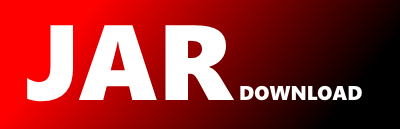
commonMain.tools.ozone.moderation.queryEvents.kt Maven / Gradle / Ivy
@file:Suppress("DEPRECATION")
package tools.ozone.moderation
import kotlin.Any
import kotlin.Boolean
import kotlin.Long
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlinx.collections.immutable.persistentListOf
import kotlinx.collections.immutable.toImmutableList
import kotlinx.serialization.Serializable
import sh.christian.ozone.api.Did
import sh.christian.ozone.api.Nsid
import sh.christian.ozone.api.Uri
import sh.christian.ozone.api.model.ReadOnlyList
import sh.christian.ozone.api.model.Timestamp
/**
* @param types The types of events (fully qualified string in the format of
* tools.ozone.moderation.defs#modEvent) to filter by. If not specified, all events are returned.
* @param sortDirection Sort direction for the events. Defaults to descending order of created at
* timestamp.
* @param createdAfter Retrieve events created after a given timestamp
* @param createdBefore Retrieve events created before a given timestamp
* @param collections If specified, only events where the subject belongs to the given collections
* will be returned. When subjectType is set to 'account', this will be ignored.
* @param subjectType If specified, only events where the subject is of the given type (account or
* record) will be returned. When this is set to 'account' the 'collections' parameter will be ignored.
* When includeAllUserRecords or subject is set, this will be ignored.
* @param includeAllUserRecords If true, events on all record types (posts, lists, profile etc.) or
* records from given 'collections' param, owned by the did are returned.
* @param hasComment If true, only events with comments are returned
* @param comment If specified, only events with comments containing the keyword are returned
* @param addedLabels If specified, only events where all of these labels were added are returned
* @param removedLabels If specified, only events where all of these labels were removed are
* returned
* @param addedTags If specified, only events where all of these tags were added are returned
* @param removedTags If specified, only events where all of these tags were removed are returned
*/
@Serializable
public data class QueryEventsQueryParams(
/**
* The types of events (fully qualified string in the format of
* tools.ozone.moderation.defs#modEvent) to filter by. If not specified, all events are
* returned.
*/
public val types: ReadOnlyList = persistentListOf(),
public val createdBy: Did? = null,
/**
* Sort direction for the events. Defaults to descending order of created at timestamp.
*/
public val sortDirection: String? = "desc",
/**
* Retrieve events created after a given timestamp
*/
public val createdAfter: Timestamp? = null,
/**
* Retrieve events created before a given timestamp
*/
public val createdBefore: Timestamp? = null,
public val subject: Uri? = null,
/**
* If specified, only events where the subject belongs to the given collections will be returned.
* When subjectType is set to 'account', this will be ignored.
*/
public val collections: ReadOnlyList = persistentListOf(),
/**
* If specified, only events where the subject is of the given type (account or record) will be
* returned. When this is set to 'account' the 'collections' parameter will be ignored. When
* includeAllUserRecords or subject is set, this will be ignored.
*/
public val subjectType: QueryEventsSubjectType? = null,
/**
* If true, events on all record types (posts, lists, profile etc.) or records from given
* 'collections' param, owned by the did are returned.
*/
public val includeAllUserRecords: Boolean? = false,
public val limit: Long? = 50,
/**
* If true, only events with comments are returned
*/
public val hasComment: Boolean? = null,
/**
* If specified, only events with comments containing the keyword are returned
*/
public val comment: String? = null,
/**
* If specified, only events where all of these labels were added are returned
*/
public val addedLabels: ReadOnlyList = persistentListOf(),
/**
* If specified, only events where all of these labels were removed are returned
*/
public val removedLabels: ReadOnlyList = persistentListOf(),
/**
* If specified, only events where all of these tags were added are returned
*/
public val addedTags: ReadOnlyList = persistentListOf(),
/**
* If specified, only events where all of these tags were removed are returned
*/
public val removedTags: ReadOnlyList = persistentListOf(),
public val reportTypes: ReadOnlyList = persistentListOf(),
public val cursor: String? = null,
) {
init {
require(collections.count() <= 20) {
"collections.count() must be <= 20, but was ${collections.count()}"
}
require(limit == null || limit >= 1) {
"limit must be >= 1, but was $limit"
}
require(limit == null || limit <= 100) {
"limit must be <= 100, but was $limit"
}
}
public fun asList(): ReadOnlyList> = buildList {
types.forEach {
add("types" to it)
}
add("createdBy" to createdBy)
add("sortDirection" to sortDirection)
add("createdAfter" to createdAfter)
add("createdBefore" to createdBefore)
add("subject" to subject)
collections.forEach {
add("collections" to it)
}
add("subjectType" to subjectType)
add("includeAllUserRecords" to includeAllUserRecords)
add("limit" to limit)
add("hasComment" to hasComment)
add("comment" to comment)
addedLabels.forEach {
add("addedLabels" to it)
}
removedLabels.forEach {
add("removedLabels" to it)
}
addedTags.forEach {
add("addedTags" to it)
}
removedTags.forEach {
add("removedTags" to it)
}
reportTypes.forEach {
add("reportTypes" to it)
}
add("cursor" to cursor)
}.toImmutableList()
}
@Serializable
public data class QueryEventsResponse(
public val cursor: String? = null,
public val events: ReadOnlyList,
)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy