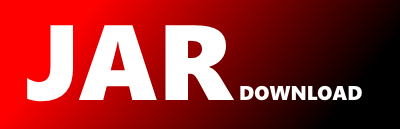
site.sorghum.anno._common.util.JSONUtil Maven / Gradle / Ivy
package site.sorghum.anno._common.util;
import cn.hutool.core.bean.BeanUtil;
import com.alibaba.fastjson2.*;
import site.sorghum.anno._common.exception.BizException;
import java.net.URL;
import java.util.List;
import java.util.Map;
/**
* json工具类
*
* @author Sorghum
* @since 2023/06/25
*/
public class JSONUtil {
public static List toBeanList(String json, Class objectClass) {
return JSONArray.parseArray(json, objectClass);
}
public static T toBean(Map,?> map, Class objectClass) {
if (map instanceof JSONObject) {
return ((JSONObject) map).toJavaObject(objectClass);
}
JSONObject jsonObject = new JSONObject();
BeanUtil.copyProperties(map,jsonObject);
return jsonObject.toJavaObject(objectClass, JSONReader.Feature.SupportSmartMatch);
}
public static T toBean(String json, Class objectClass) {
return JSONObject.parseObject(json, objectClass);
}
public static T toBean(Object object, Class objectClass) {
if (object instanceof Map) {
return toBean((Map, ?>) object, objectClass);
}
if (object instanceof String) {
return toBean((String) object, objectClass);
}
return JSONObject.parseObject(JSONObject.toJSONString(object), objectClass);
}
public static T toBean(URL url, Class objectClass) {
return JSON.parseObject(url, objectClass);
}
public static T copy(T object) {
return JSON.copy(object);
}
public static T read(Object obj, String path, Class type) {
Object eval = JSONPath.eval(obj, path);
if (eval instanceof JSONArray) {
throw new BizException("类型不匹配");
}
if (eval instanceof JSONObject evalJson) {
return evalJson.toJavaObject(type);
}
return JSONObject.parseObject(JSON.toJSONString(obj), type);
}
public static List readList(Object obj, String path, Class type) {
Object eval = JSONPath.eval(obj, path);
if (eval instanceof JSONArray evalArray) {
return evalArray.toJavaList(type);
}
if (eval instanceof JSONObject) {
throw new BizException("类型不匹配");
}
return JSONArray.parseArray(JSON.toJSONString(eval), type);
}
public static Object write(Object obj, String path, Object value) {
return JSONPath.set(obj, path, value);
}
public static String toJsonString(Object object) {
return JSON.toJSONString(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy