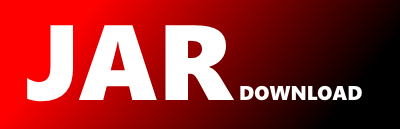
riv.ehr.patientsummary._1.RECORDCOMPONENT Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
/**
*
* Superclass of all concrete aggregator classes in the EHR
* hierarchy.
*
*
* Java class for RECORD_COMPONENT complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="RECORD_COMPONENT">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="archetype_id" type="{urn:riv:ehr:patientsummary:1}ST" minOccurs="0"/>
* <element name="meaning" type="{urn:riv:ehr:patientsummary:1}CD" minOccurs="0"/>
* <element name="name" type="{urn:riv:ehr:patientsummary:1}ST"/>
* <element name="orig_parent_ref" type="{urn:riv:ehr:patientsummary:1}II" minOccurs="0"/>
* <element name="policy_ids" type="{urn:riv:ehr:patientsummary:1}II" maxOccurs="unbounded" minOccurs="0"/>
* <element name="rc_id" type="{urn:riv:ehr:patientsummary:1}II"/>
* <element name="sensitivity" type="{urn:riv:ehr:patientsummary:1}INT" minOccurs="0"/>
* <element name="synthesised" type="{urn:riv:ehr:patientsummary:1}BL"/>
* <element name="links" type="{urn:riv:ehr:patientsummary:1}LINK" maxOccurs="unbounded" minOccurs="0"/>
* <element name="feeder_audit" type="{urn:riv:ehr:patientsummary:1}AUDIT_INFO" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RECORD_COMPONENT", propOrder = {
"archetypeId",
"meaning",
"name",
"origParentRef",
"policyIds",
"rcId",
"sensitivity",
"synthesised",
"links",
"feederAudit"
})
@XmlSeeAlso({
FOLDER.class,
ITEM.class,
COMPOSITION.class,
CONTENT.class
})
public abstract class RECORDCOMPONENT {
@XmlElement(name = "archetype_id")
protected ST archetypeId;
protected CD meaning;
@XmlElement(required = true)
protected ST name;
@XmlElement(name = "orig_parent_ref")
protected II origParentRef;
@XmlElement(name = "policy_ids")
protected List policyIds;
@XmlElement(name = "rc_id", required = true)
protected II rcId;
protected INT sensitivity;
@XmlElement(required = true)
protected BL synthesised;
protected List links;
@XmlElement(name = "feeder_audit")
protected AUDITINFO feederAudit;
/**
* Gets the value of the archetypeId property.
*
* @return
* possible object is
* {@link ST }
*
*/
public ST getArchetypeId() {
return archetypeId;
}
/**
* Sets the value of the archetypeId property.
*
* @param value
* allowed object is
* {@link ST }
*
*/
public void setArchetypeId(ST value) {
this.archetypeId = value;
}
/**
* Gets the value of the meaning property.
*
* @return
* possible object is
* {@link CD }
*
*/
public CD getMeaning() {
return meaning;
}
/**
* Sets the value of the meaning property.
*
* @param value
* allowed object is
* {@link CD }
*
*/
public void setMeaning(CD value) {
this.meaning = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link ST }
*
*/
public ST getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link ST }
*
*/
public void setName(ST value) {
this.name = value;
}
/**
* Gets the value of the origParentRef property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getOrigParentRef() {
return origParentRef;
}
/**
* Sets the value of the origParentRef property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setOrigParentRef(II value) {
this.origParentRef = value;
}
/**
* Gets the value of the policyIds property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the policyIds property.
*
*
* For example, to add a new item, do as follows:
*
* getPolicyIds().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link II }
*
*
*/
public List getPolicyIds() {
if (policyIds == null) {
policyIds = new ArrayList();
}
return this.policyIds;
}
/**
* Gets the value of the rcId property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getRcId() {
return rcId;
}
/**
* Sets the value of the rcId property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setRcId(II value) {
this.rcId = value;
}
/**
* Gets the value of the sensitivity property.
*
* @return
* possible object is
* {@link INT }
*
*/
public INT getSensitivity() {
return sensitivity;
}
/**
* Sets the value of the sensitivity property.
*
* @param value
* allowed object is
* {@link INT }
*
*/
public void setSensitivity(INT value) {
this.sensitivity = value;
}
/**
* Gets the value of the synthesised property.
*
* @return
* possible object is
* {@link BL }
*
*/
public BL getSynthesised() {
return synthesised;
}
/**
* Sets the value of the synthesised property.
*
* @param value
* allowed object is
* {@link BL }
*
*/
public void setSynthesised(BL value) {
this.synthesised = value;
}
/**
* Gets the value of the links property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the links property.
*
*
* For example, to add a new item, do as follows:
*
* getLinks().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LINK }
*
*
*/
public List getLinks() {
if (links == null) {
links = new ArrayList();
}
return this.links;
}
/**
* Gets the value of the feederAudit property.
*
* @return
* possible object is
* {@link AUDITINFO }
*
*/
public AUDITINFO getFeederAudit() {
return feederAudit;
}
/**
* Sets the value of the feederAudit property.
*
* @param value
* allowed object is
* {@link AUDITINFO }
*
*/
public void setFeederAudit(AUDITINFO value) {
this.feederAudit = value;
}
}