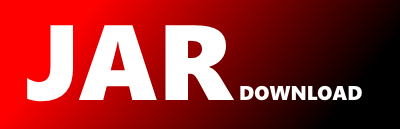
se.wfh.libs.common.utils.HashTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
Some helper classes i needed for several projects (Utils)
package se.wfh.libs.common.utils;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.Charset;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
/**
*
* @author Simon Frankenberger
* @since 0.14
*/
public final class HashTools {
/** the charset used to convert strings to raw data */
private static Charset charset = Charset.forName("UTF-8");
/**
* @return The default charset used for String to binary raw data
* transformations.
*/
public static Charset getCharset() {
return charset;
}
public static MessageDigest getMd5() throws NoSuchAlgorithmException {
return MessageDigest.getInstance("MD5");
}
public static MessageDigest getSha1() throws NoSuchAlgorithmException {
return MessageDigest.getInstance("SHA-1");
}
public static MessageDigest getSha256() throws NoSuchAlgorithmException {
return MessageDigest.getInstance("SHA-256");
}
public static MessageDigest getSha512() throws NoSuchAlgorithmException {
return MessageDigest.getInstance("SHA-512");
}
public static byte[] hash(final MessageDigest digest, final byte[] data) {
// reset digest engine
digest.reset();
// determine length of data
int length = data.length;
// if length is very small, directly pass it it to engine
if (length < 1024) {
digest.update(data);
} else {
// calculate index after last full update
final int lastFull = length - length % 1024;
// update engine in 10240b blocks
for (int i = 0; i < lastFull; i += 1024) {
digest.update(data, i, 1024);
}
// do final update with last not full block
digest.update(data, lastFull, length - lastFull);
}
return digest.digest();
}
public static byte[] hash(final MessageDigest digest, final String data) {
// convert the string to raw data and return hash
return hash(digest, data.getBytes(charset));
}
public static byte[] hashFile(final MessageDigest digest,
final InputStream is, final long length) throws IOException {
// Reset engine
digest.reset();
// how much data was read so far?
long read = 0;
// a buffer storing the pieces
byte[] buffer = new byte[10240];
// as long as we have not reached the eof
while (read < length) {
// read a piece
int temp = is.read(buffer);
// if nothing was read, something went wrong, so abort
if (temp == -1) {
throw new IOException("Premature end of file detected!");
}
// update the digest engine with the read data
digest.update(buffer, 0, temp);
// update the read data counter
read += temp;
} // end of reading loop
return digest.digest();
}
public static byte[] hashFile(final MessageDigest digest, final File file)
throws IOException {
try (FileInputStream fis = new FileInputStream(file)) {
return hashFile(digest, fis, file.length());
}
}
/**
* Converts a raw hash to a human readable hex-string.
*
* @param hash
* The hash to convert
* @return A human readable representation of the raw hash.
*/
public static String hashToString(final byte[] hash) {
// convert the byte to hex format method 2
final StringBuffer hexString = new StringBuffer();
for (byte element : hash) {
final String str = Integer.toHexString(0xFF & element);
if (str.length() == 1) {
hexString.append('0');
}
hexString.append(str);
}
return hexString.toString();
}
/**
* Set the default charset used to convert Strings to raw binary data.
*
* @param charset
* The default charset to use.
*/
public static void setCharset(final Charset charset) {
HashTools.charset = charset;
}
private HashTools() {
// hide constructor
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy