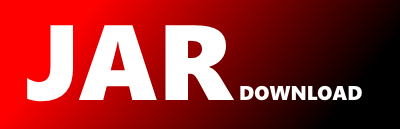
se.swedenconnect.spring.saml.idp.settings.AbstractSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-saml-idp Show documentation
Show all versions of spring-saml-idp Show documentation
Spring SAML Identity Provider Core
/*
* Copyright 2023-2024 Sweden Connect
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package se.swedenconnect.spring.saml.idp.settings;
import org.springframework.util.Assert;
import se.swedenconnect.spring.saml.idp.Saml2IdentityProviderVersion;
import java.io.Serial;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.function.Consumer;
/**
* Base implementation for configuration settings.
*
* @author Martin Lindström
*/
public abstract class AbstractSettings implements Serializable {
/** For serializing. */
@Serial
private static final long serialVersionUID = Saml2IdentityProviderVersion.SERIAL_VERSION_UID;
/** The settings. */
private final Map settings;
/**
* Constructor.
*
* @param settings the settings
*/
protected AbstractSettings(final Map settings) {
this.settings = Map.copyOf(settings);
}
/**
* Gets a named configuration setting.
*
* @param name the name of the setting
* @param the type of the setting
* @return the setting value, or null if not available
*/
@SuppressWarnings("unchecked")
public T getSetting(final String name) {
Assert.hasText(name, "name cannot be empty");
return (T) this.getSettings().get(name);
}
/**
* Returns a {@code Map} of the configuration settings.
*
* @return a map
*/
public Map getSettings() {
return this.settings;
}
/** {@inheritDoc} */
@Override
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (obj == null || this.getClass() != obj.getClass()) {
return false;
}
final AbstractSettings that = (AbstractSettings) obj;
return this.settings.equals(that.settings);
}
/** {@inheritDoc} */
@Override
public int hashCode() {
return Objects.hash(this.settings);
}
/** {@inheritDoc} */
@Override
public String toString() {
return String.format("%s", this.settings);
}
/**
* A builder for subclasses of {@link AbstractSettings}.
*/
protected static abstract class AbstractBuilder> {
private final Map settings = new HashMap<>();
/**
* Constructor.
*/
protected AbstractBuilder() {
}
/**
* Assigns a configuration setting.
*
* @param name the setting name
* @param value the setting value
* @return the builder
*/
public B setting(final String name, final Object value) {
Assert.hasText(name, "name cannot be empty");
if (value == null) {
return this.getThis();
}
this.getSettings().put(name, value);
return this.getThis();
}
/**
* A {@code Consumer} of the configuration settings {@code Map} allowing the ability to add, replace, or remove.
*
* @param settingsConsumer a Consumer of the configuration settings Map
* @return the builder
*/
public B settings(final Consumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy