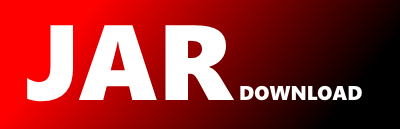
se.vgregion.mobile.hriv.service.WebserviceFake Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2010 Västra Götalandsregionen
*
* This library is free software; you can redistribute it and/or modify
* it under the terms of version 2.1 of the GNU Lesser General Public
* License as published by the Free Software Foundation.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the
* Free Software Foundation, Inc., 59 Temple Place, Suite 330,
* Boston, MA 02111-1307 USA
*/
package se.vgregion.mobile.hriv.service;
import com.thoughtworks.xstream.XStream;
import org.springframework.core.io.ClassPathResource;
import se.vgregion.mobile.hriv.utils.KivwsUnitMapper;
import se.vgregion.mobile.hriv.kivws.*;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import java.util.List;
public class WebserviceFake implements SearchService {
private ArrayOfUnit kivwsCareUnits;
private ArrayOfUnit kivwsEmergencyUnits;
private ArrayOfUnit kivwsDutyUnits;
private KivwsUnitMapper kivwsUnitMapper;
public void setKivwsUnitMapp(KivwsUnitMapper kivwsUnitMapper) {
this.kivwsUnitMapper = kivwsUnitMapper;
}
public WebserviceFake() {
try {
ClassPathResource kivwsCareUnitsResource = new ClassPathResource(
"careUnits.xml");
ClassPathResource kivwsEmergencyUnitsResource = new ClassPathResource(
"emergencyUnits.xml");
ClassPathResource kivwsDutyUnitsResource = new ClassPathResource(
"dutyUnits.xml");
XStream xStream = new XStream();
ObjectInputStream kivwsCareInputStream = xStream
.createObjectInputStream(kivwsCareUnitsResource
.getInputStream());
ObjectInputStream kivwsEmergencyInputStream = xStream
.createObjectInputStream(kivwsEmergencyUnitsResource
.getInputStream());
ObjectInputStream kivwsDutyInputStream = xStream
.createObjectInputStream(kivwsDutyUnitsResource
.getInputStream());
kivwsCareUnits = (ArrayOfUnit) kivwsCareInputStream.readObject();
kivwsEmergencyUnits = (ArrayOfUnit) kivwsEmergencyInputStream
.readObject();
kivwsDutyUnits = (ArrayOfUnit) kivwsDutyInputStream.readObject();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public ArrayOfUnit searchUnit(String arg0, ArrayOfString arg1,
VGRegionDirectory arg2, String arg3) throws VGRException_Exception {
ArrayOfUnit arrayOfUnit = null;
if (SearchService.CAREUNITS_LDAP_QUREY.equals(arg0)) {
arrayOfUnit = kivwsCareUnits;
} else if (SearchService.EMERGENCYUNITS_LDAP_QUREY.equals(arg0)) {
arrayOfUnit = kivwsEmergencyUnits;
} else if (SearchService.DUTYUNITS_LDAP_QUREY.equals(arg0)) {
arrayOfUnit = kivwsDutyUnits;
}
return arrayOfUnit;
}
@Override
public List searchUnits(
String filter, int searchScope, List attrs) {
ArrayList result = new ArrayList();
try {
ArrayOfUnit searchUnit = searchUnit(filter, null, null, null);
for (Unit unit : searchUnit.getUnit()) {
result.add(kivwsUnitMapper.mapFromContext(unit));
}
} catch (VGRException_Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy