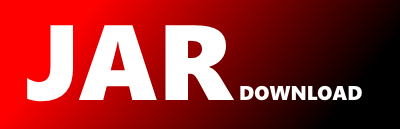
software.amazon.awscdk.services.apprunner.alpha.Service Maven / Gradle / Ivy
Show all versions of apprunner-alpha Show documentation
package software.amazon.awscdk.services.apprunner.alpha;
/**
* (experimental) The App Runner Service.
*
* Example:
*
*
* import software.amazon.awscdk.services.iam.*;
* Service service = Service.Builder.create(this, "Service")
* .source(Source.fromEcrPublic(EcrPublicProps.builder()
* .imageConfiguration(ImageConfiguration.builder().port(8000).build())
* .imageIdentifier("public.ecr.aws/aws-containers/hello-app-runner:latest")
* .build()))
* .build();
* service.addToRolePolicy(PolicyStatement.Builder.create()
* .effect(Effect.ALLOW)
* .actions(List.of("s3:GetObject"))
* .resources(List.of("*"))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-17T21:37:41.241Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.apprunner.alpha.$Module.class, fqn = "@aws-cdk/aws-apprunner-alpha.Service")
public class Service extends software.amazon.awscdk.Resource implements software.amazon.awscdk.services.iam.IGrantable {
protected Service(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Service(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Service(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.apprunner.alpha.ServiceProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import from service attributes.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param attrs This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.apprunner.alpha.IService fromServiceAttributes(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.apprunner.alpha.ServiceAttributes attrs) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.apprunner.alpha.Service.class, "fromServiceAttributes", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apprunner.alpha.IService.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(attrs, "attrs is required") });
}
/**
* (experimental) Import from service name.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param serviceName This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.apprunner.alpha.IService fromServiceName(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String serviceName) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.apprunner.alpha.Service.class, "fromServiceName", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.apprunner.alpha.IService.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(serviceName, "serviceName is required") });
}
/**
* (experimental) This method adds an environment variable to the App Runner service.
*
* @param name This parameter is required.
* @param value This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addEnvironmentVariable(final @org.jetbrains.annotations.NotNull java.lang.String name, final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.call(this, "addEnvironmentVariable", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(name, "name is required"), java.util.Objects.requireNonNull(value, "value is required") });
}
/**
* (experimental) This method adds a secret as environment variable to the App Runner service.
*
* @param name This parameter is required.
* @param secret This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addSecret(final @org.jetbrains.annotations.NotNull java.lang.String name, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.apprunner.alpha.Secret secret) {
software.amazon.jsii.Kernel.call(this, "addSecret", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(name, "name is required"), java.util.Objects.requireNonNull(secret, "secret is required") });
}
/**
* (experimental) Adds a statement to the instance role.
*
* @param statement This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addToRolePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement) {
software.amazon.jsii.Kernel.call(this, "addToRolePolicy", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(statement, "statement is required") });
}
/**
* (deprecated) Environment variables for this service.
*
* @deprecated use environmentVariables.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public @org.jetbrains.annotations.NotNull java.util.Map getEnvironment() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "environment", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* (experimental) The principal to grant permissions to.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IPrincipal getGrantPrincipal() {
return software.amazon.jsii.Kernel.get(this, "grantPrincipal", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IPrincipal.class));
}
/**
* (experimental) The ARN of the Service.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getServiceArn() {
return software.amazon.jsii.Kernel.get(this, "serviceArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The ID of the Service.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getServiceId() {
return software.amazon.jsii.Kernel.get(this, "serviceId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The name of the service.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getServiceName() {
return software.amazon.jsii.Kernel.get(this, "serviceName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The status of the Service.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getServiceStatus() {
return software.amazon.jsii.Kernel.get(this, "serviceStatus", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The URL of the Service.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getServiceUrl() {
return software.amazon.jsii.Kernel.get(this, "serviceUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.apprunner.alpha.Service}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.apprunner.alpha.ServiceProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.apprunner.alpha.ServiceProps.Builder();
}
/**
* (experimental) The source of the repository for the service.
*
* @return {@code this}
* @param source The source of the repository for the service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder source(final software.amazon.awscdk.services.apprunner.alpha.Source source) {
this.props.source(source);
return this;
}
/**
* (experimental) The IAM role that grants the App Runner service access to a source repository.
*
* It's required for ECR image repositories (but not for ECR Public repositories).
*
* The role must be assumable by the 'build.apprunner.amazonaws.com' service principal.
*
* Default: - generate a new access role.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/apprunner/latest/dg/security_iam_service-with-iam.html#security_iam_service-with-iam-roles-service.access
* @param accessRole The IAM role that grants the App Runner service access to a source repository. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder accessRole(final software.amazon.awscdk.services.iam.IRole accessRole) {
this.props.accessRole(accessRole);
return this;
}
/**
* (experimental) Specifies whether to enable continuous integration from the source repository.
*
* If true, continuous integration from the source repository is enabled for the App Runner service.
* Each repository change (including any source code commit or new image version) starts a deployment.
* By default, App Runner sets to false for a source image that uses an ECR Public repository or an ECR repository that's in an AWS account other than the one that the service is in.
* App Runner sets to true in all other cases (which currently include a source code repository or a source image using a same-account ECR repository).
*
* Default: - no value will be passed.
*
* @return {@code this}
* @param autoDeploymentsEnabled Specifies whether to enable continuous integration from the source repository. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder autoDeploymentsEnabled(final java.lang.Boolean autoDeploymentsEnabled) {
this.props.autoDeploymentsEnabled(autoDeploymentsEnabled);
return this;
}
/**
* (experimental) Specifies an App Runner Auto Scaling Configuration.
*
* A default configuration is either the AWS recommended configuration,
* or the configuration you set as the default.
*
* Default: - the latest revision of a default auto scaling configuration is used.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/apprunner/latest/dg/manage-autoscaling.html
* @param autoScalingConfiguration Specifies an App Runner Auto Scaling Configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder autoScalingConfiguration(final software.amazon.awscdk.services.apprunner.alpha.IAutoScalingConfiguration autoScalingConfiguration) {
this.props.autoScalingConfiguration(autoScalingConfiguration);
return this;
}
/**
* (experimental) The number of CPU units reserved for each instance of your App Runner service.
*
* Default: Cpu.ONE_VCPU
*
* @return {@code this}
* @param cpu The number of CPU units reserved for each instance of your App Runner service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder cpu(final software.amazon.awscdk.services.apprunner.alpha.Cpu cpu) {
this.props.cpu(cpu);
return this;
}
/**
* (experimental) Settings for the health check that AWS App Runner performs to monitor the health of a service.
*
* You can specify it by static methods HealthCheck.http
or HealthCheck.tcp
.
*
* Default: - no health check configuration
*
* @return {@code this}
* @param healthCheck Settings for the health check that AWS App Runner performs to monitor the health of a service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder healthCheck(final software.amazon.awscdk.services.apprunner.alpha.HealthCheck healthCheck) {
this.props.healthCheck(healthCheck);
return this;
}
/**
* (experimental) The IAM role that provides permissions to your App Runner service.
*
* These are permissions that your code needs when it calls any AWS APIs.
*
* The role must be assumable by the 'tasks.apprunner.amazonaws.com' service principal.
*
* Default: - generate a new instance role.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/apprunner/latest/dg/security_iam_service-with-iam.html#security_iam_service-with-iam-roles-service.instance
* @param instanceRole The IAM role that provides permissions to your App Runner service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instanceRole(final software.amazon.awscdk.services.iam.IRole instanceRole) {
this.props.instanceRole(instanceRole);
return this;
}
/**
* (experimental) The IP address type for your incoming public network configuration.
*
* Default: - IpAddressType.IPV4
*
* @return {@code this}
* @param ipAddressType The IP address type for your incoming public network configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipAddressType(final software.amazon.awscdk.services.apprunner.alpha.IpAddressType ipAddressType) {
this.props.ipAddressType(ipAddressType);
return this;
}
/**
* (experimental) Specifies whether your App Runner service is publicly accessible.
*
* If you use VpcIngressConnection
, you must set this property to false
.
*
* Default: true
*
* @return {@code this}
* @param isPubliclyAccessible Specifies whether your App Runner service is publicly accessible. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder isPubliclyAccessible(final java.lang.Boolean isPubliclyAccessible) {
this.props.isPubliclyAccessible(isPubliclyAccessible);
return this;
}
/**
* (experimental) The customer managed key that AWS App Runner uses to encrypt copies of the source repository and service logs.
*
* Default: - Use an AWS managed key
*
* @return {@code this}
* @param kmsKey The customer managed key that AWS App Runner uses to encrypt copies of the source repository and service logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder kmsKey(final software.amazon.awscdk.services.kms.IKey kmsKey) {
this.props.kmsKey(kmsKey);
return this;
}
/**
* (experimental) The amount of memory reserved for each instance of your App Runner service.
*
* Default: Memory.TWO_GB
*
* @return {@code this}
* @param memory The amount of memory reserved for each instance of your App Runner service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder memory(final software.amazon.awscdk.services.apprunner.alpha.Memory memory) {
this.props.memory(memory);
return this;
}
/**
* (experimental) Settings for an App Runner observability configuration.
*
* Default: - no observability configuration resource is associated with the service.
*
* @return {@code this}
* @param observabilityConfiguration Settings for an App Runner observability configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder observabilityConfiguration(final software.amazon.awscdk.services.apprunner.alpha.IObservabilityConfiguration observabilityConfiguration) {
this.props.observabilityConfiguration(observabilityConfiguration);
return this;
}
/**
* (experimental) Name of the service.
*
* Default: - auto-generated if undefined.
*
* @return {@code this}
* @param serviceName Name of the service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder serviceName(final java.lang.String serviceName) {
this.props.serviceName(serviceName);
return this;
}
/**
* (experimental) Settings for an App Runner VPC connector to associate with the service.
*
* Default: - no VPC connector, uses the DEFAULT egress type instead
*
* @return {@code this}
* @param vpcConnector Settings for an App Runner VPC connector to associate with the service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcConnector(final software.amazon.awscdk.services.apprunner.alpha.IVpcConnector vpcConnector) {
this.props.vpcConnector(vpcConnector);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.apprunner.alpha.Service}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.apprunner.alpha.Service build() {
return new software.amazon.awscdk.services.apprunner.alpha.Service(
this.scope,
this.id,
this.props.build()
);
}
}
}