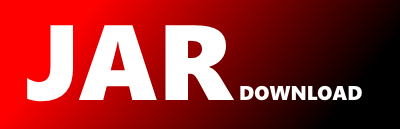
se.ugli.java.util.stream.Collectors Maven / Gradle / Ivy
package se.ugli.java.util.stream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import se.ugli.java.util.ImmutableList;
import se.ugli.java.util.ImmutableMap;
import se.ugli.java.util.ImmutableSet;
import se.ugli.java.util.juc.ImmutableListImpl;
import se.ugli.java.util.juc.ImmutableMapImpl;
import se.ugli.java.util.juc.ImmutableSetImpl;
public class Collectors {
private Collectors() {
}
public static Collector> toImmutableSet(final Supplier> mutableSetFactory,
final Function, ImmutableSet> immutableSetFactory) {
return Collector.of(mutableSetFactory, (list, e) -> list.add(e), (left, right) -> {
left.addAll(right);
return left;
}, immutableSetFactory);
}
public static Collector> toImmutableList(final Supplier> mutableListFactory,
final Function, ImmutableList> immutableListFactory) {
return Collector.of(mutableListFactory, (list, e) -> list.add(e), (left, right) -> {
left.addAll(right);
return left;
}, immutableListFactory);
}
public static Collector> toImmutableList() {
return toImmutableList(ArrayList::new, list -> new ImmutableListImpl<>(list));
}
public static Collector> toImmutableSet() {
return toImmutableSet(HashSet::new, set -> new ImmutableSetImpl<>(set));
}
public static Collector> toImmutableMap(
final Function super T, ? extends K> keyMapper, final Function super T, ? extends V> valueMapper) {
return toImmutableMap(keyMapper, valueMapper, HashMap::new, map -> new ImmutableMapImpl<>(map));
}
public static Collector> toImmutableMap(
final Function super T, ? extends K> keyMapper, final Function super T, ? extends V> valueMapper,
final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy