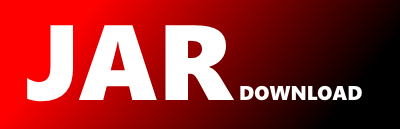
sg.dex.starfish.impl.memory.MemoryJob Maven / Gradle / Ivy
package sg.dex.starfish.impl.memory;
import sg.dex.starfish.Job;
import sg.dex.starfish.constant.Constant;
import sg.dex.starfish.exception.JobFailedException;
import sg.dex.starfish.util.Hex;
import sg.dex.starfish.util.Utils;
import java.util.Map;
import java.util.concurrent.CancellationException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
/**
* Class representing a Job being conducted asynchronously in the local JVM, which wraps
* an arbitrary Future.
*
* @author Mike
*/
public class MemoryJob implements Job {
private final Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy