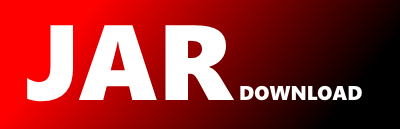
site.zfei.at.trace.WebLogBean Maven / Gradle / Ivy
package site.zfei.at.trace;
import cn.hutool.json.JSONUtil;
import lombok.Data;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import site.zfei.at.coxt.Context;
import site.zfei.at.util.Now;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* @author fgh
*/
@Data
public class WebLogBean implements LogBean {
private static final Logger logger = LoggerFactory.getLogger(WebLogBean.class);
public static final String KEY_LOG_BEAN = "X-WebLogBean";
public static WebLogBean getLogBean() {
return (WebLogBean) Context.current().getAttachments().computeIfAbsent(KEY_LOG_BEAN, k -> new WebLogBean());
}
public static void removeLogBean() {
Context.current().removeAttachment(KEY_LOG_BEAN);
}
public static WebLogBean start() {
WebLogBean logBean = getLogBean();
logBean.setBeginTime(Now.millis());
return logBean;
}
public static void stop() {
getLogBean().print();
removeLogBean();
}
private String trace;
private String uid;
private String path;
private String clientIp;
private int code = 200;
private long beginTime = 0;
private long spend = 0;
private Object headers;
private Object params;
private Object payload;
private Object result;
private String error;
private Map props = new LinkedHashMap<>();
@Override
public LogBean addProp(String key, Object value) {
if (this.getProps() != null) {
this.getProps().put(key, (value == null ? "null" : value));
}
return this;
}
public LogBean addPropFromMap(Map map, String... keys) {
for (String key : keys) {
if (map.containsKey(key)) {
this.addProp(key, map.get(key));
}
}
return this;
}
@Override
public Object delProp(String key) {
if (this.getProps() != null) {
return this.getProps().remove(key);
}
return null;
}
@Override
public Object getProp(String key) {
if (this.getProps() != null) {
return this.getProps().get(key);
}
return null;
}
@Override
public void log() {
logger.info(JSONUtil.toJsonStr(this));
}
public void print() {
this.print(Now.millis());
}
public void print(long now) {
this.setSpend(now - this.getBeginTime());
this.log();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy