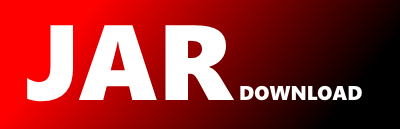
software.amazon.awscdk.services.appstream.CfnUserProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream Show documentation
Show all versions of appstream Show documentation
The CDK Construct Library for AWS::AppStream
package software.amazon.awscdk.services.appstream;
/**
* Properties for defining a `AWS::AppStream::User`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.7.15 (build e429c41)", date = "2019-03-20T12:53:22.293Z")
public interface CfnUserProps extends software.amazon.jsii.JsiiSerializable {
/**
* `AWS::AppStream::User.AuthenticationType`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-authenticationtype
*/
java.lang.String getAuthenticationType();
/**
* `AWS::AppStream::User.AuthenticationType`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-authenticationtype
*/
void setAuthenticationType(final java.lang.String value);
/**
* `AWS::AppStream::User.UserName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-username
*/
java.lang.String getUserName();
/**
* `AWS::AppStream::User.UserName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-username
*/
void setUserName(final java.lang.String value);
/**
* `AWS::AppStream::User.FirstName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-firstname
*/
java.lang.String getFirstName();
/**
* `AWS::AppStream::User.FirstName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-firstname
*/
void setFirstName(final java.lang.String value);
/**
* `AWS::AppStream::User.LastName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-lastname
*/
java.lang.String getLastName();
/**
* `AWS::AppStream::User.LastName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-lastname
*/
void setLastName(final java.lang.String value);
/**
* `AWS::AppStream::User.MessageAction`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-messageaction
*/
java.lang.String getMessageAction();
/**
* `AWS::AppStream::User.MessageAction`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-messageaction
*/
void setMessageAction(final java.lang.String value);
/**
* @return a {@link Builder} of {@link CfnUserProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnUserProps}
*/
final class Builder {
private java.lang.String _authenticationType;
private java.lang.String _userName;
@javax.annotation.Nullable
private java.lang.String _firstName;
@javax.annotation.Nullable
private java.lang.String _lastName;
@javax.annotation.Nullable
private java.lang.String _messageAction;
/**
* Sets the value of AuthenticationType
* @param value `AWS::AppStream::User.AuthenticationType`
* @return {@code this}
*/
public Builder withAuthenticationType(final java.lang.String value) {
this._authenticationType = java.util.Objects.requireNonNull(value, "authenticationType is required");
return this;
}
/**
* Sets the value of UserName
* @param value `AWS::AppStream::User.UserName`
* @return {@code this}
*/
public Builder withUserName(final java.lang.String value) {
this._userName = java.util.Objects.requireNonNull(value, "userName is required");
return this;
}
/**
* Sets the value of FirstName
* @param value `AWS::AppStream::User.FirstName`
* @return {@code this}
*/
public Builder withFirstName(@javax.annotation.Nullable final java.lang.String value) {
this._firstName = value;
return this;
}
/**
* Sets the value of LastName
* @param value `AWS::AppStream::User.LastName`
* @return {@code this}
*/
public Builder withLastName(@javax.annotation.Nullable final java.lang.String value) {
this._lastName = value;
return this;
}
/**
* Sets the value of MessageAction
* @param value `AWS::AppStream::User.MessageAction`
* @return {@code this}
*/
public Builder withMessageAction(@javax.annotation.Nullable final java.lang.String value) {
this._messageAction = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnUserProps}
* @throws NullPointerException if any required attribute was not provided
*/
public CfnUserProps build() {
return new CfnUserProps() {
private java.lang.String $authenticationType = java.util.Objects.requireNonNull(_authenticationType, "authenticationType is required");
private java.lang.String $userName = java.util.Objects.requireNonNull(_userName, "userName is required");
@javax.annotation.Nullable
private java.lang.String $firstName = _firstName;
@javax.annotation.Nullable
private java.lang.String $lastName = _lastName;
@javax.annotation.Nullable
private java.lang.String $messageAction = _messageAction;
@Override
public java.lang.String getAuthenticationType() {
return this.$authenticationType;
}
@Override
public void setAuthenticationType(final java.lang.String value) {
this.$authenticationType = java.util.Objects.requireNonNull(value, "authenticationType is required");
}
@Override
public java.lang.String getUserName() {
return this.$userName;
}
@Override
public void setUserName(final java.lang.String value) {
this.$userName = java.util.Objects.requireNonNull(value, "userName is required");
}
@Override
public java.lang.String getFirstName() {
return this.$firstName;
}
@Override
public void setFirstName(@javax.annotation.Nullable final java.lang.String value) {
this.$firstName = value;
}
@Override
public java.lang.String getLastName() {
return this.$lastName;
}
@Override
public void setLastName(@javax.annotation.Nullable final java.lang.String value) {
this.$lastName = value;
}
@Override
public java.lang.String getMessageAction() {
return this.$messageAction;
}
@Override
public void setMessageAction(@javax.annotation.Nullable final java.lang.String value) {
this.$messageAction = value;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.appstream.CfnUserProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* `AWS::AppStream::User.AuthenticationType`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-authenticationtype
*/
@Override
public java.lang.String getAuthenticationType() {
return this.jsiiGet("authenticationType", java.lang.String.class);
}
/**
* `AWS::AppStream::User.AuthenticationType`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-authenticationtype
*/
@Override
public void setAuthenticationType(final java.lang.String value) {
this.jsiiSet("authenticationType", java.util.Objects.requireNonNull(value, "authenticationType is required"));
}
/**
* `AWS::AppStream::User.UserName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-username
*/
@Override
public java.lang.String getUserName() {
return this.jsiiGet("userName", java.lang.String.class);
}
/**
* `AWS::AppStream::User.UserName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-username
*/
@Override
public void setUserName(final java.lang.String value) {
this.jsiiSet("userName", java.util.Objects.requireNonNull(value, "userName is required"));
}
/**
* `AWS::AppStream::User.FirstName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-firstname
*/
@Override
@javax.annotation.Nullable
public java.lang.String getFirstName() {
return this.jsiiGet("firstName", java.lang.String.class);
}
/**
* `AWS::AppStream::User.FirstName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-firstname
*/
@Override
public void setFirstName(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("firstName", value);
}
/**
* `AWS::AppStream::User.LastName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-lastname
*/
@Override
@javax.annotation.Nullable
public java.lang.String getLastName() {
return this.jsiiGet("lastName", java.lang.String.class);
}
/**
* `AWS::AppStream::User.LastName`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-lastname
*/
@Override
public void setLastName(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("lastName", value);
}
/**
* `AWS::AppStream::User.MessageAction`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-messageaction
*/
@Override
@javax.annotation.Nullable
public java.lang.String getMessageAction() {
return this.jsiiGet("messageAction", java.lang.String.class);
}
/**
* `AWS::AppStream::User.MessageAction`
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-appstream-user.html#cfn-appstream-user-messageaction
*/
@Override
public void setMessageAction(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("messageAction", value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy