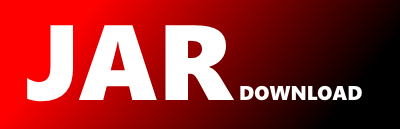
se.wfh.libs.common.utils.DateTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
Some helper classes i needed for several projects (Utils)
package se.wfh.libs.common.utils;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Locale;
/**
* Several Helper Methods to work with dates and times.
*
* @author Simon Frankenberger
* @since 0.2
*/
public final class DateTools {
/** cached formatter for exports, allways american format */
private static final SimpleDateFormat EXPORT_FORMATER = new SimpleDateFormat(
"yyyy-MM-dd HH:mm:ss", Locale.ENGLISH);
public static enum ConvertType {
DATE,
TIME,
DATE_TIME,
EXPORT,
}
/**
* Strips the seconds and milliseconds from the given calendar.
*
* @param cal
* The calendar to remove the fields
* @return The given calender without seconds and milliseconds
*/
public static Calendar cleanSeconds(final Calendar cal) {
if (cal != null) {
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
}
return cal;
}
/**
* Strips the seconds and milliseconds from the given date.
*
* @param date
* The date to remove the fields
* @return The given date without seconds and milliseconds
*/
public static Date cleanSeconds(final Date date) {
if (date == null) {
return null;
}
Calendar cal = new GregorianCalendar();
cal.setTime(date);
return cleanSeconds(cal).getTime();
}
/**
* Strips the time from the given calendar.
*
* @param cal
* The calendar to remove the fields
* @return The given calender without hours, minutes, seconds and milliseconds
*/
public static Calendar cleanTime(final Calendar cal) {
if (cal != null) {
cal.set(Calendar.HOUR_OF_DAY, 0);
cal.set(Calendar.MINUTE, 0);
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
}
return cal;
}
/**
* Strips the time from the given calendar.
*
* @param date
* The date to remove the fields
* @return The given calender without hours, minutes, seconds and milliseconds
*/
public static Date cleanTime(final Date date) {
if (date == null) {
return null;
}
Calendar cal = new GregorianCalendar();
cal.setTime(date);
return cleanTime(cal).getTime();
}
/**
* Parses the given string and extract the specified fields.
*
* @param str
* The string to parse.
* @param type
* The fields to extract / The syntax of the string
* @return A calendar representing the string contents.
* @throws ParseException
* If the given string is invalid.
*/
public static Calendar fromString(final String str, ConvertType type)
throws ParseException {
if (str == null) {
return null;
}
final GregorianCalendar cal = new GregorianCalendar();
final DateFormat format;
switch (type) {
case DATE:
format = DateFormat.getDateInstance();
break;
case DATE_TIME:
format = DateFormat.getDateTimeInstance();
break;
case EXPORT:
synchronized (EXPORT_FORMATER) {
format = EXPORT_FORMATER;
}
break;
case TIME:
format = DateFormat.getTimeInstance();
break;
default:
format = null;
break;
}
if (format == null) {
return null;
} else {
synchronized (format) {
cal.setTime(format.parse(str));
}
}
return cal;
}
/**
* Format the given date and return a string representation of it.
*
* @param date
* The calender to format.
* @param type
* The fields to export / The syntax of the resulting string
* @return A string representation of the date.
*/
public static String toString(final Date date, final ConvertType type) {
if (date == null) {
return null;
}
final DateFormat format;
switch (type) {
case DATE:
format = DateFormat.getDateInstance();
break;
case DATE_TIME:
format = DateFormat.getDateTimeInstance();
break;
case EXPORT:
format = EXPORT_FORMATER;
break;
case TIME:
format = DateFormat.getTimeInstance();
break;
default:
format = null;
break;
}
if (format == null) {
return null;
} else {
return format.format(date);
}
}
/**
* Private constructor, not used as this class has only static methods.
*/
private DateTools() {
// hide constructor
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy