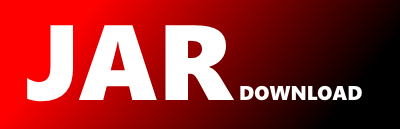
commonMain.sh.calvin.reorderable.ReorderableLazyGrid.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reorderable-jvm Show documentation
Show all versions of reorderable-jvm Show documentation
A library for reordering items in a LazyColumn
/*
* Copyright 2023 Calvin Liang
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package sh.calvin.reorderable
import androidx.compose.animation.core.AnimationSpec
import androidx.compose.foundation.ExperimentalFoundationApi
import androidx.compose.foundation.gestures.Orientation
import androidx.compose.foundation.gestures.animateScrollBy
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.layout.calculateEndPadding
import androidx.compose.foundation.layout.calculateStartPadding
import androidx.compose.foundation.lazy.grid.LazyGridItemInfo
import androidx.compose.foundation.lazy.grid.LazyGridItemScope
import androidx.compose.foundation.lazy.grid.LazyGridLayoutInfo
import androidx.compose.foundation.lazy.grid.LazyGridState
import androidx.compose.runtime.Composable
import androidx.compose.runtime.Stable
import androidx.compose.runtime.State
import androidx.compose.runtime.getValue
import androidx.compose.runtime.remember
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.runtime.rememberUpdatedState
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.graphicsLayer
import androidx.compose.ui.platform.LocalDensity
import androidx.compose.ui.platform.LocalLayoutDirection
import androidx.compose.ui.unit.Dp
import androidx.compose.ui.unit.IntOffset
import androidx.compose.ui.unit.IntSize
import androidx.compose.ui.unit.LayoutDirection
import androidx.compose.ui.unit.dp
import androidx.compose.ui.zIndex
import kotlinx.coroutines.CoroutineScope
/**
* Creates a [ReorderableLazyGridState] that is remembered across compositions.
*
* Changes to [lazyGridState], [scrollThresholdPadding], [scrollThreshold], and [scroller] will result in [ReorderableLazyGridState] being updated.
*
* @param lazyGridState The return value of [rememberLazyGridState](androidx.compose.foundation.lazy.LazyGridStateKt.rememberLazyGridState)
* @param scrollThresholdPadding The padding that will be added to the top and bottom, or start and end of the grid to determine the scrollThreshold. Useful for when the grid is displayed under the navigation bar or notification bar.
* @param scrollThreshold The distance in dp from the top and bottom, or start and end of the grid that will trigger scrolling
* @param scroller The [Scroller] that will be used to scroll the grid. Use [rememberScroller](sh.calvin.reorderable.ScrollerKt.rememberScroller) to create a [Scroller].
* @param onMove The function that is called when an item is moved. Make sure this function returns only after the items are moved. This suspend function is invoked with the `rememberReorderableLazyGridState` scope, allowing for async processing, if desired. Note that the scope used here is the one provided by the composition where `rememberReorderableLazyGridState` is called, for long running work that needs to outlast `rememberReorderableLazyGridState` being in the composition you should use a scope that fits the lifecycle needed.
*/
@Composable
fun rememberReorderableLazyGridState(
lazyGridState: LazyGridState,
scrollThresholdPadding: PaddingValues = PaddingValues(0.dp),
scrollThreshold: Dp = ReorderableLazyCollectionDefaults.ScrollThreshold,
scroller: Scroller = rememberScroller(
scrollableState = lazyGridState,
pixelAmountProvider = { lazyGridState.layoutInfo.mainAxisViewportSize * ScrollAmountMultiplier },
),
onMove: suspend CoroutineScope.(from: LazyGridItemInfo, to: LazyGridItemInfo) -> Unit,
): ReorderableLazyGridState {
val density = LocalDensity.current
val scrollThresholdPx = with(density) { scrollThreshold.toPx() }
val scope = rememberCoroutineScope()
val onMoveState = rememberUpdatedState(onMove)
val layoutDirection = LocalLayoutDirection.current
val absoluteScrollThresholdPadding = AbsolutePixelPadding(
start = with(density) {
scrollThresholdPadding.calculateStartPadding(layoutDirection).toPx()
},
end = with(density) {
scrollThresholdPadding.calculateEndPadding(layoutDirection).toPx()
},
top = with(density) { scrollThresholdPadding.calculateTopPadding().toPx() },
bottom = with(density) { scrollThresholdPadding.calculateBottomPadding().toPx() },
)
val state = remember(
scope, lazyGridState, scrollThreshold, scrollThresholdPadding, scroller,
) {
ReorderableLazyGridState(
state = lazyGridState,
scope = scope,
onMoveState = onMoveState,
scrollThreshold = scrollThresholdPx,
scrollThresholdPadding = absoluteScrollThresholdPadding,
scroller = scroller,
layoutDirection = layoutDirection,
)
}
return state
}
private val LazyGridLayoutInfo.mainAxisViewportSize: Int
get() = when (orientation) {
Orientation.Vertical -> viewportSize.height
Orientation.Horizontal -> viewportSize.width
}
private fun LazyGridItemInfo.toLazyCollectionItemInfo() =
object : LazyCollectionItemInfo {
override val index: Int
get() = [email protected]
override val key: Any
get() = [email protected]
override val offset: IntOffset
get() = [email protected]
override val size: IntSize
get() = [email protected]
override val data: LazyGridItemInfo
get() = this@toLazyCollectionItemInfo
}
private fun LazyGridLayoutInfo.toLazyCollectionLayoutInfo() =
object : LazyCollectionLayoutInfo {
override val visibleItemsInfo: List>
get() = [email protected] {
it.toLazyCollectionItemInfo()
}
override val viewportSize: IntSize
get() = [email protected]
override val orientation: Orientation
get() = [email protected]
override val reverseLayout: Boolean
get() = [email protected]
override val beforeContentPadding: Int
get() = [email protected]
}
private fun LazyGridState.toLazyCollectionState() =
object : LazyCollectionState {
override val firstVisibleItemIndex: Int
get() = [email protected]
override val firstVisibleItemScrollOffset: Int
get() = [email protected]
override val layoutInfo: LazyCollectionLayoutInfo
get() = [email protected]()
override suspend fun animateScrollBy(value: Float, animationSpec: AnimationSpec) =
[email protected](value, animationSpec)
override suspend fun scrollToItem(scrollToIndex: Int, firstVisibleItemScrollOffset: Int) =
[email protected](scrollToIndex, firstVisibleItemScrollOffset)
}
@Stable
class ReorderableLazyGridState internal constructor(
state: LazyGridState,
scope: CoroutineScope,
onMoveState: State Unit>,
/**
* The threshold in pixels for scrolling the grid when dragging an item.
* If the dragged item is within this threshold of the top or bottom of the grid, the grid will scroll.
* Must be greater than 0.
*/
scrollThreshold: Float,
scrollThresholdPadding: AbsolutePixelPadding,
scroller: Scroller,
layoutDirection: LayoutDirection,
) : ReorderableLazyCollectionState(
state.toLazyCollectionState(),
scope,
onMoveState,
scrollThreshold,
scrollThresholdPadding,
scroller,
layoutDirection,
)
/**
* A composable that allows an item in LazyVerticalGrid or LazyHorizontalGrid to be reordered by dragging.
*
* @param state The return value of [rememberReorderableLazyGridState]
* @param key The key of the item, must be the same as the key passed to [LazyGridScope.item](androidx.compose.foundation.lazy.grid.item), [LazyGridScope.items](androidx.compose.foundation.lazy.grid.items) or similar functions in [LazyGridScope](androidx.compose.foundation.lazy.grid.LazyGridScope)
* @param enabled Whether or this item is reorderable. If true, the item will not move for other items but may still be draggable. To make an item not draggable, set `enable = false` in [Modifier.draggable] or [Modifier.longPressDraggable] instead.
* @param animateItemModifier The [Modifier] that will be applied to items that are not being dragged.
*/
@ExperimentalFoundationApi
@Composable
fun LazyGridItemScope.ReorderableItem(
state: ReorderableLazyGridState,
key: Any,
modifier: Modifier = Modifier,
enabled: Boolean = true,
animateItemModifier: Modifier = Modifier.animateItemPlacement(),
content: @Composable ReorderableCollectionItemScope.(isDragging: Boolean) -> Unit,
) {
val dragging by state.isItemDragging(key)
val offsetModifier = if (dragging) {
Modifier
.zIndex(1f)
.graphicsLayer {
translationY = state.draggingItemOffset.y
translationX = state.draggingItemOffset.x
}
} else if (key == state.previousDraggingItemKey) {
Modifier
.zIndex(1f)
.graphicsLayer {
translationY =
state.previousDraggingItemOffset.value.y
translationX =
state.previousDraggingItemOffset.value.x
}
} else {
animateItemModifier
}
ReorderableCollectionItem(
state = state,
key = key,
modifier = modifier.then(offsetModifier),
enabled = enabled,
dragging = dragging,
content = content,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy