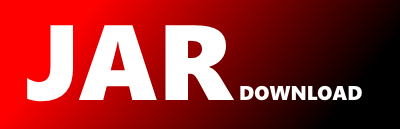
software.amazon.awscdk.services.appconfig.CfnDeployment Maven / Gradle / Ivy
package software.amazon.awscdk.services.appconfig;
/**
* A CloudFormation `AWS::AppConfig::Deployment`.
*
* The AWS::AppConfig::Deployment
resource starts a deployment. Starting a deployment in AWS AppConfig calls the StartDeployment
API action. This call includes the IDs of the AWS AppConfig application, the environment, the configuration profile, and (optionally) the configuration data version to deploy. The call also includes the ID of the deployment strategy to use, which determines how the configuration data is deployed.
*
* AWS AppConfig monitors the distribution to all hosts and reports status. If a distribution fails, then AWS AppConfig rolls back the configuration.
*
* AWS AppConfig requires that you create resources and deploy a configuration in the following order:
*
*
* - Create an application
* - Create an environment
* - Create a configuration profile
* - Create a deployment strategy
* - Deploy the configuration
*
*
* For more information, see AWS AppConfig in the AWS AppConfig User Guide .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appconfig.*;
* CfnDeployment cfnDeployment = CfnDeployment.Builder.create(this, "MyCfnDeployment")
* .applicationId("applicationId")
* .configurationProfileId("configurationProfileId")
* .configurationVersion("configurationVersion")
* .deploymentStrategyId("deploymentStrategyId")
* .environmentId("environmentId")
* // the properties below are optional
* .description("description")
* .tags(List.of(TagsProperty.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.50.0 (build d1830a4)", date = "2022-01-09T19:25:18.535Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appconfig.$Module.class, fqn = "@aws-cdk/aws-appconfig.CfnDeployment")
public class CfnDeployment extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnDeployment(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnDeployment(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.appconfig.CfnDeployment.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::AppConfig::Deployment`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnDeployment(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appconfig.CfnDeploymentProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The application ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getApplicationId() {
return software.amazon.jsii.Kernel.get(this, "applicationId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The application ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setApplicationId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "applicationId", java.util.Objects.requireNonNull(value, "applicationId is required"));
}
/**
* The configuration profile ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getConfigurationProfileId() {
return software.amazon.jsii.Kernel.get(this, "configurationProfileId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The configuration profile ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setConfigurationProfileId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "configurationProfileId", java.util.Objects.requireNonNull(value, "configurationProfileId is required"));
}
/**
* The configuration version to deploy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getConfigurationVersion() {
return software.amazon.jsii.Kernel.get(this, "configurationVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The configuration version to deploy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setConfigurationVersion(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "configurationVersion", java.util.Objects.requireNonNull(value, "configurationVersion is required"));
}
/**
* The deployment strategy ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getDeploymentStrategyId() {
return software.amazon.jsii.Kernel.get(this, "deploymentStrategyId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The deployment strategy ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDeploymentStrategyId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "deploymentStrategyId", java.util.Objects.requireNonNull(value, "deploymentStrategyId is required"));
}
/**
* The environment ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getEnvironmentId() {
return software.amazon.jsii.Kernel.get(this, "environmentId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The environment ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEnvironmentId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "environmentId", java.util.Objects.requireNonNull(value, "environmentId is required"));
}
/**
* A description of the deployment.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A description of the deployment.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* Metadata to assign to the deployment.
*
* Tags help organize and categorize your AWS AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.util.List getTags() {
return java.util.Optional.ofNullable((java.util.List)(software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.appconfig.CfnDeployment.TagsProperty.class))))).map(java.util.Collections::unmodifiableList).orElse(null);
}
/**
* Metadata to assign to the deployment.
*
* Tags help organize and categorize your AWS AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setTags(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "tags", value);
}
/**
* Metadata to assign to the deployment strategy.
*
* Tags help organize and categorize your AWS AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appconfig.*;
* TagsProperty tagsProperty = TagsProperty.builder()
* .key("key")
* .value("value")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appconfig.$Module.class, fqn = "@aws-cdk/aws-appconfig.CfnDeployment.TagsProperty")
@software.amazon.jsii.Jsii.Proxy(TagsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface TagsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The key-value string map.
*
* The valid character set is [a-zA-Z+-=._:/]
. The tag key can be up to 128 characters and must not start with aws:
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getKey() {
return null;
}
/**
* The tag value can be up to 256 characters.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getValue() {
return null;
}
/**
* @return a {@link Builder} of {@link TagsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TagsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String key;
java.lang.String value;
/**
* Sets the value of {@link TagsProperty#getKey}
* @param key The key-value string map.
* The valid character set is [a-zA-Z+-=._:/]
. The tag key can be up to 128 characters and must not start with aws:
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder key(java.lang.String key) {
this.key = key;
return this;
}
/**
* Sets the value of {@link TagsProperty#getValue}
* @param value The tag value can be up to 256 characters.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.String value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TagsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public TagsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link TagsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements TagsProperty {
private final java.lang.String key;
private final java.lang.String value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.key = software.amazon.jsii.Kernel.get(this, "key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.key = builder.key;
this.value = builder.value;
}
@Override
public final java.lang.String getKey() {
return this.key;
}
@Override
public final java.lang.String getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getKey() != null) {
data.set("key", om.valueToTree(this.getKey()));
}
if (this.getValue() != null) {
data.set("value", om.valueToTree(this.getValue()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appconfig.CfnDeployment.TagsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TagsProperty.Jsii$Proxy that = (TagsProperty.Jsii$Proxy) o;
if (this.key != null ? !this.key.equals(that.key) : that.key != null) return false;
return this.value != null ? this.value.equals(that.value) : that.value == null;
}
@Override
public final int hashCode() {
int result = this.key != null ? this.key.hashCode() : 0;
result = 31 * result + (this.value != null ? this.value.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.appconfig.CfnDeployment}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.appconfig.CfnDeploymentProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.appconfig.CfnDeploymentProps.Builder();
}
/**
* The application ID.
*
* @return {@code this}
* @param applicationId The application ID. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder applicationId(final java.lang.String applicationId) {
this.props.applicationId(applicationId);
return this;
}
/**
* The configuration profile ID.
*
* @return {@code this}
* @param configurationProfileId The configuration profile ID. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder configurationProfileId(final java.lang.String configurationProfileId) {
this.props.configurationProfileId(configurationProfileId);
return this;
}
/**
* The configuration version to deploy.
*
* @return {@code this}
* @param configurationVersion The configuration version to deploy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder configurationVersion(final java.lang.String configurationVersion) {
this.props.configurationVersion(configurationVersion);
return this;
}
/**
* The deployment strategy ID.
*
* @return {@code this}
* @param deploymentStrategyId The deployment strategy ID. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentStrategyId(final java.lang.String deploymentStrategyId) {
this.props.deploymentStrategyId(deploymentStrategyId);
return this;
}
/**
* The environment ID.
*
* @return {@code this}
* @param environmentId The environment ID. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environmentId(final java.lang.String environmentId) {
this.props.environmentId(environmentId);
return this;
}
/**
* A description of the deployment.
*
* @return {@code this}
* @param description A description of the deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* Metadata to assign to the deployment.
*
* Tags help organize and categorize your AWS AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*
* @return {@code this}
* @param tags Metadata to assign to the deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.services.appconfig.CfnDeployment.TagsProperty> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.appconfig.CfnDeployment}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.appconfig.CfnDeployment build() {
return new software.amazon.awscdk.services.appconfig.CfnDeployment(
this.scope,
this.id,
this.props.build()
);
}
}
}