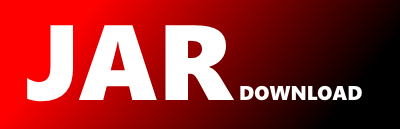
software.amazon.awscdk.services.appstream.CfnApplicationProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appstream Show documentation
Show all versions of appstream Show documentation
The CDK Construct Library for AWS::AppStream
package software.amazon.awscdk.services.appstream;
/**
* Properties for defining a `AWS::AppStream::Application`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnApplicationProps cfnApplicationProps = CfnApplicationProps.builder()
* .appBlockArn("appBlockArn")
* .iconS3Location(S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build())
* .instanceFamilies(List.of("instanceFamilies"))
* .launchPath("launchPath")
* .name("name")
* .platforms(List.of("platforms"))
* // the properties below are optional
* .attributesToDelete(List.of("attributesToDelete"))
* .description("description")
* .displayName("displayName")
* .launchParameters("launchParameters")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .workingDirectory("workingDirectory")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.47.0 (build 86d2c33)", date = "2021-12-10T18:01:58.701Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnApplicationProps")
@software.amazon.jsii.Jsii.Proxy(CfnApplicationProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnApplicationProps extends software.amazon.jsii.JsiiSerializable {
/**
* `AWS::AppStream::Application.AppBlockArn`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAppBlockArn();
/**
* `AWS::AppStream::Application.AttributesToDelete`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getAttributesToDelete() {
return null;
}
/**
* `AWS::AppStream::Application.Description`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* `AWS::AppStream::Application.DisplayName`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDisplayName() {
return null;
}
/**
* `AWS::AppStream::Application.IconS3Location`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getIconS3Location();
/**
* `AWS::AppStream::Application.InstanceFamilies`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getInstanceFamilies();
/**
* `AWS::AppStream::Application.LaunchParameters`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getLaunchParameters() {
return null;
}
/**
* `AWS::AppStream::Application.LaunchPath`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getLaunchPath();
/**
* `AWS::AppStream::Application.Name`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* `AWS::AppStream::Application.Platforms`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getPlatforms();
/**
* `AWS::AppStream::Application.Tags`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* `AWS::AppStream::Application.WorkingDirectory`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getWorkingDirectory() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnApplicationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnApplicationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String appBlockArn;
java.util.List attributesToDelete;
java.lang.String description;
java.lang.String displayName;
java.lang.Object iconS3Location;
java.util.List instanceFamilies;
java.lang.String launchParameters;
java.lang.String launchPath;
java.lang.String name;
java.util.List platforms;
java.util.List tags;
java.lang.String workingDirectory;
/**
* Sets the value of {@link CfnApplicationProps#getAppBlockArn}
* @param appBlockArn `AWS::AppStream::Application.AppBlockArn`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appBlockArn(java.lang.String appBlockArn) {
this.appBlockArn = appBlockArn;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getAttributesToDelete}
* @param attributesToDelete `AWS::AppStream::Application.AttributesToDelete`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder attributesToDelete(java.util.List attributesToDelete) {
this.attributesToDelete = attributesToDelete;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getDescription}
* @param description `AWS::AppStream::Application.Description`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getDisplayName}
* @param displayName `AWS::AppStream::Application.DisplayName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder displayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getIconS3Location}
* @param iconS3Location `AWS::AppStream::Application.IconS3Location`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iconS3Location(software.amazon.awscdk.core.IResolvable iconS3Location) {
this.iconS3Location = iconS3Location;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getIconS3Location}
* @param iconS3Location `AWS::AppStream::Application.IconS3Location`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iconS3Location(software.amazon.awscdk.services.appstream.CfnApplication.S3LocationProperty iconS3Location) {
this.iconS3Location = iconS3Location;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getInstanceFamilies}
* @param instanceFamilies `AWS::AppStream::Application.InstanceFamilies`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceFamilies(java.util.List instanceFamilies) {
this.instanceFamilies = instanceFamilies;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getLaunchParameters}
* @param launchParameters `AWS::AppStream::Application.LaunchParameters`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder launchParameters(java.lang.String launchParameters) {
this.launchParameters = launchParameters;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getLaunchPath}
* @param launchPath `AWS::AppStream::Application.LaunchPath`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder launchPath(java.lang.String launchPath) {
this.launchPath = launchPath;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getName}
* @param name `AWS::AppStream::Application.Name`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getPlatforms}
* @param platforms `AWS::AppStream::Application.Platforms`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder platforms(java.util.List platforms) {
this.platforms = platforms;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getTags}
* @param tags `AWS::AppStream::Application.Tags`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnApplicationProps#getWorkingDirectory}
* @param workingDirectory `AWS::AppStream::Application.WorkingDirectory`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workingDirectory(java.lang.String workingDirectory) {
this.workingDirectory = workingDirectory;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnApplicationProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnApplicationProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnApplicationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnApplicationProps {
private final java.lang.String appBlockArn;
private final java.util.List attributesToDelete;
private final java.lang.String description;
private final java.lang.String displayName;
private final java.lang.Object iconS3Location;
private final java.util.List instanceFamilies;
private final java.lang.String launchParameters;
private final java.lang.String launchPath;
private final java.lang.String name;
private final java.util.List platforms;
private final java.util.List tags;
private final java.lang.String workingDirectory;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.appBlockArn = software.amazon.jsii.Kernel.get(this, "appBlockArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.attributesToDelete = software.amazon.jsii.Kernel.get(this, "attributesToDelete", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.displayName = software.amazon.jsii.Kernel.get(this, "displayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.iconS3Location = software.amazon.jsii.Kernel.get(this, "iconS3Location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.instanceFamilies = software.amazon.jsii.Kernel.get(this, "instanceFamilies", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.launchParameters = software.amazon.jsii.Kernel.get(this, "launchParameters", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.launchPath = software.amazon.jsii.Kernel.get(this, "launchPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.platforms = software.amazon.jsii.Kernel.get(this, "platforms", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
this.workingDirectory = software.amazon.jsii.Kernel.get(this, "workingDirectory", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.appBlockArn = java.util.Objects.requireNonNull(builder.appBlockArn, "appBlockArn is required");
this.attributesToDelete = builder.attributesToDelete;
this.description = builder.description;
this.displayName = builder.displayName;
this.iconS3Location = java.util.Objects.requireNonNull(builder.iconS3Location, "iconS3Location is required");
this.instanceFamilies = java.util.Objects.requireNonNull(builder.instanceFamilies, "instanceFamilies is required");
this.launchParameters = builder.launchParameters;
this.launchPath = java.util.Objects.requireNonNull(builder.launchPath, "launchPath is required");
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.platforms = java.util.Objects.requireNonNull(builder.platforms, "platforms is required");
this.tags = (java.util.List)builder.tags;
this.workingDirectory = builder.workingDirectory;
}
@Override
public final java.lang.String getAppBlockArn() {
return this.appBlockArn;
}
@Override
public final java.util.List getAttributesToDelete() {
return this.attributesToDelete;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.String getDisplayName() {
return this.displayName;
}
@Override
public final java.lang.Object getIconS3Location() {
return this.iconS3Location;
}
@Override
public final java.util.List getInstanceFamilies() {
return this.instanceFamilies;
}
@Override
public final java.lang.String getLaunchParameters() {
return this.launchParameters;
}
@Override
public final java.lang.String getLaunchPath() {
return this.launchPath;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.util.List getPlatforms() {
return this.platforms;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.lang.String getWorkingDirectory() {
return this.workingDirectory;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("appBlockArn", om.valueToTree(this.getAppBlockArn()));
if (this.getAttributesToDelete() != null) {
data.set("attributesToDelete", om.valueToTree(this.getAttributesToDelete()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getDisplayName() != null) {
data.set("displayName", om.valueToTree(this.getDisplayName()));
}
data.set("iconS3Location", om.valueToTree(this.getIconS3Location()));
data.set("instanceFamilies", om.valueToTree(this.getInstanceFamilies()));
if (this.getLaunchParameters() != null) {
data.set("launchParameters", om.valueToTree(this.getLaunchParameters()));
}
data.set("launchPath", om.valueToTree(this.getLaunchPath()));
data.set("name", om.valueToTree(this.getName()));
data.set("platforms", om.valueToTree(this.getPlatforms()));
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getWorkingDirectory() != null) {
data.set("workingDirectory", om.valueToTree(this.getWorkingDirectory()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appstream.CfnApplicationProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnApplicationProps.Jsii$Proxy that = (CfnApplicationProps.Jsii$Proxy) o;
if (!appBlockArn.equals(that.appBlockArn)) return false;
if (this.attributesToDelete != null ? !this.attributesToDelete.equals(that.attributesToDelete) : that.attributesToDelete != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.displayName != null ? !this.displayName.equals(that.displayName) : that.displayName != null) return false;
if (!iconS3Location.equals(that.iconS3Location)) return false;
if (!instanceFamilies.equals(that.instanceFamilies)) return false;
if (this.launchParameters != null ? !this.launchParameters.equals(that.launchParameters) : that.launchParameters != null) return false;
if (!launchPath.equals(that.launchPath)) return false;
if (!name.equals(that.name)) return false;
if (!platforms.equals(that.platforms)) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
return this.workingDirectory != null ? this.workingDirectory.equals(that.workingDirectory) : that.workingDirectory == null;
}
@Override
public final int hashCode() {
int result = this.appBlockArn.hashCode();
result = 31 * result + (this.attributesToDelete != null ? this.attributesToDelete.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.displayName != null ? this.displayName.hashCode() : 0);
result = 31 * result + (this.iconS3Location.hashCode());
result = 31 * result + (this.instanceFamilies.hashCode());
result = 31 * result + (this.launchParameters != null ? this.launchParameters.hashCode() : 0);
result = 31 * result + (this.launchPath.hashCode());
result = 31 * result + (this.name.hashCode());
result = 31 * result + (this.platforms.hashCode());
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.workingDirectory != null ? this.workingDirectory.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy