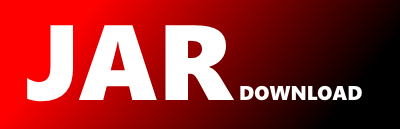
sk.uniq.protobuf.parser.ProtoGenerator Maven / Gradle / Ivy
/*
* Copyright 2016 Jakub Herkel.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package sk.uniq.protobuf.parser;
import sk.uniq.protobuf.parser.impl.DefaultProtoGenerator;
import sk.uniq.protobuf.schema.ProtoFile;
/**
*
* @author jherkel
*/
public class ProtoGenerator {
/**
*
*/
public static final Options PRETTY_PRINT = Options.builder().prettyPrint(true).tabSeparator(4).hideComments(false).build();
/**
*
*/
public static final Options MINIMAL_SIZE = Options.builder().prettyPrint(false).tabSeparator(0).hideComments(true).build();
/**
*
*/
public static class Options {
private final boolean prettyPrint;
private final int tabSeparator;
private final String lineSeparator;
private final String header;
private final boolean hideComments;
private Options(Builder builder) {
if (builder.tabSeparator < 0) {
throw new IllegalArgumentException("Invalid tab separator, tabSeparator:" + builder.tabSeparator);
}
if (builder.lineSeparator == null || builder.lineSeparator.isEmpty() == true) {
throw new IllegalArgumentException("Invalid lineSeparator, lineSeparator is empty");
}
this.prettyPrint = builder.prettyPrint;
this.tabSeparator = builder.tabSeparator;
this.lineSeparator = builder.lineSeparator;
this.header = builder.header;
this.hideComments = builder.hideComments;
}
/**
*
* @return true if pretty print is set
*/
public boolean isPrettyPrint() {
return prettyPrint;
}
/**
*
* @return true if pretty print is set
*/
public int getTabSeparator() {
return tabSeparator;
}
public String getLineSeparator() {
return lineSeparator;
}
public String getHeader() {
return header;
}
public boolean getHideComments() {
return hideComments;
}
/**
*
* @return
*/
public static Builder builder() {
return new Builder();
}
/**
*
*/
public static final class Builder {
private boolean prettyPrint = true;
private int tabSeparator = 4;
private String lineSeparator = System.lineSeparator();
private String header;
private boolean hideComments = false;
private Builder() {
}
/**
*
* @param prettyPrint
* @return
*/
public Builder prettyPrint(boolean prettyPrint) {
this.prettyPrint = prettyPrint;
return this;
}
/**
*
* @param lineSeparator
* @return
*/
public Builder lineSeparator(String lineSeparator) {
this.lineSeparator = lineSeparator;
return this;
}
/**
*
* @param tabSeparator
* @return
*/
public Builder tabSeparator(int tabSeparator) {
this.tabSeparator = tabSeparator;
return this;
}
/**
*
* @param header
* @return
*/
public Builder header(String header) {
this.header = header;
return this;
}
/**
*
* @param hideComments
* @return
*/
public Builder hideComments(boolean hideComments) {
this.hideComments = hideComments;
return this;
}
/**
*
* @return
*/
public Options build() {
return new Options(this);
}
}
}
private ProtoGenerator() {
}
/**
*
* @param protoFile
* @param options
* @return
*/
public static String generate(ProtoFile protoFile, Options options) {
DefaultProtoGenerator generator = new DefaultProtoGenerator(options);
try {
generator.visit(protoFile, null);
} catch (Exception ex) {
throw new IllegalStateException(ex);
}
return generator.getResult();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy