public EDIMAGE createEDIMAGE() {
return new EDIMAGE();
}
/**
* Create an instance of {@link QSITSDATETIMEFULL }
*
*/
public QSITSDATETIMEFULL createQSITSDATETIMEFULL() {
return new QSITSDATETIMEFULL();
}
/**
* Create an instance of {@link IVLWIDTHINTNONNEG }
*
*/
public IVLWIDTHINTNONNEG createIVLWIDTHINTNONNEG() {
return new IVLWIDTHINTNONNEG();
}
/**
* Create an instance of {@link GLISTRTO }
*
*/
public GLISTRTO createGLISTRTO() {
return new GLISTRTO();
}
/**
* Create an instance of {@link NPPDEN }
*
*/
public NPPDEN createNPPDEN() {
return new NPPDEN();
}
/**
* Create an instance of {@link QSIINTPOS }
*
*/
public QSIINTPOS createQSIINTPOS() {
return new QSIINTPOS();
}
/**
* Create an instance of {@link IVLTSDATETIMEFULL }
*
*/
public IVLTSDATETIMEFULL createIVLTSDATETIMEFULL() {
return new IVLTSDATETIMEFULL();
}
/**
* Create an instance of {@link MO }
*
*/
public MO createMO() {
return new MO();
}
/**
* Create an instance of {@link URGLOWTSDATETIME }
*
*/
public URGLOWTSDATETIME createURGLOWTSDATETIME() {
return new URGLOWTSDATETIME();
}
/**
* Create an instance of {@link PIVLTSDATETIMEFULL }
*
*/
public PIVLTSDATETIMEFULL createPIVLTSDATETIMEFULL() {
return new PIVLTSDATETIMEFULL();
}
/**
* Create an instance of {@link URGINTPOS }
*
*/
public URGINTPOS createURGINTPOS() {
return new URGINTPOS();
}
/**
* Create an instance of {@link IVLINTPOS }
*
*/
public IVLINTPOS createIVLINTPOS() {
return new IVLINTPOS();
}
/**
* Create an instance of {@link UVPSTNT }
*
*/
public UVPSTNT createUVPSTNT() {
return new UVPSTNT();
}
/**
* Create an instance of {@link NPPDEDTEXT }
*
*/
public NPPDEDTEXT createNPPDEDTEXT() {
return new NPPDEDTEXT();
}
/**
* Create an instance of {@link IVLLOWTSDATE }
*
*/
public IVLLOWTSDATE createIVLLOWTSDATE() {
return new IVLLOWTSDATE();
}
/**
* Create an instance of {@link NPPDED }
*
*/
public NPPDED createNPPDED() {
return new NPPDED();
}
/**
* Create an instance of {@link DSETII }
*
*/
public DSETII createDSETII() {
return new DSETII();
}
/**
* Create an instance of {@link SLISTINTNONNEG }
*
*/
public SLISTINTNONNEG createSLISTINTNONNEG() {
return new SLISTINTNONNEG();
}
/**
* Create an instance of {@link IVLLOWRTO }
*
*/
public IVLLOWRTO createIVLLOWRTO() {
return new IVLLOWRTO();
}
/**
* Create an instance of {@link UVPTELURL }
*
*/
public UVPTELURL createUVPTELURL() {
return new UVPTELURL();
}
/**
* Create an instance of {@link PIVLTSDATE }
*
*/
public PIVLTSDATE createPIVLTSDATE() {
return new PIVLTSDATE();
}
/**
* Create an instance of {@link QSDTSBIRTH }
*
*/
public QSDTSBIRTH createQSDTSBIRTH() {
return new QSDTSBIRTH();
}
/**
* Create an instance of {@link SECTION }
*
*/
public SECTION createSECTION() {
return new SECTION();
}
/**
* Create an instance of {@link QSSPQ }
*
*/
public QSSPQ createQSSPQ() {
return new QSSPQ();
}
/**
* Create an instance of {@link UVPREAL }
*
*/
public UVPREAL createUVPREAL() {
return new UVPREAL();
}
/**
* Create an instance of {@link QSUMO }
*
*/
public QSUMO createQSUMO() {
return new QSUMO();
}
/**
* Create an instance of {@link HISTTELPHONE }
*
*/
public HISTTELPHONE createHISTTELPHONE() {
return new HISTTELPHONE();
}
/**
* Create an instance of {@link UVPTSDATEFULL }
*
*/
public UVPTSDATEFULL createUVPTSDATEFULL() {
return new UVPTSDATEFULL();
}
/**
* Create an instance of {@link BAGCDCV }
*
*/
public BAGCDCV createBAGCDCV() {
return new BAGCDCV();
}
/**
* Create an instance of {@link IVLHIGHPQ }
*
*/
public IVLHIGHPQ createIVLHIGHPQ() {
return new IVLHIGHPQ();
}
/**
* Create an instance of {@link NPPDINTPOS }
*
*/
public NPPDINTPOS createNPPDINTPOS() {
return new NPPDINTPOS();
}
/**
* Create an instance of {@link NPPDTELPERSON }
*
*/
public NPPDTELPERSON createNPPDTELPERSON() {
return new NPPDTELPERSON();
}
/**
* Create an instance of {@link UVPTELEMAIL }
*
*/
public UVPTELEMAIL createUVPTELEMAIL() {
return new UVPTELEMAIL();
}
/**
* Create an instance of {@link TEL }
*
*/
public TEL createTEL() {
return new TEL();
}
/**
* Create an instance of {@link GLISTCO }
*
*/
public GLISTCO createGLISTCO() {
return new GLISTCO();
}
/**
* Create an instance of {@link URGLOWRTO }
*
*/
public URGLOWRTO createURGLOWRTO() {
return new URGLOWRTO();
}
/**
* Create an instance of {@link HISTRTO }
*
*/
public HISTRTO createHISTRTO() {
return new HISTRTO();
}
/**
* Create an instance of {@link NPPDCO }
*
*/
public NPPDCO createNPPDCO() {
return new NPPDCO();
}
/**
* Create an instance of {@link QSPREAL }
*
*/
public QSPREAL createQSPREAL() {
return new QSPREAL();
}
/**
* Create an instance of {@link QSUTSDATETIMEFULL }
*
*/
public QSUTSDATETIMEFULL createQSUTSDATETIMEFULL() {
return new QSUTSDATETIMEFULL();
}
/**
* Create an instance of {@link NPPDCS }
*
*/
public NPPDCS createNPPDCS() {
return new NPPDCS();
}
/**
* Create an instance of {@link GLISTINTPOS }
*
*/
public GLISTINTPOS createGLISTINTPOS() {
return new GLISTINTPOS();
}
/**
* Create an instance of {@link NPPDRTO }
*
*/
public NPPDRTO createNPPDRTO() {
return new NPPDRTO();
}
/**
* Create an instance of {@link IVLHIGHTSDATETIME }
*
*/
public IVLHIGHTSDATETIME createIVLHIGHTSDATETIME() {
return new IVLHIGHTSDATETIME();
}
/**
* Create an instance of {@link ADXPCTY }
*
*/
public ADXPCTY createADXPCTY() {
return new ADXPCTY();
}
/**
* Create an instance of {@link HISTENTN }
*
*/
public HISTENTN createHISTENTN() {
return new HISTENTN();
}
/**
* Create an instance of {@link DSETINT }
*
*/
public DSETINT createDSETINT() {
return new DSETINT();
}
/**
* Create an instance of {@link NPPDCD }
*
*/
public NPPDCD createNPPDCD() {
return new NPPDCD();
}
/**
* Create an instance of {@link UVPEDDOC }
*
*/
public UVPEDDOC createUVPEDDOC() {
return new UVPEDDOC();
}
/**
* Create an instance of {@link QSDCO }
*
*/
public QSDCO createQSDCO() {
return new QSDCO();
}
/**
* Create an instance of {@link QSSREAL }
*
*/
public QSSREAL createQSSREAL() {
return new QSSREAL();
}
/**
* Create an instance of {@link LISTED }
*
*/
public LISTED createLISTED() {
return new LISTED();
}
/**
* Create an instance of {@link GLISTTSDATEFULL }
*
*/
public GLISTTSDATEFULL createGLISTTSDATEFULL() {
return new GLISTTSDATEFULL();
}
/**
* Create an instance of {@link LISTEDSTRUCTUREDTEXT }
*
*/
public LISTEDSTRUCTUREDTEXT createLISTEDSTRUCTUREDTEXT() {
return new LISTEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link BAGTSDATETIME }
*
*/
public BAGTSDATETIME createBAGTSDATETIME() {
return new BAGTSDATETIME();
}
/**
* Create an instance of {@link StrucDocList }
*
*/
public StrucDocList createStrucDocList() {
return new StrucDocList();
}
/**
* Create an instance of {@link QSDTS }
*
*/
public QSDTS createQSDTS() {
return new QSDTS();
}
/**
* Create an instance of {@link IVLLOWTSDATEFULL }
*
*/
public IVLLOWTSDATEFULL createIVLLOWTSDATEFULL() {
return new IVLLOWTSDATEFULL();
}
/**
* Create an instance of {@link HISTINT }
*
*/
public HISTINT createHISTINT() {
return new HISTINT();
}
/**
* Create an instance of {@link QSDREAL }
*
*/
public QSDREAL createQSDREAL() {
return new QSDREAL();
}
/**
* Create an instance of {@link UVPEDTEXT }
*
*/
public UVPEDTEXT createUVPEDTEXT() {
return new UVPEDTEXT();
}
/**
* Create an instance of {@link IVLHIGHTSDATEFULL }
*
*/
public IVLHIGHTSDATEFULL createIVLHIGHTSDATEFULL() {
return new IVLHIGHTSDATEFULL();
}
/**
* Create an instance of {@link ADXPPOB }
*
*/
public ADXPPOB createADXPPOB() {
return new ADXPPOB();
}
/**
* Create an instance of {@link LISTEN }
*
*/
public LISTEN createLISTEN() {
return new LISTEN();
}
/**
* Create an instance of {@link CDCV }
*
*/
public CDCV createCDCV() {
return new CDCV();
}
/**
* Create an instance of {@link LISTTELURL }
*
*/
public LISTTELURL createLISTTELURL() {
return new LISTTELURL();
}
/**
* Create an instance of {@link HISTTS }
*
*/
public HISTTS createHISTTS() {
return new HISTTS();
}
/**
* Create an instance of {@link IVLTSDATEFULL }
*
*/
public IVLTSDATEFULL createIVLTSDATEFULL() {
return new IVLTSDATEFULL();
}
/**
* Create an instance of {@link URGLOWTSDATE }
*
*/
public URGLOWTSDATE createURGLOWTSDATE() {
return new URGLOWTSDATE();
}
/**
* Create an instance of {@link II }
*
*/
public II createII() {
return new II();
}
/**
* Create an instance of {@link HISTSC }
*
*/
public HISTSC createHISTSC() {
return new HISTSC();
}
/**
* Create an instance of {@link URGMO }
*
*/
public URGMO createURGMO() {
return new URGMO();
}
/**
* Create an instance of {@link QSDPQTIME }
*
*/
public QSDPQTIME createQSDPQTIME() {
return new QSDPQTIME();
}
/**
* Create an instance of {@link URGHIGHINTNONNEG }
*
*/
public URGHIGHINTNONNEG createURGHIGHINTNONNEG() {
return new URGHIGHINTNONNEG();
}
/**
* Create an instance of {@link QSIINTNONNEG }
*
*/
public QSIINTNONNEG createQSIINTNONNEG() {
return new QSIINTNONNEG();
}
/**
* Create an instance of {@link QSDTSDATETIME }
*
*/
public QSDTSDATETIME createQSDTSDATETIME() {
return new QSDTSDATETIME();
}
/**
* Create an instance of {@link StrucDocCol }
*
*/
public StrucDocCol createStrucDocCol() {
return new StrucDocCol();
}
/**
* Create an instance of {@link QSDINTPOS }
*
*/
public QSDINTPOS createQSDINTPOS() {
return new QSDINTPOS();
}
/**
* Create an instance of {@link IVLINT }
*
*/
public IVLINT createIVLINT() {
return new IVLINT();
}
/**
* Create an instance of {@link IVLLOWTS }
*
*/
public IVLLOWTS createIVLLOWTS() {
return new IVLLOWTS();
}
/**
* Create an instance of {@link IVLWIDTHTSBIRTH }
*
*/
public IVLWIDTHTSBIRTH createIVLWIDTHTSBIRTH() {
return new IVLWIDTHTSBIRTH();
}
/**
* Create an instance of {@link IVLTSDATE }
*
*/
public IVLTSDATE createIVLTSDATE() {
return new IVLTSDATE();
}
/**
* Create an instance of {@link ADXPBNN }
*
*/
public ADXPBNN createADXPBNN() {
return new ADXPBNN();
}
/**
* Create an instance of {@link HISTST }
*
*/
public HISTST createHISTST() {
return new HISTST();
}
/**
* Create an instance of {@link ADXPBNR }
*
*/
public ADXPBNR createADXPBNR() {
return new ADXPBNR();
}
/**
* Create an instance of {@link NPPDII }
*
*/
public NPPDII createNPPDII() {
return new NPPDII();
}
/**
* Create an instance of {@link DSETED }
*
*/
public DSETED createDSETED() {
return new DSETED();
}
/**
* Create an instance of {@link ADXPBNS }
*
*/
public ADXPBNS createADXPBNS() {
return new ADXPBNS();
}
/**
* Create an instance of {@link SLISTTSDATEFULL }
*
*/
public SLISTTSDATEFULL createSLISTTSDATEFULL() {
return new SLISTTSDATEFULL();
}
/**
* Create an instance of {@link DSETSTNT }
*
*/
public DSETSTNT createDSETSTNT() {
return new DSETSTNT();
}
/**
* Create an instance of {@link UVPTSDATETIME }
*
*/
public UVPTSDATETIME createUVPTSDATETIME() {
return new UVPTSDATETIME();
}
/**
* Create an instance of {@link ADXPCNT }
*
*/
public ADXPCNT createADXPCNT() {
return new ADXPCNT();
}
/**
* Create an instance of {@link URGLOWINTNONNEG }
*
*/
public URGLOWINTNONNEG createURGLOWINTNONNEG() {
return new URGLOWINTNONNEG();
}
/**
* Create an instance of {@link DSETEN }
*
*/
public DSETEN createDSETEN() {
return new DSETEN();
}
/**
* Create an instance of {@link StrucDocTableItem }
*
*/
public StrucDocTableItem createStrucDocTableItem() {
return new StrucDocTableItem();
}
/**
* Create an instance of {@link NPPDTELEMAIL }
*
*/
public NPPDTELEMAIL createNPPDTELEMAIL() {
return new NPPDTELEMAIL();
}
/**
* Create an instance of {@link EIVLTSDATEFULL }
*
*/
public EIVLTSDATEFULL createEIVLTSDATEFULL() {
return new EIVLTSDATEFULL();
}
/**
* Create an instance of {@link HISTSTNT }
*
*/
public HISTSTNT createHISTSTNT() {
return new HISTSTNT();
}
/**
* Create an instance of {@link GLISTINT }
*
*/
public GLISTINT createGLISTINT() {
return new GLISTINT();
}
/**
* Create an instance of {@link LISTCD }
*
*/
public LISTCD createLISTCD() {
return new LISTCD();
}
/**
* Create an instance of {@link DSETEDSTRUCTUREDTEXT }
*
*/
public DSETEDSTRUCTUREDTEXT createDSETEDSTRUCTUREDTEXT() {
return new DSETEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link LISTCO }
*
*/
public LISTCO createLISTCO() {
return new LISTCO();
}
/**
* Create an instance of {@link LISTTSDATETIME }
*
*/
public LISTTSDATETIME createLISTTSDATETIME() {
return new LISTTSDATETIME();
}
/**
* Create an instance of {@link ADXPDINSTA }
*
*/
public ADXPDINSTA createADXPDINSTA() {
return new ADXPDINSTA();
}
/**
* Create an instance of {@link IVLHIGHREAL }
*
*/
public IVLHIGHREAL createIVLHIGHREAL() {
return new IVLHIGHREAL();
}
/**
* Create an instance of {@link UVPRTO }
*
*/
public UVPRTO createUVPRTO() {
return new UVPRTO();
}
/**
* Create an instance of {@link BAGST }
*
*/
public BAGST createBAGST() {
return new BAGST();
}
/**
* Create an instance of {@link ADXPINT }
*
*/
public ADXPINT createADXPINT() {
return new ADXPINT();
}
/**
* Create an instance of {@link BAGSC }
*
*/
public BAGSC createBAGSC() {
return new BAGSC();
}
/**
* Create an instance of {@link LISTCS }
*
*/
public LISTCS createLISTCS() {
return new LISTCS();
}
/**
* Create an instance of {@link LISTTSDATETIMEFULL }
*
*/
public LISTTSDATETIMEFULL createLISTTSDATETIMEFULL() {
return new LISTTSDATETIMEFULL();
}
/**
* Create an instance of {@link StrucDocTitle }
*
*/
public StrucDocTitle createStrucDocTitle() {
return new StrucDocTitle();
}
/**
* Create an instance of {@link URGLOWCO }
*
*/
public URGLOWCO createURGLOWCO() {
return new URGLOWCO();
}
/**
* Create an instance of {@link QSSTSDATEFULL }
*
*/
public QSSTSDATEFULL createQSSTSDATEFULL() {
return new QSSTSDATEFULL();
}
/**
* Create an instance of {@link ADXPDINSTQ }
*
*/
public ADXPDINSTQ createADXPDINSTQ() {
return new ADXPDINSTQ();
}
/**
* Create an instance of {@link StrucDocCMTitle }
*
*/
public StrucDocCMTitle createStrucDocCMTitle() {
return new StrucDocCMTitle();
}
/**
* Create an instance of {@link StrucDocColItem }
*
*/
public StrucDocColItem createStrucDocColItem() {
return new StrucDocColItem();
}
/**
* Create an instance of {@link UVPEDDOCREF }
*
*/
public UVPEDDOCREF createUVPEDDOCREF() {
return new UVPEDDOCREF();
}
/**
* Create an instance of {@link BAGTS }
*
*/
public BAGTS createBAGTS() {
return new BAGTS();
}
/**
* Create an instance of {@link QSUTSDATE }
*
*/
public QSUTSDATE createQSUTSDATE() {
return new QSUTSDATE();
}
/**
* Create an instance of {@link IVLHIGHMO }
*
*/
public IVLHIGHMO createIVLHIGHMO() {
return new IVLHIGHMO();
}
/**
* Create an instance of {@link ADXPCPA }
*
*/
public ADXPCPA createADXPCPA() {
return new ADXPCPA();
}
/**
* Create an instance of {@link QSUINTPOS }
*
*/
public QSUINTPOS createQSUINTPOS() {
return new QSUINTPOS();
}
/**
* Create an instance of {@link BAGINT }
*
*/
public BAGINT createBAGINT() {
return new BAGINT();
}
/**
* Create an instance of {@link LISTBL }
*
*/
public LISTBL createLISTBL() {
return new LISTBL();
}
/**
* Create an instance of {@link ADXPPRE }
*
*/
public ADXPPRE createADXPPRE() {
return new ADXPPRE();
}
/**
* Create an instance of {@link StrucDocColGroup }
*
*/
public StrucDocColGroup createStrucDocColGroup() {
return new StrucDocColGroup();
}
/**
* Create an instance of {@link IVLWIDTHCO }
*
*/
public IVLWIDTHCO createIVLWIDTHCO() {
return new IVLWIDTHCO();
}
/**
* Create an instance of {@link CLUSTER }
*
*/
public CLUSTER createCLUSTER() {
return new CLUSTER();
}
/**
* Create an instance of {@link StrucDocContent }
*
*/
public StrucDocContent createStrucDocContent() {
return new StrucDocContent();
}
/**
* Create an instance of {@link IVLLOWINT }
*
*/
public IVLLOWINT createIVLLOWINT() {
return new IVLLOWINT();
}
/**
* Create an instance of {@link SLISTTSDATETIMEFULL }
*
*/
public SLISTTSDATETIMEFULL createSLISTTSDATETIMEFULL() {
return new SLISTTSDATETIMEFULL();
}
/**
* Create an instance of {@link HISTENPN }
*
*/
public HISTENPN createHISTENPN() {
return new HISTENPN();
}
/**
* Create an instance of {@link TELPHONE }
*
*/
public TELPHONE createTELPHONE() {
return new TELPHONE();
}
/**
* Create an instance of {@link QSSMO }
*
*/
public QSSMO createQSSMO() {
return new QSSMO();
}
/**
* Create an instance of {@link ADXPDMODID }
*
*/
public ADXPDMODID createADXPDMODID() {
return new ADXPDMODID();
}
/**
* Create an instance of {@link HISTII }
*
*/
public HISTII createHISTII() {
return new HISTII();
}
/**
* Create an instance of {@link DSETTSDATETIMEFULL }
*
*/
public DSETTSDATETIMEFULL createDSETTSDATETIMEFULL() {
return new DSETTSDATETIMEFULL();
}
/**
* Create an instance of {@link QSIREAL }
*
*/
public QSIREAL createQSIREAL() {
return new QSIREAL();
}
/**
* Create an instance of {@link DSETST }
*
*/
public DSETST createDSETST() {
return new DSETST();
}
/**
* Create an instance of {@link NPPDEDIMAGE }
*
*/
public NPPDEDIMAGE createNPPDEDIMAGE() {
return new NPPDEDIMAGE();
}
/**
* Create an instance of {@link URGTSDATEFULL }
*
*/
public URGTSDATEFULL createURGTSDATEFULL() {
return new URGTSDATEFULL();
}
/**
* Create an instance of {@link NPPDTEL }
*
*/
public NPPDTEL createNPPDTEL() {
return new NPPDTEL();
}
/**
* Create an instance of {@link IVLHIGHTSDATE }
*
*/
public IVLHIGHTSDATE createIVLHIGHTSDATE() {
return new IVLHIGHTSDATE();
}
/**
* Create an instance of {@link URGHIGHTS }
*
*/
public URGHIGHTS createURGHIGHTS() {
return new URGHIGHTS();
}
/**
* Create an instance of {@link NPPDENON }
*
*/
public NPPDENON createNPPDENON() {
return new NPPDENON();
}
/**
* Create an instance of {@link LISTPQTIME }
*
*/
public LISTPQTIME createLISTPQTIME() {
return new LISTPQTIME();
}
/**
* Create an instance of {@link URGTSBIRTH }
*
*/
public URGTSBIRTH createURGTSBIRTH() {
return new URGTSBIRTH();
}
/**
* Create an instance of {@link UVPBL }
*
*/
public UVPBL createUVPBL() {
return new UVPBL();
}
/**
* Create an instance of {@link URGREAL }
*
*/
public URGREAL createURGREAL() {
return new URGREAL();
}
/**
* Create an instance of {@link QSIMO }
*
*/
public QSIMO createQSIMO() {
return new QSIMO();
}
/**
* Create an instance of {@link IVLLOWTSBIRTH }
*
*/
public IVLLOWTSBIRTH createIVLLOWTSBIRTH() {
return new IVLLOWTSBIRTH();
}
/**
* Create an instance of {@link HISTENON }
*
*/
public HISTENON createHISTENON() {
return new HISTENON();
}
/**
* Create an instance of {@link URGHIGHTSDATETIME }
*
*/
public URGHIGHTSDATETIME createURGHIGHTSDATETIME() {
return new URGHIGHTSDATETIME();
}
/**
* Create an instance of {@link URGTSDATE }
*
*/
public URGTSDATE createURGTSDATE() {
return new URGTSDATE();
}
/**
* Create an instance of {@link LISTINTNONNEG }
*
*/
public LISTINTNONNEG createLISTINTNONNEG() {
return new LISTINTNONNEG();
}
/**
* Create an instance of {@link IVLWIDTHINTPOS }
*
*/
public IVLWIDTHINTPOS createIVLWIDTHINTPOS() {
return new IVLWIDTHINTPOS();
}
/**
* Create an instance of {@link DSETTS }
*
*/
public DSETTS createDSETTS() {
return new DSETTS();
}
/**
* Create an instance of {@link SOFTWAREORDEVICE }
*
*/
public SOFTWAREORDEVICE createSOFTWAREORDEVICE() {
return new SOFTWAREORDEVICE();
}
/**
* Create an instance of {@link HISTEDDOC }
*
*/
public HISTEDDOC createHISTEDDOC() {
return new HISTEDDOC();
}
/**
* Create an instance of {@link IVLTS }
*
*/
public IVLTS createIVLTS() {
return new IVLTS();
}
/**
* Create an instance of {@link PIVLTS }
*
*/
public PIVLTS createPIVLTS() {
return new PIVLTS();
}
/**
* Create an instance of {@link BAGEDSIGNATURE }
*
*/
public BAGEDSIGNATURE createBAGEDSIGNATURE() {
return new BAGEDSIGNATURE();
}
/**
* Create an instance of {@link UVPEDDOCINLINE }
*
*/
public UVPEDDOCINLINE createUVPEDDOCINLINE() {
return new UVPEDDOCINLINE();
}
/**
* Create an instance of {@link NPPDSCNT }
*
*/
public NPPDSCNT createNPPDSCNT() {
return new NPPDSCNT();
}
/**
* Create an instance of {@link QSUCO }
*
*/
public QSUCO createQSUCO() {
return new QSUCO();
}
/**
* Create an instance of {@link URGHIGHTSDATEFULL }
*
*/
public URGHIGHTSDATEFULL createURGHIGHTSDATEFULL() {
return new URGHIGHTSDATEFULL();
}
/**
* Create an instance of {@link UVPAD }
*
*/
public UVPAD createUVPAD() {
return new UVPAD();
}
/**
* Create an instance of {@link SLISTTSDATE }
*
*/
public SLISTTSDATE createSLISTTSDATE() {
return new SLISTTSDATE();
}
/**
* Create an instance of {@link UVPBLNONNULL }
*
*/
public UVPBLNONNULL createUVPBLNONNULL() {
return new UVPBLNONNULL();
}
/**
* Create an instance of {@link GLISTTSDATETIME }
*
*/
public GLISTTSDATETIME createGLISTTSDATETIME() {
return new GLISTTSDATETIME();
}
/**
* Create an instance of {@link URGINTNONNEG }
*
*/
public URGINTNONNEG createURGINTNONNEG() {
return new URGINTNONNEG();
}
/**
* Create an instance of {@link QSPTSDATETIMEFULL }
*
*/
public QSPTSDATETIMEFULL createQSPTSDATETIMEFULL() {
return new QSPTSDATETIMEFULL();
}
/**
* Create an instance of {@link HISTTSDATE }
*
*/
public HISTTSDATE createHISTTSDATE() {
return new HISTTSDATE();
}
/**
* Create an instance of {@link UVPCS }
*
*/
public UVPCS createUVPCS() {
return new UVPCS();
}
/**
* Create an instance of {@link StrucDocTRow }
*
*/
public StrucDocTRow createStrucDocTRow() {
return new StrucDocTRow();
}
/**
* Create an instance of {@link BAGPQ }
*
*/
public BAGPQ createBAGPQ() {
return new BAGPQ();
}
/**
* Create an instance of {@link SLISTTSDATETIME }
*
*/
public SLISTTSDATETIME createSLISTTSDATETIME() {
return new SLISTTSDATETIME();
}
/**
* Create an instance of {@link IVLPQ }
*
*/
public IVLPQ createIVLPQ() {
return new IVLPQ();
}
/**
* Create an instance of {@link QSPINTNONNEG }
*
*/
public QSPINTNONNEG createQSPINTNONNEG() {
return new QSPINTNONNEG();
}
/**
* Create an instance of {@link URGHIGHINTPOS }
*
*/
public URGHIGHINTPOS createURGHIGHINTPOS() {
return new URGHIGHINTPOS();
}
/**
* Create an instance of {@link IVLHIGHTSBIRTH }
*
*/
public IVLHIGHTSBIRTH createIVLHIGHTSBIRTH() {
return new IVLHIGHTSBIRTH();
}
/**
* Create an instance of {@link UVPENTN }
*
*/
public UVPENTN createUVPENTN() {
return new UVPENTN();
}
/**
* Create an instance of {@link DSETENTN }
*
*/
public DSETENTN createDSETENTN() {
return new DSETENTN();
}
/**
* Create an instance of {@link URGHIGHTSDATE }
*
*/
public URGHIGHTSDATE createURGHIGHTSDATE() {
return new URGHIGHTSDATE();
}
/**
* Create an instance of {@link IVLHIGHPQTIME }
*
*/
public IVLHIGHPQTIME createIVLHIGHPQTIME() {
return new IVLHIGHPQTIME();
}
/**
* Create an instance of {@link REAL }
*
*/
public REAL createREAL() {
return new REAL();
}
/**
* Create an instance of {@link UVPTELPERSON }
*
*/
public UVPTELPERSON createUVPTELPERSON() {
return new UVPTELPERSON();
}
/**
* Create an instance of {@link QSITSDATE }
*
*/
public QSITSDATE createQSITSDATE() {
return new QSITSDATE();
}
/**
* Create an instance of {@link LISTMO }
*
*/
public LISTMO createLISTMO() {
return new LISTMO();
}
/**
* Create an instance of {@link QSDINT }
*
*/
public QSDINT createQSDINT() {
return new QSDINT();
}
/**
* Create an instance of {@link GLISTMO }
*
*/
public GLISTMO createGLISTMO() {
return new GLISTMO();
}
/**
* Create an instance of {@link DSETSC }
*
*/
public DSETSC createDSETSC() {
return new DSETSC();
}
/**
* Create an instance of {@link TELPERSON }
*
*/
public TELPERSON createTELPERSON() {
return new TELPERSON();
}
/**
* Create an instance of {@link UVPCD }
*
*/
public UVPCD createUVPCD() {
return new UVPCD();
}
/**
* Create an instance of {@link DSETRTO }
*
*/
public DSETRTO createDSETRTO() {
return new DSETRTO();
}
/**
* Create an instance of {@link UVPCO }
*
*/
public UVPCO createUVPCO() {
return new UVPCO();
}
/**
* Create an instance of {@link URGCO }
*
*/
public URGCO createURGCO() {
return new URGCO();
}
/**
* Create an instance of {@link QSDRTO }
*
*/
public QSDRTO createQSDRTO() {
return new QSDRTO();
}
/**
* Create an instance of {@link QSDTSDATETIMEFULL }
*
*/
public QSDTSDATETIMEFULL createQSDTSDATETIMEFULL() {
return new QSDTSDATETIMEFULL();
}
/**
* Create an instance of {@link GLISTPQTIME }
*
*/
public GLISTPQTIME createGLISTPQTIME() {
return new GLISTPQTIME();
}
/**
* Create an instance of {@link IVLTSDATETIME }
*
*/
public IVLTSDATETIME createIVLTSDATETIME() {
return new IVLTSDATETIME();
}
/**
* Create an instance of {@link ENXP }
*
*/
public ENXP createENXP() {
return new ENXP();
}
/**
* Create an instance of {@link IVLREAL }
*
*/
public IVLREAL createIVLREAL() {
return new IVLREAL();
}
/**
* Create an instance of {@link URGHIGHPQ }
*
*/
public URGHIGHPQ createURGHIGHPQ() {
return new URGHIGHPQ();
}
/**
* Create an instance of {@link NPPDTS }
*
*/
public NPPDTS createNPPDTS() {
return new NPPDTS();
}
/**
* Create an instance of {@link NPPDAD }
*
*/
public NPPDAD createNPPDAD() {
return new NPPDAD();
}
/**
* Create an instance of {@link HISTMO }
*
*/
public HISTMO createHISTMO() {
return new HISTMO();
}
/**
* Create an instance of {@link NPPDTSBIRTH }
*
*/
public NPPDTSBIRTH createNPPDTSBIRTH() {
return new NPPDTSBIRTH();
}
/**
* Create an instance of {@link SLISTINT }
*
*/
public SLISTINT createSLISTINT() {
return new SLISTINT();
}
/**
* Create an instance of {@link ADXPAL }
*
*/
public ADXPAL createADXPAL() {
return new ADXPAL();
}
/**
* Create an instance of {@link LISTTELPHONE }
*
*/
public LISTTELPHONE createLISTTELPHONE() {
return new LISTTELPHONE();
}
/**
* Create an instance of {@link AUDITINFO }
*
*/
public AUDITINFO createAUDITINFO() {
return new AUDITINFO();
}
/**
* Create an instance of {@link DSETINTNONNEG }
*
*/
public DSETINTNONNEG createDSETINTNONNEG() {
return new DSETINTNONNEG();
}
/**
* Create an instance of {@link HISTINTNONNEG }
*
*/
public HISTINTNONNEG createHISTINTNONNEG() {
return new HISTINTNONNEG();
}
/**
* Create an instance of {@link LISTEDDOCREF }
*
*/
public LISTEDDOCREF createLISTEDDOCREF() {
return new LISTEDDOCREF();
}
/**
* Create an instance of {@link IVLLOWINTNONNEG }
*
*/
public IVLLOWINTNONNEG createIVLLOWINTNONNEG() {
return new IVLLOWINTNONNEG();
}
/**
* Create an instance of {@link GLISTINTNONNEG }
*
*/
public GLISTINTNONNEG createGLISTINTNONNEG() {
return new GLISTINTNONNEG();
}
/**
* Create an instance of {@link URGHIGHREAL }
*
*/
public URGHIGHREAL createURGHIGHREAL() {
return new URGHIGHREAL();
}
/**
* Create an instance of {@link ADXPDMOD }
*
*/
public ADXPDMOD createADXPDMOD() {
return new ADXPDMOD();
}
/**
* Create an instance of {@link NPPDST }
*
*/
public NPPDST createNPPDST() {
return new NPPDST();
}
/**
* Create an instance of {@link LISTTELPERSON }
*
*/
public LISTTELPERSON createLISTTELPERSON() {
return new LISTTELPERSON();
}
/**
* Create an instance of {@link IVLHIGHTS }
*
*/
public IVLHIGHTS createIVLHIGHTS() {
return new IVLHIGHTS();
}
/**
* Create an instance of {@link NPPDINTNONNEG }
*
*/
public NPPDINTNONNEG createNPPDINTNONNEG() {
return new NPPDINTNONNEG();
}
/**
* Create an instance of {@link EIVLTSDATETIME }
*
*/
public EIVLTSDATETIME createEIVLTSDATETIME() {
return new EIVLTSDATETIME();
}
/**
* Create an instance of {@link DSETPQ }
*
*/
public DSETPQ createDSETPQ() {
return new DSETPQ();
}
/**
* Create an instance of {@link QSSINTPOS }
*
*/
public QSSINTPOS createQSSINTPOS() {
return new QSSINTPOS();
}
/**
* Create an instance of {@link StrucDocCaption }
*
*/
public StrucDocCaption createStrucDocCaption() {
return new StrucDocCaption();
}
/**
* Create an instance of {@link QSIPQ }
*
*/
public QSIPQ createQSIPQ() {
return new QSIPQ();
}
/**
* Create an instance of {@link PQ }
*
*/
public PQ createPQ() {
return new PQ();
}
/**
* Create an instance of {@link UVPCDCV }
*
*/
public UVPCDCV createUVPCDCV() {
return new UVPCDCV();
}
/**
* Create an instance of {@link NPPDBL }
*
*/
public NPPDBL createNPPDBL() {
return new NPPDBL();
}
/**
* Create an instance of {@link QSPCO }
*
*/
public QSPCO createQSPCO() {
return new QSPCO();
}
/**
* Create an instance of {@link BAGMO }
*
*/
public BAGMO createBAGMO() {
return new BAGMO();
}
/**
* Create an instance of {@link StrucDocItem }
*
*/
public StrucDocItem createStrucDocItem() {
return new StrucDocItem();
}
/**
* Create an instance of {@link DSETSCNT }
*
*/
public DSETSCNT createDSETSCNT() {
return new DSETSCNT();
}
/**
* Create an instance of {@link GLISTTSDATE }
*
*/
public GLISTTSDATE createGLISTTSDATE() {
return new GLISTTSDATE();
}
/**
* Create an instance of {@link DSETMO }
*
*/
public DSETMO createDSETMO() {
return new DSETMO();
}
/**
* Create an instance of {@link SUBJECTOFCAREPERSONIDENTIFICATION }
*
*/
public SUBJECTOFCAREPERSONIDENTIFICATION createSUBJECTOFCAREPERSONIDENTIFICATION() {
return new SUBJECTOFCAREPERSONIDENTIFICATION();
}
/**
* Create an instance of {@link TS }
*
*/
public TS createTS() {
return new TS();
}
/**
* Create an instance of {@link ADXPSTR }
*
*/
public ADXPSTR createADXPSTR() {
return new ADXPSTR();
}
/**
* Create an instance of {@link DSETENON }
*
*/
public DSETENON createDSETENON() {
return new DSETENON();
}
/**
* Create an instance of {@link URGLOWTSDATETIMEFULL }
*
*/
public URGLOWTSDATETIMEFULL createURGLOWTSDATETIMEFULL() {
return new URGLOWTSDATETIMEFULL();
}
/**
* Create an instance of {@link DSETBLNONNULL }
*
*/
public DSETBLNONNULL createDSETBLNONNULL() {
return new DSETBLNONNULL();
}
/**
* Create an instance of {@link INTNONNEG }
*
*/
public INTNONNEG createINTNONNEG() {
return new INTNONNEG();
}
/**
* Create an instance of {@link ST }
*
*/
public ST createST() {
return new ST();
}
/**
* Create an instance of {@link STNT }
*
*/
public STNT createSTNT() {
return new STNT();
}
/**
* Create an instance of {@link ADXPBR }
*
*/
public ADXPBR createADXPBR() {
return new ADXPBR();
}
/**
* Create an instance of {@link NPPDTSDATEFULL }
*
*/
public NPPDTSDATEFULL createNPPDTSDATEFULL() {
return new NPPDTSDATEFULL();
}
/**
* Create an instance of {@link NPPDEDSTRUCTUREDTEXT }
*
*/
public NPPDEDSTRUCTUREDTEXT createNPPDEDSTRUCTUREDTEXT() {
return new NPPDEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link PIVLTSDATETIME }
*
*/
public PIVLTSDATETIME createPIVLTSDATETIME() {
return new PIVLTSDATETIME();
}
/**
* Create an instance of {@link URGLOWPQTIME }
*
*/
public URGLOWPQTIME createURGLOWPQTIME() {
return new URGLOWPQTIME();
}
/**
* Create an instance of {@link IVLRTO }
*
*/
public IVLRTO createIVLRTO() {
return new IVLRTO();
}
/**
* Create an instance of {@link URGLOWINT }
*
*/
public URGLOWINT createURGLOWINT() {
return new URGLOWINT();
}
/**
* Create an instance of {@link ADXPSTB }
*
*/
public ADXPSTB createADXPSTB() {
return new ADXPSTB();
}
/**
* Create an instance of {@link ADXPSTA }
*
*/
public ADXPSTA createADXPSTA() {
return new ADXPSTA();
}
/**
* Create an instance of {@link UVPSCNT }
*
*/
public UVPSCNT createUVPSCNT() {
return new UVPSCNT();
}
/**
* Create an instance of {@link SC }
*
*/
public SC createSC() {
return new SC();
}
/**
* Create an instance of {@link QSSPQTIME }
*
*/
public QSSPQTIME createQSSPQTIME() {
return new QSSPQTIME();
}
/**
* Create an instance of {@link LISTEDSTRUCTUREDTITLE }
*
*/
public LISTEDSTRUCTUREDTITLE createLISTEDSTRUCTUREDTITLE() {
return new LISTEDSTRUCTUREDTITLE();
}
/**
* Create an instance of {@link DSETEDSIGNATURE }
*
*/
public DSETEDSIGNATURE createDSETEDSIGNATURE() {
return new DSETEDSIGNATURE();
}
/**
* Create an instance of {@link IVLTSBIRTH }
*
*/
public IVLTSBIRTH createIVLTSBIRTH() {
return new IVLTSBIRTH();
}
/**
* Create an instance of {@link LISTII }
*
*/
public LISTII createLISTII() {
return new LISTII();
}
/**
* Create an instance of {@link HISTEDSTRUCTUREDTEXT }
*
*/
public HISTEDSTRUCTUREDTEXT createHISTEDSTRUCTUREDTEXT() {
return new HISTEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link QSDTSDATE }
*
*/
public QSDTSDATE createQSDTSDATE() {
return new QSDTSDATE();
}
/**
* Create an instance of {@link EDSTRUCTUREDTEXT }
*
*/
public EDSTRUCTUREDTEXT createEDSTRUCTUREDTEXT() {
return new EDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link LISTINTPOS }
*
*/
public LISTINTPOS createLISTINTPOS() {
return new LISTINTPOS();
}
/**
* Create an instance of {@link QSPTSDATE }
*
*/
public QSPTSDATE createQSPTSDATE() {
return new QSPTSDATE();
}
/**
* Create an instance of {@link DSETENPN }
*
*/
public DSETENPN createDSETENPN() {
return new DSETENPN();
}
/**
* Create an instance of {@link QSPTSBIRTH }
*
*/
public QSPTSBIRTH createQSPTSBIRTH() {
return new QSPTSBIRTH();
}
/**
* Create an instance of {@link GLISTREAL }
*
*/
public GLISTREAL createGLISTREAL() {
return new GLISTREAL();
}
/**
* Create an instance of {@link SLISTCO }
*
*/
public SLISTCO createSLISTCO() {
return new SLISTCO();
}
/**
* Create an instance of {@link IVLWIDTHTSDATE }
*
*/
public IVLWIDTHTSDATE createIVLWIDTHTSDATE() {
return new IVLWIDTHTSDATE();
}
/**
* Create an instance of {@link LISTBLNONNULL }
*
*/
public LISTBLNONNULL createLISTBLNONNULL() {
return new LISTBLNONNULL();
}
/**
* Create an instance of {@link IVLMO }
*
*/
public IVLMO createIVLMO() {
return new IVLMO();
}
/**
* Create an instance of {@link BAGTEL }
*
*/
public BAGTEL createBAGTEL() {
return new BAGTEL();
}
/**
* Create an instance of {@link URGHIGHPQTIME }
*
*/
public URGHIGHPQTIME createURGHIGHPQTIME() {
return new URGHIGHPQTIME();
}
/**
* Create an instance of {@link DSETTSDATE }
*
*/
public DSETTSDATE createDSETTSDATE() {
return new DSETTSDATE();
}
/**
* Create an instance of {@link ORGANISATION }
*
*/
public ORGANISATION createORGANISATION() {
return new ORGANISATION();
}
/**
* Create an instance of {@link ADXPCAR }
*
*/
public ADXPCAR createADXPCAR() {
return new ADXPCAR();
}
/**
* Create an instance of {@link StrucDocRenderMultiMedia }
*
*/
public StrucDocRenderMultiMedia createStrucDocRenderMultiMedia() {
return new StrucDocRenderMultiMedia();
}
/**
* Create an instance of {@link IVLLOWINTPOS }
*
*/
public IVLLOWINTPOS createIVLLOWINTPOS() {
return new IVLLOWINTPOS();
}
/**
* Create an instance of {@link IVLHIGHCO }
*
*/
public IVLHIGHCO createIVLHIGHCO() {
return new IVLHIGHCO();
}
/**
* Create an instance of {@link IVLWIDTHMO }
*
*/
public IVLWIDTHMO createIVLWIDTHMO() {
return new IVLWIDTHMO();
}
/**
* Create an instance of {@link NPPDEDDOC }
*
*/
public NPPDEDDOC createNPPDEDDOC() {
return new NPPDEDDOC();
}
/**
* Create an instance of {@link LISTSC }
*
*/
public LISTSC createLISTSC() {
return new LISTSC();
}
/**
* Create an instance of {@link IVLWIDTHTSDATEFULL }
*
*/
public IVLWIDTHTSDATEFULL createIVLWIDTHTSDATEFULL() {
return new IVLWIDTHTSDATEFULL();
}
/**
* Create an instance of {@link HISTTSDATEFULL }
*
*/
public HISTTSDATEFULL createHISTTSDATEFULL() {
return new HISTTSDATEFULL();
}
/**
* Create an instance of {@link EXTRACTCRITERIA }
*
*/
public EXTRACTCRITERIA createEXTRACTCRITERIA() {
return new EXTRACTCRITERIA();
}
/**
* Create an instance of {@link RELATEDPARTY }
*
*/
public RELATEDPARTY createRELATEDPARTY() {
return new RELATEDPARTY();
}
/**
* Create an instance of {@link QSSCO }
*
*/
public QSSCO createQSSCO() {
return new QSSCO();
}
/**
* Create an instance of {@link QSITS }
*
*/
public QSITS createQSITS() {
return new QSITS();
}
/**
* Create an instance of {@link UVPII }
*
*/
public UVPII createUVPII() {
return new UVPII();
}
/**
* Create an instance of {@link TELEMAIL }
*
*/
public TELEMAIL createTELEMAIL() {
return new TELEMAIL();
}
/**
* Create an instance of {@link DSETTELURL }
*
*/
public DSETTELURL createDSETTELURL() {
return new DSETTELURL();
}
/**
* Create an instance of {@link IVLWIDTHREAL }
*
*/
public IVLWIDTHREAL createIVLWIDTHREAL() {
return new IVLWIDTHREAL();
}
/**
* Create an instance of {@link UVPTEL }
*
*/
public UVPTEL createUVPTEL() {
return new UVPTEL();
}
/**
* Create an instance of {@link BAGII }
*
*/
public BAGII createBAGII() {
return new BAGII();
}
/**
* Create an instance of {@link HISTAD }
*
*/
public HISTAD createHISTAD() {
return new HISTAD();
}
/**
* Create an instance of {@link LISTENPN }
*
*/
public LISTENPN createLISTENPN() {
return new LISTENPN();
}
/**
* Create an instance of {@link HISTTEL }
*
*/
public HISTTEL createHISTTEL() {
return new HISTTEL();
}
/**
* Create an instance of {@link BAGEDSTRUCTUREDTEXT }
*
*/
public BAGEDSTRUCTUREDTEXT createBAGEDSTRUCTUREDTEXT() {
return new BAGEDSTRUCTUREDTEXT();
}
/**
* Create an instance of {@link LISTTSDATEFULL }
*
*/
public LISTTSDATEFULL createLISTTSDATEFULL() {
return new LISTTSDATEFULL();
}
/**
* Create an instance of {@link LISTRTO }
*
*/
public LISTRTO createLISTRTO() {
return new LISTRTO();
}
/**
* Create an instance of {@link IVLLOWTSDATETIME }
*
*/
public IVLLOWTSDATETIME createIVLLOWTSDATETIME() {
return new IVLLOWTSDATETIME();
}
/**
* Create an instance of {@link LISTST }
*
*/
public LISTST createLISTST() {
return new LISTST();
}
/**
* Create an instance of {@link EDDOC }
*
*/
public EDDOC createEDDOC() {
return new EDDOC();
}
/**
* Create an instance of {@link LISTREAL }
*
*/
public LISTREAL createLISTREAL() {
return new LISTREAL();
}
/**
* Create an instance of {@link URGHIGHMO }
*
*/
public URGHIGHMO createURGHIGHMO() {
return new URGHIGHMO();
}
/**
* Create an instance of {@link HISTTELEMAIL }
*
*/
public HISTTELEMAIL createHISTTELEMAIL() {
return new HISTTELEMAIL();
}
/**
* Create an instance of {@link BAGTSBIRTH }
*
*/
public BAGTSBIRTH createBAGTSBIRTH() {
return new BAGTSBIRTH();
}
/**
* Create an instance of {@link StrucDocSub }
*
*/
public StrucDocSub createStrucDocSub() {
return new StrucDocSub();
}
/**
* Create an instance of {@link QSDINTNONNEG }
*
*/
public QSDINTNONNEG createQSDINTNONNEG() {
return new QSDINTNONNEG();
}
/**
* Create an instance of {@link TSDATE }
*
*/
public TSDATE createTSDATE() {
return new TSDATE();
}
/**
* Create an instance of {@link EIVLTSDATE }
*
*/
public EIVLTSDATE createEIVLTSDATE() {
return new EIVLTSDATE();
}
/**
* Create an instance of {@link LISTINT }
*
*/
public LISTINT createLISTINT() {
return new LISTINT();
}
/**
* Create an instance of {@link IDENTIFIEDHEALTHCAREPROFESSIONAL }
*
*/
public IDENTIFIEDHEALTHCAREPROFESSIONAL createIDENTIFIEDHEALTHCAREPROFESSIONAL() {
return new IDENTIFIEDHEALTHCAREPROFESSIONAL();
}
/**
* Create an instance of {@link URGTSDATETIMEFULL }
*
*/
public URGTSDATETIMEFULL createURGTSDATETIMEFULL() {
return new URGTSDATETIMEFULL();
}
/**
* Create an instance of {@link EDSIGNATURE }
*
*/
public EDSIGNATURE createEDSIGNATURE() {
return new EDSIGNATURE();
}
/**
* Create an instance of {@link StrucDocSup }
*
*/
public StrucDocSup createStrucDocSup() {
return new StrucDocSup();
}
/**
* Create an instance of {@link SLISTTS }
*
*/
public SLISTTS createSLISTTS() {
return new SLISTTS();
}
/**
* Create an instance of {@link DSETEDIMAGE }
*
*/
public DSETEDIMAGE createDSETEDIMAGE() {
return new DSETEDIMAGE();
}
/**
* Create an instance of {@link SLISTRTO }
*
*/
public SLISTRTO createSLISTRTO() {
return new SLISTRTO();
}
/**
* Create an instance of {@link LISTSTNT }
*
*/
public LISTSTNT createLISTSTNT() {
return new LISTSTNT();
}
/**
* Create an instance of {@link LISTENTN }
*
*/
public LISTENTN createLISTENTN() {
return new LISTENTN();
}
/**
* Create an instance of {@link BAGSTNT }
*
*/
public BAGSTNT createBAGSTNT() {
return new BAGSTNT();
}
/**
* Create an instance of {@link GLISTTS }
*
*/
public GLISTTS createGLISTTS() {
return new GLISTTS();
}
/**
* Create an instance of {@link BAGBLNONNULL }
*
*/
public BAGBLNONNULL createBAGBLNONNULL() {
return new BAGBLNONNULL();
}
/**
* Create an instance of {@link NPPDBLNONNULL }
*
*/
public NPPDBLNONNULL createNPPDBLNONNULL() {
return new NPPDBLNONNULL();
}
/**
* Create an instance of {@link LISTTS }
*
*/
public LISTTS createLISTTS() {
return new LISTTS();
}
/**
* Create an instance of {@link GLISTTSDATETIMEFULL }
*
*/
public GLISTTSDATETIMEFULL createGLISTTSDATETIMEFULL() {
return new GLISTTSDATETIMEFULL();
}
/**
* Create an instance of {@link GLISTTSBIRTH }
*
*/
public GLISTTSBIRTH createGLISTTSBIRTH() {
return new GLISTTSBIRTH();
}
/**
* Create an instance of {@link PQR }
*
*/
public PQR createPQR() {
return new PQR();
}
/**
* Create an instance of {@link PQV }
*
*/
public PQV createPQV() {
return new PQV();
}
/**
* Create an instance of {@link StrucDocTCell }
*
*/
public StrucDocTCell createStrucDocTCell() {
return new StrucDocTCell();
}
/**
* Create an instance of {@link BAGEDDOC }
*
*/
public BAGEDDOC createBAGEDDOC() {
return new BAGEDDOC();
}
/**
* Create an instance of {@link ADXPZIP }
*
*/
public ADXPZIP createADXPZIP() {
return new ADXPZIP();
}
/**
* Create an instance of {@link DSETTELEMAIL }
*
*/
public DSETTELEMAIL createDSETTELEMAIL() {
return new DSETTELEMAIL();
}
/**
* Create an instance of {@link NPPDENTN }
*
*/
public NPPDENTN createNPPDENTN() {
return new NPPDENTN();
}
/**
* Create an instance of {@link ADXPDIR }
*
*/
public ADXPDIR createADXPDIR() {
return new ADXPDIR();
}
/**
* Create an instance of {@link COMPOSITION }
*
*/
public COMPOSITION createCOMPOSITION() {
return new COMPOSITION();
}
/**
* Create an instance of {@link UVPTSDATE }
*
*/
public UVPTSDATE createUVPTSDATE() {
return new UVPTSDATE();
}
/**
* Create an instance of {@link QSSINT }
*
*/
public QSSINT createQSSINT() {
return new QSSINT();
}
/**
* Create an instance of {@link IVLHIGHINT }
*
*/
public IVLHIGHINT createIVLHIGHINT() {
return new IVLHIGHINT();
}
/**
* Create an instance of {@link UVPTSDATETIMEFULL }
*
*/
public UVPTSDATETIMEFULL createUVPTSDATETIMEFULL() {
return new UVPTSDATETIMEFULL();
}
/**
* Create an instance of {@link DSETTSBIRTH }
*
*/
public DSETTSBIRTH createDSETTSBIRTH() {
return new DSETTSBIRTH();
}
/**
* Create an instance of {@link IVLLOWREAL }
*
*/
public IVLLOWREAL createIVLLOWREAL() {
return new IVLLOWREAL();
}
/**
* Create an instance of {@link QSSTSBIRTH }
*
*/
public QSSTSBIRTH createQSSTSBIRTH() {
return new QSSTSBIRTH();
}
/**
* Create an instance of {@link StrucDocLinkHtml }
*
*/
public StrucDocLinkHtml createStrucDocLinkHtml() {
return new StrucDocLinkHtml();
}
/**
* Create an instance of {@link BAGTELPHONE }
*
*/
public BAGTELPHONE createBAGTELPHONE() {
return new BAGTELPHONE();
}
/**
* Create an instance of {@link BAGSCNT }
*
*/
public BAGSCNT createBAGSCNT() {
return new BAGSCNT();
}
/**
* Create an instance of {@link LISTEDDOCINLINE }
*
*/
public LISTEDDOCINLINE createLISTEDDOCINLINE() {
return new LISTEDDOCINLINE();
}
/**
* Create an instance of {@link DSETTEL }
*
*/
public DSETTEL createDSETTEL() {
return new DSETTEL();
}
/**
* Create an instance of {@link TSDATEFULL }
*
*/
public TSDATEFULL createTSDATEFULL() {
return new TSDATEFULL();
}
/**
* Create an instance of {@link IVLLOWCO }
*
*/
public IVLLOWCO createIVLLOWCO() {
return new IVLLOWCO();
}
/**
* Create an instance of {@link IVLLOWTSDATETIMEFULL }
*
*/
public IVLLOWTSDATETIMEFULL createIVLLOWTSDATETIMEFULL() {
return new IVLLOWTSDATETIMEFULL();
}
/**
* Create an instance of {@link NPPDEDSIGNATURE }
*
*/
public NPPDEDSIGNATURE createNPPDEDSIGNATURE() {
return new NPPDEDSIGNATURE();
}
/**
* Create an instance of {@link QSPINTPOS }
*
*/
public QSPINTPOS createQSPINTPOS() {
return new QSPINTPOS();
}
/**
* Create an instance of {@link SLISTTSBIRTH }
*
*/
public SLISTTSBIRTH createSLISTTSBIRTH() {
return new SLISTTSBIRTH();
}
/**
* Create an instance of {@link DSETREAL }
*
*/
public DSETREAL createDSETREAL() {
return new DSETREAL();
}
/**
* Create an instance of {@link BAGTSDATE }
*
*/
public BAGTSDATE createBAGTSDATE() {
return new BAGTSDATE();
}
/**
* Create an instance of {@link HISTTELURL }
*
*/
public HISTTELURL createHISTTELURL() {
return new HISTTELURL();
}
/**
* Create an instance of {@link BAGEN }
*
*/
public BAGEN createBAGEN() {
return new BAGEN();
}
/**
* Create an instance of {@link BLNONNULL }
*
*/
public BLNONNULL createBLNONNULL() {
return new BLNONNULL();
}
/**
* Create an instance of {@link EHREXTRACT }
*
*/
public EHREXTRACT createEHREXTRACT() {
return new EHREXTRACT();
}
/**
* Create an instance of {@link HISTED }
*
*/
public HISTED createHISTED() {
return new HISTED();
}
/**
* Create an instance of {@link LISTTSDATE }
*
*/
public LISTTSDATE createLISTTSDATE() {
return new LISTTSDATE();
}
/**
* Create an instance of {@link HISTINTPOS }
*
*/
public HISTINTPOS createHISTINTPOS() {
return new HISTINTPOS();
}
/**
* Create an instance of {@link UVPEN }
*
*/
public UVPEN createUVPEN() {
return new UVPEN();
}
/**
* Create an instance of {@link HISTEN }
*
*/
public HISTEN createHISTEN() {
return new HISTEN();
}
/**
* Create an instance of {@link PIVLTSDATEFULL }
*
*/
public PIVLTSDATEFULL createPIVLTSDATEFULL() {
return new PIVLTSDATEFULL();
}
/**
* Create an instance of {@link BAGED }
*
*/
public BAGED createBAGED() {
return new BAGED();
}
/**
* Create an instance of {@link IVLLOWPQTIME }
*
*/
public IVLLOWPQTIME createIVLLOWPQTIME() {
return new IVLLOWPQTIME();
}
/**
* Create an instance of {@link QSSTSDATETIMEFULL }
*
*/
public QSSTSDATETIMEFULL createQSSTSDATETIMEFULL() {
return new QSSTSDATETIMEFULL();
}
/**
* Create an instance of {@link IVLWIDTHPQ }
*
*/
public IVLWIDTHPQ createIVLWIDTHPQ() {
return new IVLWIDTHPQ();
}
/**
* Create an instance of {@link HISTTSDATETIMEFULL }
*
*/
public HISTTSDATETIMEFULL createHISTTSDATETIMEFULL() {
return new HISTTSDATETIMEFULL();
}
/**
* Create an instance of {@link QSETBOUNDEDPIVL }
*
*/
public QSETBOUNDEDPIVL createQSETBOUNDEDPIVL() {
return new QSETBOUNDEDPIVL();
}
/**
* Create an instance of {@link UVPED }
*
*/
public UVPED createUVPED() {
return new UVPED();
}
/**
* Create an instance of {@link DSETEDDOC }
*
*/
public DSETEDDOC createDSETEDDOC() {
return new DSETEDDOC();
}
/**
* Create an instance of {@link HISTCDCV }
*
*/
public HISTCDCV createHISTCDCV() {
return new HISTCDCV();
}
/**
* Create an instance of {@link UVPEDSIGNATURE }
*
*/
public UVPEDSIGNATURE createUVPEDSIGNATURE() {
return new UVPEDSIGNATURE();
}
/**
* Create an instance of {@link BAGREAL }
*
*/
public BAGREAL createBAGREAL() {
return new BAGREAL();
}
/**
* Create an instance of {@link HISTBLNONNULL }
*
*/
public HISTBLNONNULL createHISTBLNONNULL() {
return new HISTBLNONNULL();
}
/**
* Create an instance of {@link LISTENON }
*
*/
public LISTENON createLISTENON() {
return new LISTENON();
}
/**
* Create an instance of {@link HISTBL }
*
*/
public HISTBL createHISTBL() {
return new HISTBL();
}
/**
* Create an instance of {@link QSPTSDATETIME }
*
*/
public QSPTSDATETIME createQSPTSDATETIME() {
return new QSPTSDATETIME();
}
/**
* Create an instance of {@link QSPRTO }
*
*/
public QSPRTO createQSPRTO() {
return new QSPRTO();
}
/**
* Create an instance of {@link RTO }
*
*/
public RTO createRTO() {
return new RTO();
}
/**
* Create an instance of {@link IVLWIDTHPQTIME }
*
*/
public IVLWIDTHPQTIME createIVLWIDTHPQTIME() {
return new IVLWIDTHPQTIME();
}
/**
* Create an instance of {@link BAGEDIMAGE }
*
*/
public BAGEDIMAGE createBAGEDIMAGE() {
return new BAGEDIMAGE();
}
/**
* Create an instance of {@link UVPENPN }
*
*/
public UVPENPN createUVPENPN() {
return new UVPENPN();
}
/**
* Create an instance of {@link BAGCO }
*
*/
public BAGCO createBAGCO() {
return new BAGCO();
}
/**
* Create an instance of {@link GLISTPQ }
*
*/
public GLISTPQ createGLISTPQ() {
return new GLISTPQ();
}
/**
* Create an instance of {@link INTPOS }
*
*/
public INTPOS createINTPOS() {
return new INTPOS();
}
/**
* Create an instance of {@link LISTEDDOC }
*
*/
public LISTEDDOC createLISTEDDOC() {
return new LISTEDDOC();
}
/**
* Create an instance of {@link LISTPQ }
*
*/
public LISTPQ createLISTPQ() {
return new LISTPQ();
}
/**
* Create an instance of {@link ENTRY }
*
*/
public ENTRY createENTRY() {
return new ENTRY();
}
/**
* Create an instance of {@link BAGCS }
*
*/
public BAGCS createBAGCS() {
return new BAGCS();
}
/**
* Create an instance of {@link UVPINTNONNEG }
*
*/
public UVPINTNONNEG createUVPINTNONNEG() {
return new UVPINTNONNEG();
}
/**
* Create an instance of {@link NPPDTELURL }
*
*/
public NPPDTELURL createNPPDTELURL() {
return new NPPDTELURL();
}
/**
* Create an instance of {@link URGHIGHTSDATETIMEFULL }
*
*/
public URGHIGHTSDATETIMEFULL createURGHIGHTSDATETIMEFULL() {
return new URGHIGHTSDATETIMEFULL();
}
/**
* Create an instance of {@link HISTCD }
*
*/
public HISTCD createHISTCD() {
return new HISTCD();
}
/**
* Create an instance of {@link StrucDocText }
*
*/
public StrucDocText createStrucDocText() {
return new StrucDocText();
}
/**
* Create an instance of {@link FUNCTIONALROLE }
*
*/
public FUNCTIONALROLE createFUNCTIONALROLE() {
return new FUNCTIONALROLE();
}
/**
* Create an instance of {@link IVLHIGHRTO }
*
*/
public IVLHIGHRTO createIVLHIGHRTO() {
return new IVLHIGHRTO();
}
/**
* Create an instance of {@link ANY }
*
*/
public ANY createANY() {
return new ANY();
}
/**
* Create an instance of {@link ADXP }
*
*/
public ADXP createADXP() {
return new ADXP();
}
/**
* Create an instance of {@link HISTCO }
*
*/
public HISTCO createHISTCO() {
return new HISTCO();
}
/**
* Create an instance of {@link BAGCD }
*
*/
public BAGCD createBAGCD() {
return new BAGCD();
}
/**
* Create an instance of {@link NPPDTSDATE }
*
*/
public NPPDTSDATE createNPPDTSDATE() {
return new NPPDTSDATE();
}
/**
* Create an instance of {@link BAGTSDATEFULL }
*
*/
public BAGTSDATEFULL createBAGTSDATEFULL() {
return new BAGTSDATEFULL();
}
/**
* Create an instance of {@link HISTCS }
*
*/
public HISTCS createHISTCS() {
return new HISTCS();
}
/**
* Create an instance of {@link EDTEXT }
*
*/
public EDTEXT createEDTEXT() {
return new EDTEXT();
}
/**
* Create an instance of {@link URGTSDATETIME }
*
*/
public URGTSDATETIME createURGTSDATETIME() {
return new URGTSDATETIME();
}
/**
* Create an instance of {@link LISTTSBIRTH }
*
*/
public LISTTSBIRTH createLISTTSBIRTH() {
return new LISTTSBIRTH();
}
/**
* Create an instance of {@link QSSTSDATETIME }
*
*/
public QSSTSDATETIME createQSSTSDATETIME() {
return new QSSTSDATETIME();
}
/**
* Create an instance of {@link BAGBL }
*
*/
public BAGBL createBAGBL() {
return new BAGBL();
}
/**
* Create an instance of {@link BAGEDDOCINLINE }
*
*/
public BAGEDDOCINLINE createBAGEDDOCINLINE() {
return new BAGEDDOCINLINE();
}
/**
* Create an instance of {@link NPPDENPN }
*
*/
public NPPDENPN createNPPDENPN() {
return new NPPDENPN();
}
/**
* Create an instance of {@link EIVLTSDATETIMEFULL }
*
*/
public EIVLTSDATETIMEFULL createEIVLTSDATETIMEFULL() {
return new EIVLTSDATETIMEFULL();
}
/**
* Create an instance of {@link EDDOCREF }
*
*/
public EDDOCREF createEDDOCREF() {
return new EDDOCREF();
}
/**
* Create an instance of {@link QSUTSBIRTH }
*
*/
public QSUTSBIRTH createQSUTSBIRTH() {
return new QSUTSBIRTH();
}
/**
* Create an instance of {@link BAGTELEMAIL }
*
*/
public BAGTELEMAIL createBAGTELEMAIL() {
return new BAGTELEMAIL();
}
/**
* Create an instance of {@link UVPENON }
*
*/
public UVPENON createUVPENON() {
return new UVPENON();
}
/**
* Create an instance of {@link LISTEDIMAGE }
*
*/
public LISTEDIMAGE createLISTEDIMAGE() {
return new LISTEDIMAGE();
}
/**
* Create an instance of {@link DSETCDCV }
*
*/
public DSETCDCV createDSETCDCV() {
return new DSETCDCV();
}
/**
* Create an instance of {@link DSETEDDOCINLINE }
*
*/
public DSETEDDOCINLINE createDSETEDDOCINLINE() {
return new DSETEDDOCINLINE();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocCMTitle }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleContent(StrucDocCMTitle value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocCMTitle.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTitleFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleFootnote(StrucDocTitleFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocTitleFootnote.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocTitle.class)
public JAXBElement createStrucDocTitleFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocList }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "list", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellList(StrucDocList value) {
return new JAXBElement(_StrucDocTCellList_QNAME, StrucDocList.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTable }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "table", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellTable(StrucDocTable value) {
return new JAXBElement(_StrucDocTCellTable_QNAME, StrucDocTable.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocRenderMultiMedia }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "renderMultiMedia", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellRenderMultiMedia(StrucDocRenderMultiMedia value) {
return new JAXBElement(_StrucDocTCellRenderMultiMedia_QNAME, StrucDocRenderMultiMedia.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocContent }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellContent(StrucDocContent value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocContent.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocParagraph }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "paragraph", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellParagraph(StrucDocParagraph value) {
return new JAXBElement(_StrucDocTCellParagraph_QNAME, StrucDocParagraph.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocTCell.class)
public JAXBElement createStrucDocTCellFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocTCell.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocList }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "list", scope = StrucDocText.class)
public JAXBElement createStrucDocTextList(StrucDocList value) {
return new JAXBElement(_StrucDocTCellList_QNAME, StrucDocList.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTable }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "table", scope = StrucDocText.class)
public JAXBElement createStrucDocTextTable(StrucDocTable value) {
return new JAXBElement(_StrucDocTCellTable_QNAME, StrucDocTable.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocText.class)
public JAXBElement createStrucDocTextSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocText.class)
public JAXBElement createStrucDocTextBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocText.class)
public JAXBElement createStrucDocTextLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocRenderMultiMedia }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "renderMultiMedia", scope = StrucDocText.class)
public JAXBElement createStrucDocTextRenderMultiMedia(StrucDocRenderMultiMedia value) {
return new JAXBElement(_StrucDocTCellRenderMultiMedia_QNAME, StrucDocRenderMultiMedia.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocContent }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocText.class)
public JAXBElement createStrucDocTextContent(StrucDocContent value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocContent.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocText.class)
public JAXBElement createStrucDocTextSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocParagraph }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "paragraph", scope = StrucDocText.class)
public JAXBElement createStrucDocTextParagraph(StrucDocParagraph value) {
return new JAXBElement(_StrucDocTCellParagraph_QNAME, StrucDocParagraph.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocText.class)
public JAXBElement createStrucDocTextFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocText.class)
public JAXBElement createStrucDocTextFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocText.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTCell }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "td", scope = StrucDocTRow.class)
public JAXBElement createStrucDocTRowTd(StrucDocTCell value) {
return new JAXBElement(_StrucDocTRowTd_QNAME, StrucDocTCell.class, StrucDocTRow.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTCell }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "th", scope = StrucDocTRow.class)
public JAXBElement createStrucDocTRowTh(StrucDocTCell value) {
return new JAXBElement(_StrucDocTRowTh_QNAME, StrucDocTCell.class, StrucDocTRow.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocCMTitle }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteContent(StrucDocCMTitle value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocCMTitle.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTitleFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteFootnote(StrucDocTitleFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocTitleFootnote.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocTitleFootnote.class)
public JAXBElement createStrucDocTitleFootnoteFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocTitleFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocList }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "list", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteList(StrucDocList value) {
return new JAXBElement(_StrucDocTCellList_QNAME, StrucDocList.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTable }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "table", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteTable(StrucDocTable value) {
return new JAXBElement(_StrucDocTCellTable_QNAME, StrucDocTable.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocRenderMultiMedia }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "renderMultiMedia", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteRenderMultiMedia(StrucDocRenderMultiMedia value) {
return new JAXBElement(_StrucDocTCellRenderMultiMedia_QNAME, StrucDocRenderMultiMedia.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocContent }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteContent(StrucDocContent value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocContent.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocParagraph }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "paragraph", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteParagraph(StrucDocParagraph value) {
return new JAXBElement(_StrucDocTCellParagraph_QNAME, StrucDocParagraph.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocFootnote.class)
public JAXBElement createStrucDocFootnoteFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocFootnote.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocCMTitle }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleContent(StrucDocCMTitle value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocCMTitle.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocTitleFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleFootnote(StrucDocTitleFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocTitleFootnote.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocCMTitle.class)
public JAXBElement createStrucDocCMTitleFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocCMTitle.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocBr }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "br", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentBr(StrucDocBr value) {
return new JAXBElement(_StrucDocTitleBr_QNAME, StrucDocBr.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocRenderMultiMedia }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "renderMultiMedia", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentRenderMultiMedia(StrucDocRenderMultiMedia value) {
return new JAXBElement(_StrucDocTCellRenderMultiMedia_QNAME, StrucDocRenderMultiMedia.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocContent }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "content", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentContent(StrucDocContent value) {
return new JAXBElement(_StrucDocTitleContent_QNAME, StrucDocContent.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocContent.class)
public JAXBElement createStrucDocContentFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocContent.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocLinkHtml.class)
public JAXBElement createStrucDocLinkHtmlFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocLinkHtml.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocLinkHtml.class)
public JAXBElement createStrucDocLinkHtmlFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocLinkHtml.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocCaption }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "caption", scope = StrucDocCaptioned.class)
public JAXBElement createStrucDocCaptionedCaption(StrucDocCaption value) {
return new JAXBElement(_StrucDocCaptionedCaption_QNAME, StrucDocCaption.class, StrucDocCaptioned.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSup }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sup", scope = StrucDocCaption.class)
public JAXBElement createStrucDocCaptionSup(StrucDocSup value) {
return new JAXBElement(_StrucDocTitleSup_QNAME, StrucDocSup.class, StrucDocCaption.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocLinkHtml }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "linkHtml", scope = StrucDocCaption.class)
public JAXBElement createStrucDocCaptionLinkHtml(StrucDocLinkHtml value) {
return new JAXBElement(_StrucDocTitleLinkHtml_QNAME, StrucDocLinkHtml.class, StrucDocCaption.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocSub }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "sub", scope = StrucDocCaption.class)
public JAXBElement createStrucDocCaptionSub(StrucDocSub value) {
return new JAXBElement(_StrucDocTitleSub_QNAME, StrucDocSub.class, StrucDocCaption.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnote }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnote", scope = StrucDocCaption.class)
public JAXBElement createStrucDocCaptionFootnote(StrucDocFootnote value) {
return new JAXBElement(_StrucDocTitleFootnote_QNAME, StrucDocFootnote.class, StrucDocCaption.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StrucDocFootnoteRef }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:riv:ehr:patientsummary:1", name = "footnoteRef", scope = StrucDocCaption.class)
public JAXBElement createStrucDocCaptionFootnoteRef(StrucDocFootnoteRef value) {
return new JAXBElement(_StrucDocTitleFootnoteRef_QNAME, StrucDocFootnoteRef.class, StrucDocCaption.class, value);
}
}