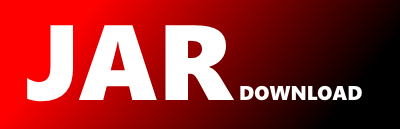
site.zfei.at.coxt.WebAdvisor Maven / Gradle / Ivy
package site.zfei.at.coxt;
import lombok.extern.slf4j.Slf4j;
import org.aspectj.lang.ProceedingJoinPoint;
import org.springframework.util.StringUtils;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import org.springframework.web.util.ContentCachingRequestWrapper;
import org.springframework.web.util.WebUtils;
import site.zfei.at.file.AtTraceConfigurationProperties;
import site.zfei.at.trace.WebLogBean;
import site.zfei.at.util.IPUntils;
import javax.servlet.http.HttpServletRequest;
/**
* @author fgh
*/
@Slf4j
public abstract class WebAdvisor {
protected AtTraceConfigurationProperties traceConfigurationProperties;
public WebAdvisor(AtTraceConfigurationProperties atProperties) {
this.traceConfigurationProperties = atProperties;
}
protected Object around(ProceedingJoinPoint jp) throws Throwable {
WebLogBean logBean = WebLogBean.getLogBean();
before(logBean);
postBefore(jp, logBean);
Object result = jp.proceed();
postAfter(result, jp, logBean);
after(logBean, result);
return result;
}
protected void postBefore(ProceedingJoinPoint jp, WebLogBean logBean) {
}
protected void postAfter(Object result, ProceedingJoinPoint jp, WebLogBean logBean) {
}
protected void before(WebLogBean logBean) {
RequestAttributes requestAttributes = null;
try {
requestAttributes = RequestContextHolder.currentRequestAttributes();
} catch (IllegalStateException e) {
log.warn("Please do not execute non-web-server requests in the Controller, such as scheduled tasks, as its speech will be intercepted by the Advisor.", e);
}
HttpServletRequest request = ((ServletRequestAttributes) requestAttributes).getRequest();
ContentCachingRequestWrapper requestWrapper = WebUtils.getNativeRequest(request, ContentCachingRequestWrapper.class);
String body = new String(requestWrapper != null ? requestWrapper.getContentAsByteArray() : ByteArrays.EMPTY);
if (StringUtils.isEmpty(body)) {
body = ByteArrays.EMPTY_BODY;
}
logBean.setPayload(body);
if (traceConfigurationProperties.isEnable() && Global.isPrintCurl(request.getRequestURI())) {
try {
log.info(IPUntils.getCurl(request, traceConfigurationProperties.getServerHost()));
} catch (Exception e) {
log.error(e.getMessage(), e);
}
}
}
protected void after(WebLogBean logBean, Object result) {
if (traceConfigurationProperties.isEnable() && Global.printResult) {
logBean.setResult(result);
}
}
static class ByteArrays {
/**
* The Empty Byte Array
*/
public static final byte[] EMPTY = new byte[0];
public static final String EMPTY_BODY = "{}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy