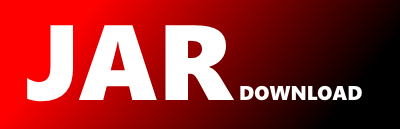
si.trina.socket.live.SocketConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of socket-live Show documentation
Show all versions of socket-live Show documentation
Communication with s7 PLCs helper classes
The newest version!
package si.trina.socket.live;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.LinkedList;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class SocketConnection implements Runnable {
final Logger logger = LoggerFactory.getLogger(SocketConnection.class);
public String name, ip;
public int port;
public DataOutputStream outToServer;
public InputStream inFromServer;
public Socket socket;
public LinkedList toServerQueue;
public ArrayList listeners;
public boolean connected = false;
public Object socketObjectLock, readerLock, writerLock, toServerQueueLock, fromServerQueueLock;
public boolean connectionError = false;
public String charsetName = "UTF-8";
public long reconnectInterval = 1000;
public long checkConnectionInterval = 1000;
public boolean readEnabled = true;
public boolean writeEnabled = true;
private SocketWrite socketWriteThread;
private SocketRead socketReadThread;
public SocketConnection(String name,String ip,int port) {
this.name = name;
this.ip = ip;
this.port = port;
this.toServerQueue = new LinkedList();
this.listeners = new ArrayList();
this.readerLock = new Object();
this.writerLock = new Object();
this.toServerQueueLock = new Object();
this.fromServerQueueLock = new Object();
this.socketObjectLock = new Object();
}
public int byteArrayToInt(byte[] b) {
return b[0] & 0xFF |
(b[1] & 0xFF) << 8 |
(b[2] & 0xFF) << 16 |
(b[3] & 0xFF) << 24;
}
// using least significant byte first
public short byteArrayToUInt16(byte[] b) {
if (b.length == 1) {
return (short) (b[0] & 0xFF);
}
return (short) (b[0] & 0xFF |
(b[1] & 0xFF) << 8);
}
public short byteArrayToUInt8(byte[] b) {
return (short) (b[0] & 0xFF);
}
public byte[] intToByteArray(int a) {
return new byte[] {
(byte) (a & 0xFF),
(byte) ((a >> 8) & 0xFF),
(byte) ((a >> 16) & 0xFF),
(byte) ((a >> 24) & 0xFF)
};
}
public byte[] uint16ToByteArray(short a)
{
return new byte[] {
(byte) (a & 0xFF),
(byte) ((a >> 8) & 0xFF)
};
}
public byte[] stringToByteArray(String str, int numBytes) {
byte[] stringBytes = null;
try {
stringBytes = str.getBytes(Charset.forName(this.charsetName));
} catch (Exception e) {
e.printStackTrace();
return stringBytes;
}
byte [] bytes = new byte[numBytes];
int len = stringBytes.length;
if (len > numBytes) {
len = numBytes;
}
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy