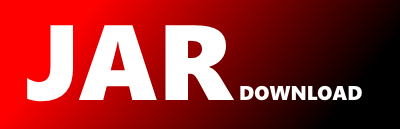
se.wfh.libs.common.gui.widgets.WComboBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gui Show documentation
Show all versions of gui Show documentation
Some helper classes i needed for several projects (GUI / Swing parts)
The newest version!
package se.wfh.libs.common.gui.widgets;
import java.util.Arrays;
import java.util.Collection;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JComboBox;
import se.wfh.libs.common.gui.exceptions.ChangeVetoException;
import se.wfh.libs.common.gui.widgets.base.AbstractWComponent;
import se.wfh.libs.common.gui.widgets.base.EnumSelection;
public class WComboBox extends AbstractWComponent> implements
EnumSelection {
private static final long serialVersionUID = 1L;
private final DefaultComboBoxModel model;
public WComboBox(final Collection items) {
this(items, null);
}
@SuppressWarnings("unchecked")
public WComboBox(final Collection items, E value) {
super(new JComboBox<>(), value);
JComboBox component = getComponent();
model = new DefaultComboBoxModel<>();
if (items != null) {
items.forEach(item -> model.addElement(item));
}
component.setModel(model);
setValue(value);
component.addActionListener(event -> setValue((E) model.getSelectedItem()));
}
public WComboBox(final E[] items) {
this(Arrays.asList(items));
}
public void addElement(final E element) {
model.addElement(element);
}
public void clear() {
model.removeAllElements();
}
@Override
protected void currentValueChanging(E newVal) throws ChangeVetoException {
getComponent().setSelectedItem(newVal);
}
@Override
public boolean isReadonly() {
return !getComponent().isEnabled();
}
public void removeElement(final E element) {
model.removeElement(element);
}
@Override
public void setReadonly(boolean readonly) {
getComponent().setEnabled(!readonly);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy