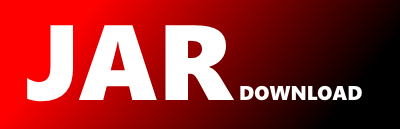
sk.uniq.protobuf.parser.ProtoParserOptions Maven / Gradle / Ivy
/*
* Copyright 2016 Jakub Herkel.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package sk.uniq.protobuf.parser;
/**
*
* @author jherkel
*/
public final class ProtoParserOptions {
public enum ParserCancellationMode {
CANCEL_AFTER_FIRST_ERROR,
TRY_REPORT_ALL_ERRORS
}
/**
*
*/
public static final ProtoParserOptions DEFAULT = builder()
.parseComments(true)
.includeWellKnownTypes(true)
.allowAllias(true)
.parserCancellationMode(ParserCancellationMode.TRY_REPORT_ALL_ERRORS)
.debugOutput(false)
.build();
public static final ProtoParserOptions DEBUG = builder()
.parseComments(true)
.includeWellKnownTypes(true)
.allowAllias(true)
.parserCancellationMode(ParserCancellationMode.TRY_REPORT_ALL_ERRORS)
.debugOutput(true)
.build();
private final boolean parseComments;
private final boolean includeWellKnownTypes;
private final boolean allowAlias;
private final ParserCancellationMode parserCancellationMode;
private final boolean debugOutput;
private ProtoParserOptions(Builder builder) {
this.parseComments = builder.parseComments;
this.includeWellKnownTypes = builder.includeWellKnownTypes;
this.allowAlias = builder.allowAllias;
this.parserCancellationMode = builder.parserCancellationMode;
this.debugOutput = builder.debugOutput;
}
public ParserCancellationMode getParseCancellationMode() {
return parserCancellationMode;
}
/**
*
* @return
*/
public boolean isParseComments() {
return parseComments;
}
/**
*
* @return
*/
public boolean isIncludeWellKnownTypes() {
return includeWellKnownTypes;
}
/**
*
* @return
*/
public boolean isAllowAlias() {
return allowAlias;
}
public ParserCancellationMode getParserCancellationMode() {
return parserCancellationMode;
}
public boolean isDebugOutput() {
return debugOutput;
}
/**
*
* @return
*/
public static Builder builder() {
return new Builder();
}
/**
*
*/
public static final class Builder {
private boolean parseComments = true;
private boolean includeWellKnownTypes = true;
private boolean allowAllias = true;
private ParserCancellationMode parserCancellationMode = ParserCancellationMode.TRY_REPORT_ALL_ERRORS;
private boolean debugOutput = false;
private Builder() {
}
/**
*
* @param parseComments
* @return
*/
public Builder parseComments(boolean parseComments) {
this.parseComments = parseComments;
return this;
}
/**
*
* @param includeWellKnownTypes
* @return
*/
public Builder includeWellKnownTypes(boolean includeWellKnownTypes) {
this.includeWellKnownTypes = includeWellKnownTypes;
return this;
}
/**
*
* @param allowAllias
* @return
*/
public Builder allowAllias(boolean allowAllias) {
this.allowAllias = allowAllias;
return this;
}
/**
*
* @param debugOutput
* @return
*/
public Builder debugOutput(boolean debugOutput) {
this.debugOutput = debugOutput;
return this;
}
/**
*
* @param parserCancellationMode
* @return
*/
public Builder parserCancellationMode(ParserCancellationMode parserCancellationMode) {
this.parserCancellationMode = parserCancellationMode;
return this;
}
/**
*
* @return
*/
public ProtoParserOptions build() {
return new ProtoParserOptions(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy