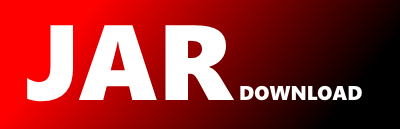
sk.seges.acris.server.AcrisSiteDtoConverterProvider Maven / Gradle / Ivy
The newest version!
package sk.seges.acris.server;
import javax.annotation.Generated;
import javax.persistence.EntityManager;
import sk.seges.acris.server.model.dto.configuration.CountryDTOConverter;
import sk.seges.acris.server.model.dto.configuration.FTPWebSettingsDTOConverter;
import sk.seges.acris.server.model.dto.configuration.MetaDataDTOConverter;
import sk.seges.acris.server.model.dto.configuration.WebSettingsDTOConverter;
import sk.seges.acris.server.model.dto.configuration.WebSitesDTOConverter;
import sk.seges.acris.shared.model.dto.CountryDTO;
import sk.seges.acris.shared.model.dto.FTPWebSettingsDTO;
import sk.seges.acris.shared.model.dto.MetaDataDTO;
import sk.seges.acris.shared.model.dto.WebSettingsDTO;
import sk.seges.acris.shared.model.dto.WebSitesDTO;
import sk.seges.acris.site.server.domain.jpa.JpaFTPWebSettings;
import sk.seges.acris.site.server.domain.jpa.JpaWebSettings;
import sk.seges.acris.site.server.domain.jpa.JpaWebSettings.JpaMetaData;
import sk.seges.acris.site.server.domain.jpa.JpaWebSites;
import sk.seges.corpis.pap.converter.hibernate.provider.AbstractTransactionalConverterProvider;
import sk.seges.corpis.server.domain.jpa.JpaCountry;
import sk.seges.sesam.pap.model.annotation.ConverterProviderDefinition;
import sk.seges.sesam.shared.model.converter.ConverterProviderContext;
import sk.seges.sesam.shared.model.converter.api.DtoConverter;
@ConverterProviderDefinition
@Generated(value = "sk.seges.corpis.pap.converter.hibernate.DataConverterProviderProcessor")
public class AcrisSiteDtoConverterProvider extends AbstractTransactionalConverterProvider {
protected final EntityManager entityManager;
protected final ConverterProviderContext converterProviderContext;
public AcrisSiteDtoConverterProvider(EntityManager entityManager, ConverterProviderContext converterProviderContext) {
this.entityManager = entityManager;
this.converterProviderContext = converterProviderContext;
}
public DtoConverter getConverterForDomain(Class domainClass) {
if (domainClass == null) {
return null;
}
if (JpaCountry.class.equals(domainClass)) {
return (DtoConverter)getDomainCountryDTOConverter();
}
if (JpaWebSettings.class.equals(domainClass)) {
return (DtoConverter)getDomainWebSettingsDTOConverter();
}
if (JpaWebSites.class.equals(domainClass)) {
return (DtoConverter)getDomainWebSitesDTOConverter();
}
if (JpaFTPWebSettings.class.equals(domainClass)) {
return (DtoConverter)getDomainFTPWebSettingsDTOConverter();
}
if (JpaMetaData.class.equals(domainClass)) {
return (DtoConverter)getDomainMetaDataDTOConverter();
}
return null;
}
public DtoConverter getConverterForDto(Class dto) {
if (dto == null) {
return null;
}
if (CountryDTO.class.equals(dto)) {
return (DtoConverter) getDtoCountryDTOConverter();
}
if (WebSettingsDTO.class.equals(dto)) {
return (DtoConverter) getDtoWebSettingsDTOConverter();
}
if (WebSitesDTO.class.equals(dto)) {
return (DtoConverter) getDtoWebSitesDTOConverter();
}
if (FTPWebSettingsDTO.class.equals(dto)) {
return (DtoConverter) getDtoFTPWebSettingsDTOConverter();
}
if (MetaDataDTO.class.equals(dto)) {
return (DtoConverter) getDtoMetaDataDTOConverter();
}
return null;
}
protected WebSettingsDTOConverter getDomainWebSettingsDTOConverter(){
WebSettingsDTOConverter result = new WebSettingsDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected WebSettingsDTOConverter getDtoWebSettingsDTOConverter(){
WebSettingsDTOConverter result = new WebSettingsDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected MetaDataDTOConverter getDomainMetaDataDTOConverter(){
MetaDataDTOConverter result = new MetaDataDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected MetaDataDTOConverter getDtoMetaDataDTOConverter(){
MetaDataDTOConverter result = new MetaDataDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected WebSitesDTOConverter getDomainWebSitesDTOConverter(){
WebSitesDTOConverter result = new WebSitesDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected WebSitesDTOConverter getDtoWebSitesDTOConverter(){
WebSitesDTOConverter result = new WebSitesDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected CountryDTOConverter getDomainCountryDTOConverter(){
CountryDTOConverter result = new CountryDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected CountryDTOConverter getDtoCountryDTOConverter(){
CountryDTOConverter result = new CountryDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected FTPWebSettingsDTOConverter getDomainFTPWebSettingsDTOConverter(){
FTPWebSettingsDTOConverter result = new FTPWebSettingsDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
protected FTPWebSettingsDTOConverter getDtoFTPWebSettingsDTOConverter(){
FTPWebSettingsDTOConverter result = new FTPWebSettingsDTOConverter(entityManager, converterProviderContext);
result.setTransactionPropagations(transactionPropagations);
result.setCache(cache);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy