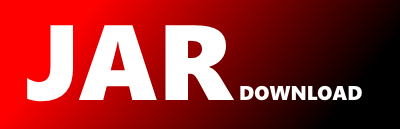
se.swedenconnect.schemas.saml_1_1.assertion.ObjectFactory Maven / Gradle / Ivy
Show all versions of oasis-dss-jaxb Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2022.10.06 at 01:06:56 PM CEST
//
package se.swedenconnect.schemas.saml_1_1.assertion;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the se.swedenconnect.schemas.saml_1_1.assertion package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AssertionIDReference_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "AssertionIDReference");
private final static QName _Audience_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "Audience");
private final static QName _Condition_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "Condition");
private final static QName _Statement_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "Statement");
private final static QName _SubjectStatement_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "SubjectStatement");
private final static QName _NameIdentifier_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "NameIdentifier");
private final static QName _ConfirmationMethod_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "ConfirmationMethod");
private final static QName _SubjectConfirmationData_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "SubjectConfirmationData");
private final static QName _AttributeValue_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "AttributeValue");
private final static QName _AttributeDesignator_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:assertion", "AttributeDesignator");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: se.swedenconnect.schemas.saml_1_1.assertion
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link NameIdentifierType }
*
*/
public NameIdentifierType createNameIdentifierType() {
return new NameIdentifierType();
}
/**
* Create an instance of {@link Assertion }
*
*/
public Assertion createAssertion() {
return new Assertion();
}
/**
* Create an instance of {@link Conditions }
*
*/
public Conditions createConditions() {
return new Conditions();
}
/**
* Create an instance of {@link AudienceRestrictionCondition }
*
*/
public AudienceRestrictionCondition createAudienceRestrictionCondition() {
return new AudienceRestrictionCondition();
}
/**
* Create an instance of {@link DoNotCacheCondition }
*
*/
public DoNotCacheCondition createDoNotCacheCondition() {
return new DoNotCacheCondition();
}
/**
* Create an instance of {@link Advice }
*
*/
public Advice createAdvice() {
return new Advice();
}
/**
* Create an instance of {@link AuthenticationStatement }
*
*/
public AuthenticationStatement createAuthenticationStatement() {
return new AuthenticationStatement();
}
/**
* Create an instance of {@link Subject }
*
*/
public Subject createSubject() {
return new Subject();
}
/**
* Create an instance of {@link SubjectConfirmation }
*
*/
public SubjectConfirmation createSubjectConfirmation() {
return new SubjectConfirmation();
}
/**
* Create an instance of {@link SubjectLocality }
*
*/
public SubjectLocality createSubjectLocality() {
return new SubjectLocality();
}
/**
* Create an instance of {@link AuthorityBinding }
*
*/
public AuthorityBinding createAuthorityBinding() {
return new AuthorityBinding();
}
/**
* Create an instance of {@link AuthorizationDecisionStatement }
*
*/
public AuthorizationDecisionStatement createAuthorizationDecisionStatement() {
return new AuthorizationDecisionStatement();
}
/**
* Create an instance of {@link Action }
*
*/
public Action createAction() {
return new Action();
}
/**
* Create an instance of {@link Evidence }
*
*/
public Evidence createEvidence() {
return new Evidence();
}
/**
* Create an instance of {@link AttributeStatement }
*
*/
public AttributeStatement createAttributeStatement() {
return new AttributeStatement();
}
/**
* Create an instance of {@link Attribute }
*
*/
public Attribute createAttribute() {
return new Attribute();
}
/**
* Create an instance of {@link AttributeDesignatorType }
*
*/
public AttributeDesignatorType createAttributeDesignatorType() {
return new AttributeDesignatorType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "AssertionIDReference")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
public JAXBElement createAssertionIDReference(String value) {
return new JAXBElement(_AssertionIDReference_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "Audience")
public JAXBElement createAudience(String value) {
return new JAXBElement(_Audience_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ConditionAbstractType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ConditionAbstractType }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "Condition")
public JAXBElement createCondition(ConditionAbstractType value) {
return new JAXBElement(_Condition_QNAME, ConditionAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StatementAbstractType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link StatementAbstractType }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "Statement")
public JAXBElement createStatement(StatementAbstractType value) {
return new JAXBElement(_Statement_QNAME, StatementAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SubjectStatementAbstractType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SubjectStatementAbstractType }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "SubjectStatement")
public JAXBElement createSubjectStatement(SubjectStatementAbstractType value) {
return new JAXBElement(_SubjectStatement_QNAME, SubjectStatementAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link NameIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link NameIdentifierType }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "NameIdentifier")
public JAXBElement createNameIdentifier(NameIdentifierType value) {
return new JAXBElement(_NameIdentifier_QNAME, NameIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "ConfirmationMethod")
public JAXBElement createConfirmationMethod(String value) {
return new JAXBElement(_ConfirmationMethod_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:assertion", name = "SubjectConfirmationData")
public JAXBElement