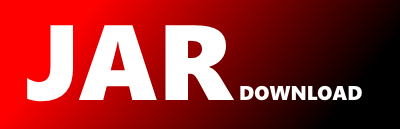
sk.seges.acris.shared.model.dto.ContentDTO Maven / Gradle / Ivy
The newest version!
package sk.seges.acris.shared.model.dto;
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import javax.annotation.Generated;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import sk.seges.acris.core.shared.model.IDataTransferObject;
import sk.seges.acris.node.MenuItem;
import sk.seges.acris.site.shared.domain.api.ContentForUtils;
import sk.seges.sesam.pap.model.annotation.TransferObjectMapping;
@SuppressWarnings("serial")
@TransferObjectMapping(dtoClass = ContentDTO.class,
domainClassName = "sk.seges.acris.domain.server.domain.base.ContentBase",
configurationClassName = "sk.seges.acris.server.model.dto.configuration.ContentDTOConfiguration",
generateConverter = false, generateDto = false)
@Generated(value = "sk.seges.corpis.pap.model.hibernate.HibernateTransferObjectProcessor")
public class ContentDTO implements IDataTransferObject, ContentForUtils, Serializable {
public static final String CONTENT_DETACHED = "contentDetached";
private String contentDetached;
public static final String CREATED = "created";
private Date created;
public static final String DECORATION = "decoration";
private String decoration;
public static final String DEFAULT_STYLE_CLASS = "defaultStyleClass";
private String defaultStyleClass;
public static final String DEFAULTLY_LOADED = "defaultlyLoaded";
private Boolean defaultlyLoaded;
public static final String DESCRIPTION = "description";
private String description;
public static final String GROUP = "group";
private String group;
public static final String HAS_CHILDREN = "hasChildren";
private Boolean hasChildren;
public static final String ID = "id";
private ContentPkDTO id;
public static final String ID_FOR_A_C_L = "idForACL";
private Long idForACL;
public static final String INDEX = "index";
private Integer index;
public static final String KEYWORDS = "keywords";
private String keywords;
public static final String LABEL = "label";
private String label;
public static final String MENU_ITEMS_DATA = "menuItemsData";
private MenuItem menuItemsData;
public static final String MODIFIED = "modified";
private Date modified;
public static final String NICE_URL = "niceUrl";
private String niceUrl;
public static final String PAGE_NAME = "pageName";
private String pageName;
public static final String PARAMS = "params";
private String params;
public static final String PARENT = "parent";
private ContentDTO parent;
public static final String POSITION = "position";
private String position;
public static final String REF = "ref";
private String ref;
public static final String SUB_CONTENTS = "subContents";
private List subContents;
public static final String TITLE = "title";
private String title;
public static final String TOKEN = "token";
private String token;
public static final String VERSION = "version";
private Integer version;
public ContentDTO() {}
public ContentDTO(String contentDetached, Date created, String decoration, String defaultStyleClass, Boolean defaultlyLoaded, String description, String group, Boolean hasChildren, ContentPkDTO id, Long idForACL, Integer index, String keywords, String label, MenuItem menuItemsData, Date modified, String niceUrl, String pageName, String params, ContentDTO parent, String position, String ref, List subContents, String title, String token, Integer version) {
this.contentDetached = contentDetached;
this.created = created;
this.decoration = decoration;
this.defaultStyleClass = defaultStyleClass;
this.defaultlyLoaded = defaultlyLoaded;
this.description = description;
this.group = group;
this.hasChildren = hasChildren;
this.id = id;
this.idForACL = idForACL;
this.index = index;
this.keywords = keywords;
this.label = label;
this.menuItemsData = menuItemsData;
this.modified = modified;
this.niceUrl = niceUrl;
this.pageName = pageName;
this.params = params;
this.parent = parent;
this.position = position;
this.ref = ref;
this.subContents = subContents;
this.title = title;
this.token = token;
this.version = version;
}
public String getContentDetached() {
return contentDetached;
}
public void setContentDetached(String contentDetached) {
this.contentDetached = contentDetached;
}
public Date getCreated() {
return created;
}
public void setCreated(Date created) {
this.created = created;
}
public String getDecoration() {
return decoration;
}
public void setDecoration(String decoration) {
this.decoration = decoration;
}
public String getDefaultStyleClass() {
return defaultStyleClass;
}
public void setDefaultStyleClass(String defaultStyleClass) {
this.defaultStyleClass = defaultStyleClass;
}
public Boolean getDefaultlyLoaded() {
return defaultlyLoaded;
}
public void setDefaultlyLoaded(Boolean defaultlyLoaded) {
this.defaultlyLoaded = defaultlyLoaded;
}
@Size(max = 255, min = 0)
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getGroup() {
return group;
}
public void setGroup(String group) {
this.group = group;
}
public Boolean getHasChildren() {
return hasChildren;
}
public void setHasChildren(Boolean hasChildren) {
this.hasChildren = hasChildren;
}
public ContentPkDTO getId() {
return id;
}
public void setId(ContentPkDTO id) {
this.id = id;
}
public Long getIdForACL() {
return idForACL;
}
public void setIdForACL(Long idForACL) {
this.idForACL = idForACL;
}
public Integer getIndex() {
return index;
}
public void setIndex(Integer index) {
this.index = index;
}
@Size(min = 0, max = 255)
public String getKeywords() {
return keywords;
}
public void setKeywords(String keywords) {
this.keywords = keywords;
}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public MenuItem getMenuItemsData() {
return menuItemsData;
}
public void setMenuItemsData(MenuItem menuItemsData) {
this.menuItemsData = menuItemsData;
}
public Date getModified() {
return modified;
}
public void setModified(Date modified) {
this.modified = modified;
}
@NotNull
@Size(min = 1, max = 255)
@Pattern(regexp = "[a-zA-Z0-9\\-_/]*")
public String getNiceUrl() {
return niceUrl;
}
public void setNiceUrl(String niceUrl) {
this.niceUrl = niceUrl;
}
public String getPageName() {
return pageName;
}
public void setPageName(String pageName) {
this.pageName = pageName;
}
public String getParams() {
return params;
}
public void setParams(String params) {
this.params = params;
}
public ContentDTO getParent() {
return parent;
}
public void setParent(ContentDTO parent) {
this.parent = parent;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
public String getRef() {
return ref;
}
public void setRef(String ref) {
this.ref = ref;
}
public List getSubContents() {
return subContents;
}
public void setSubContents(List subContents) {
this.subContents = subContents;
}
@Size(max = 255, min = 0)
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
private boolean processingEquals = false;
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ContentDTO other = (ContentDTO) obj;
if (!_equalsSupport(contentDetached, other.contentDetached)) return false;
if (!_equalsSupport(created, other.created)) return false;
if (!_equalsSupport(decoration, other.decoration)) return false;
if (!_equalsSupport(defaultStyleClass, other.defaultStyleClass)) return false;
if (!_equalsSupport(defaultlyLoaded, other.defaultlyLoaded)) return false;
if (!_equalsSupport(description, other.description)) return false;
if (!_equalsSupport(group, other.group)) return false;
if (!_equalsSupport(hasChildren, other.hasChildren)) return false;
if (!_equalsSupport(id, other.id)) return false;
if (!_equalsSupport(idForACL, other.idForACL)) return false;
if (!_equalsSupport(index, other.index)) return false;
if (!_equalsSupport(keywords, other.keywords)) return false;
if (!_equalsSupport(label, other.label)) return false;
if (!_equalsSupport(menuItemsData, other.menuItemsData)) return false;
if (!_equalsSupport(modified, other.modified)) return false;
if (!_equalsSupport(niceUrl, other.niceUrl)) return false;
if (!_equalsSupport(pageName, other.pageName)) return false;
if (!_equalsSupport(params, other.params)) return false;
if (!_equalsSupport(parent, other.parent)) return false;
if (!_equalsSupport(position, other.position)) return false;
if (!_equalsSupport(ref, other.ref)) return false;
if (!_equalsSupport(subContents, other.subContents)) return false;
if (!_equalsSupport(title, other.title)) return false;
if (!_equalsSupport(token, other.token)) return false;
if (!_equalsSupport(version, other.version)) return false;
return true;
}
private boolean processingHashCode = false;
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = _hashCodeSupport(contentDetached, prime, result);
result = _hashCodeSupport(created, prime, result);
result = _hashCodeSupport(decoration, prime, result);
result = _hashCodeSupport(defaultStyleClass, prime, result);
result = _hashCodeSupport(defaultlyLoaded, prime, result);
result = _hashCodeSupport(description, prime, result);
result = _hashCodeSupport(group, prime, result);
result = _hashCodeSupport(hasChildren, prime, result);
result = _hashCodeSupport(id, prime, result);
result = _hashCodeSupport(idForACL, prime, result);
result = _hashCodeSupport(index, prime, result);
result = _hashCodeSupport(keywords, prime, result);
result = _hashCodeSupport(label, prime, result);
result = _hashCodeSupport(menuItemsData, prime, result);
result = _hashCodeSupport(modified, prime, result);
result = _hashCodeSupport(niceUrl, prime, result);
result = _hashCodeSupport(pageName, prime, result);
result = _hashCodeSupport(params, prime, result);
result = _hashCodeSupport(parent, prime, result);
result = _hashCodeSupport(position, prime, result);
result = _hashCodeSupport(ref, prime, result);
result = _hashCodeSupport(subContents, prime, result);
result = _hashCodeSupport(title, prime, result);
result = _hashCodeSupport(token, prime, result);
result = _hashCodeSupport(version, prime, result);
return result;
}
private boolean _equalsSupport(Object o1, Object o2) {
if (o1 == null) {
if (o2 != null) return false;
} else if (!processingEquals) {
processingEquals = true;
if (!o1.equals(o2)) return processingEquals = false;
else processingEquals = false;
}
return true;
}
private int _hashCodeSupport(Object o1, int prime, int result) {
if (!processingHashCode) {
processingHashCode = true;
result =
prime * result + ((o1 == null) ? 0 : o1.hashCode());processingHashCode = false;
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy