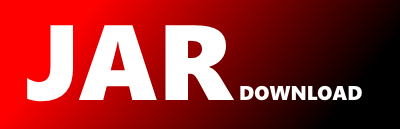
sk.uniq.protobuf.schema.ProtoSchemaVisitor Maven / Gradle / Ivy
/*
* Copyright 2016 Jakub Herkel.
*
* Licensed under the Apache License, Version 2.0 (the "License",T context);
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package sk.uniq.protobuf.schema;
/**
*
* @author jherkel
*/
public interface ProtoSchemaVisitor {
public void visit(ProtoFile file, T context) throws Exception;
public void visit(ProtoVersion version, T context) throws Exception;
public void visit(ProtoPackage packagePB, T context) throws Exception;
public void visit(ProtoEnum enumPB, T context) throws Exception;
public void visit(ProtoEnumField enumField, T context) throws Exception;
public void visit(ProtoIdentifier identifier, T context) throws Exception;
public void visit(ProtoImport importPB, T context) throws Exception;
public void visit(ProtoMap map, T context) throws Exception;
public void visit(ProtoMessage message, T context) throws Exception;
public void visit(ProtoMessageField messageField, T context) throws Exception;
public void visit(ProtoOption option, T context) throws Exception;
public void visit(ProtoOneOf oneof, T context) throws Exception;
public void visit(ProtoOneOfField oneOfField, T context) throws Exception;
public void visit(ProtoReserved reserved, T context) throws Exception;
public void visit(ProtoRpc rpc, T context) throws Exception;
public void visit(ProtoService service, T context) throws Exception;
static void visitInternal(ProtoSchemaVisitor visitor, ProtoSyntaxElement element, T context) throws Exception {
switch (element.getType()) {
case ENUM:
visitor.visit((ProtoEnum) element, context);
break;
case ENUM_FIELD:
visitor.visit((ProtoEnumField) element, context);
break;
case IDENTIFIER:
visitor.visit((ProtoIdentifier) element, context);
break;
case IMPORT:
visitor.visit((ProtoOption) element, context);
break;
case MAP:
visitor.visit((ProtoMap) element, context);
break;
case MESSAGE:
visitor.visit((ProtoMessage) element, context);
break;
case MESSAGE_FIELD:
visitor.visit((ProtoMessageField) element, context);
break;
case ONEOF:
visitor.visit((ProtoOneOf) element, context);
break;
case ONEOF_FIELD:
visitor.visit((ProtoOneOfField) element, context);
break;
case OPTION:
visitor.visit((ProtoOption) element, context);
break;
case PACKAGE:
visitor.visit((ProtoPackage) element, context);
break;
case PROTO:
visitor.visit((ProtoFile) element, context);
break;
case RESERVED:
visitor.visit((ProtoReserved) element, context);
break;
case RPC:
visitor.visit((ProtoRpc) element, context);
break;
case SERVICE:
visitor.visit((ProtoService) element, context);
break;
case VERSION:
visitor.visit((ProtoVersion) element, context);
break;
default:
throw new IllegalStateException("Unknown type:" + element.getType());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy