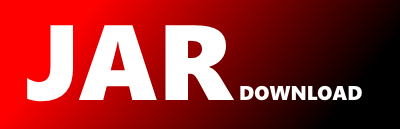
sg.dex.starfish.util.JSON Maven / Gradle / Ivy
package sg.dex.starfish.util;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
import sg.dex.starfish.exception.StarfishValidationException;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Utility class for handling JSON objects
*
* This class include method to covert json string to map , parse the json
* string.
*
*
* @author Mike
* @version 0.5
*/
public class JSON {
private static String WHITESPACE = " ";
private static int WHITESPACE_LENGTH = WHITESPACE.length();
/**
* Converts an object to an efficient JSON string representation
*
* @param value Object to represent as a JSON String
* @return JSON string representing the value
* @throws RuntimeException on failure to create JSON from value
*/
public static String toString(Object value) {
ObjectMapper Obj = new ObjectMapper();
try {
return Obj.writeValueAsString(value);
} catch (IOException e) {
throw new StarfishValidationException("Converts an object" + value.toString() + " to an efficient JSON string failed", e);
}
}
/**
* Converts an object to a pretty-printed JSON string representation suitable
* for human consumption
*
* @param value Object to represent as a JSON String
* @return JSON string representing the value
*/
public static String toPrettyString(Object value) {
StringBuilder sb = new StringBuilder();
sb = appendPrettyString(sb, value, 0);
return sb.toString();
}
@SuppressWarnings("unchecked")
private static StringBuilder appendPrettyString(StringBuilder sb, Object o, int indent) {
if (o instanceof Map) {
int entryIndent = indent + 2;
sb.append("{\n");
Map m = ((Map) o);
int size = m.size();
int pos = 0;
for (Map.Entry me : m.entrySet()) {
String k = me.getKey();
sb = appendWhitespaceString(sb, entryIndent);
sb.append(toString(k));
sb.append(": ");
int vIndent = entryIndent + k.length() + 4; // indent for value
Object v = me.getValue();
appendPrettyString(sb, v, vIndent);
pos++;
if (pos == size) {
sb.append('\n'); // final entry
} else {
sb.append(",\n"); // comma for next entry
}
}
sb = appendWhitespaceString(sb, indent);
sb.append("}");
} else if (o instanceof List) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy