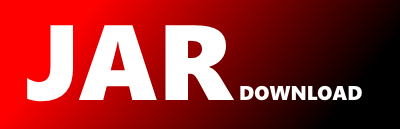
riv.ehr.patientsummary._1.StrucDocTable Maven / Gradle / Ivy
package riv.ehr.patientsummary._1;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for StrucDoc.Table complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="StrucDoc.Table">
* <complexContent>
* <extension base="{urn:riv:ehr:patientsummary:1}StrucDoc.Captioned">
* <sequence>
* <element name="col" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Col" maxOccurs="unbounded" minOccurs="0"/>
* <element name="colgroup" type="{urn:riv:ehr:patientsummary:1}StrucDoc.ColGroup" maxOccurs="unbounded" minOccurs="0"/>
* <element name="thead" type="{urn:riv:ehr:patientsummary:1}StrucDoc.TRowGroup" minOccurs="0"/>
* <element name="tfoot" type="{urn:riv:ehr:patientsummary:1}StrucDoc.TRowGroup" minOccurs="0"/>
* <element name="tbody" type="{urn:riv:ehr:patientsummary:1}StrucDoc.TRowGroup" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="summary" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="width" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Length" />
* <attribute name="border" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Length" />
* <attribute name="frame" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Frame" />
* <attribute name="rules" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Rules" />
* <attribute name="cellspacing" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Length" />
* <attribute name="cellpadding" type="{urn:riv:ehr:patientsummary:1}StrucDoc.Length" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "StrucDoc.Table")
public class StrucDocTable
extends StrucDocCaptioned
{
@XmlAttribute(name = "summary")
protected String summary;
@XmlAttribute(name = "width")
protected String width;
@XmlAttribute(name = "border")
protected String border;
@XmlAttribute(name = "frame")
protected StrucDocFrame frame;
@XmlAttribute(name = "rules")
protected StrucDocRules rules;
@XmlAttribute(name = "cellspacing")
protected String cellspacing;
@XmlAttribute(name = "cellpadding")
protected String cellpadding;
/**
* Gets the value of the summary property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSummary() {
return summary;
}
/**
* Sets the value of the summary property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSummary(String value) {
this.summary = value;
}
/**
* Gets the value of the width property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWidth() {
return width;
}
/**
* Sets the value of the width property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWidth(String value) {
this.width = value;
}
/**
* Gets the value of the border property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBorder() {
return border;
}
/**
* Sets the value of the border property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBorder(String value) {
this.border = value;
}
/**
* Gets the value of the frame property.
*
* @return
* possible object is
* {@link StrucDocFrame }
*
*/
public StrucDocFrame getFrame() {
return frame;
}
/**
* Sets the value of the frame property.
*
* @param value
* allowed object is
* {@link StrucDocFrame }
*
*/
public void setFrame(StrucDocFrame value) {
this.frame = value;
}
/**
* Gets the value of the rules property.
*
* @return
* possible object is
* {@link StrucDocRules }
*
*/
public StrucDocRules getRules() {
return rules;
}
/**
* Sets the value of the rules property.
*
* @param value
* allowed object is
* {@link StrucDocRules }
*
*/
public void setRules(StrucDocRules value) {
this.rules = value;
}
/**
* Gets the value of the cellspacing property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCellspacing() {
return cellspacing;
}
/**
* Sets the value of the cellspacing property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCellspacing(String value) {
this.cellspacing = value;
}
/**
* Gets the value of the cellpadding property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCellpadding() {
return cellpadding;
}
/**
* Sets the value of the cellpadding property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCellpadding(String value) {
this.cellpadding = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy