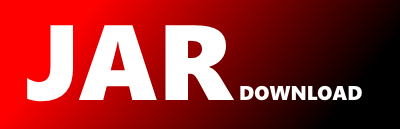
software.amazon.awscdk.services.appstream.CfnAppBlock Maven / Gradle / Ivy
Show all versions of appstream Show documentation
package software.amazon.awscdk.services.appstream;
/**
* A CloudFormation `AWS::AppStream::AppBlock`.
*
* This resource creates an app block. App blocks store details about the virtual hard disk that contains the files for the application in an S3 bucket. It also stores the setup script with details about how to mount the virtual hard disk. App blocks are only supported for Elastic fleets.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnAppBlock cfnAppBlock = CfnAppBlock.Builder.create(this, "MyCfnAppBlock")
* .name("name")
* .setupScriptDetails(ScriptDetailsProperty.builder()
* .executablePath("executablePath")
* .scriptS3Location(S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build())
* .timeoutInSeconds(123)
* // the properties below are optional
* .executableParameters("executableParameters")
* .build())
* .sourceS3Location(S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build())
* // the properties below are optional
* .description("description")
* .displayName("displayName")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.50.0 (build d1830a4)", date = "2022-01-09T19:25:18.623Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnAppBlock")
public class CfnAppBlock extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnAppBlock(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnAppBlock(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.appstream.CfnAppBlock.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::AppStream::AppBlock`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnAppBlock(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnAppBlockProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The ARN of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrArn() {
return software.amazon.jsii.Kernel.get(this, "attrArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The time when the app block was created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreatedTime() {
return software.amazon.jsii.Kernel.get(this, "attrCreatedTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The tags of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The name of the app block.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getName() {
return software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the app block.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "name", java.util.Objects.requireNonNull(value, "name is required"));
}
/**
* The setup script details of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getSetupScriptDetails() {
return software.amazon.jsii.Kernel.get(this, "setupScriptDetails", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The setup script details of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSetupScriptDetails(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "setupScriptDetails", java.util.Objects.requireNonNull(value, "setupScriptDetails is required"));
}
/**
* The setup script details of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSetupScriptDetails(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnAppBlock.ScriptDetailsProperty value) {
software.amazon.jsii.Kernel.set(this, "setupScriptDetails", java.util.Objects.requireNonNull(value, "setupScriptDetails is required"));
}
/**
* The source S3 location of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getSourceS3Location() {
return software.amazon.jsii.Kernel.get(this, "sourceS3Location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The source S3 location of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSourceS3Location(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "sourceS3Location", java.util.Objects.requireNonNull(value, "sourceS3Location is required"));
}
/**
* The source S3 location of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSourceS3Location(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnAppBlock.S3LocationProperty value) {
software.amazon.jsii.Kernel.set(this, "sourceS3Location", java.util.Objects.requireNonNull(value, "sourceS3Location is required"));
}
/**
* The description of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* The display name of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDisplayName() {
return software.amazon.jsii.Kernel.get(this, "displayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The display name of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDisplayName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "displayName", value);
}
/**
* The S3 location of the app block.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* S3LocationProperty s3LocationProperty = S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnAppBlock.S3LocationProperty")
@software.amazon.jsii.Jsii.Proxy(S3LocationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface S3LocationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The S3 bucket of the app block.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getS3Bucket();
/**
* The S3 key of the S3 object of the virtual hard disk.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getS3Key();
/**
* @return a {@link Builder} of {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String s3Bucket;
java.lang.String s3Key;
/**
* Sets the value of {@link S3LocationProperty#getS3Bucket}
* @param s3Bucket The S3 bucket of the app block. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3Bucket(java.lang.String s3Bucket) {
this.s3Bucket = s3Bucket;
return this;
}
/**
* Sets the value of {@link S3LocationProperty#getS3Key}
* @param s3Key The S3 key of the S3 object of the virtual hard disk. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3Key(java.lang.String s3Key) {
this.s3Key = s3Key;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link S3LocationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public S3LocationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements S3LocationProperty {
private final java.lang.String s3Bucket;
private final java.lang.String s3Key;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.s3Bucket = software.amazon.jsii.Kernel.get(this, "s3Bucket", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.s3Key = software.amazon.jsii.Kernel.get(this, "s3Key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.s3Bucket = java.util.Objects.requireNonNull(builder.s3Bucket, "s3Bucket is required");
this.s3Key = java.util.Objects.requireNonNull(builder.s3Key, "s3Key is required");
}
@Override
public final java.lang.String getS3Bucket() {
return this.s3Bucket;
}
@Override
public final java.lang.String getS3Key() {
return this.s3Key;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("s3Bucket", om.valueToTree(this.getS3Bucket()));
data.set("s3Key", om.valueToTree(this.getS3Key()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appstream.CfnAppBlock.S3LocationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
S3LocationProperty.Jsii$Proxy that = (S3LocationProperty.Jsii$Proxy) o;
if (!s3Bucket.equals(that.s3Bucket)) return false;
return this.s3Key.equals(that.s3Key);
}
@Override
public final int hashCode() {
int result = this.s3Bucket.hashCode();
result = 31 * result + (this.s3Key.hashCode());
return result;
}
}
}
/**
* The details of the script.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* ScriptDetailsProperty scriptDetailsProperty = ScriptDetailsProperty.builder()
* .executablePath("executablePath")
* .scriptS3Location(S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build())
* .timeoutInSeconds(123)
* // the properties below are optional
* .executableParameters("executableParameters")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnAppBlock.ScriptDetailsProperty")
@software.amazon.jsii.Jsii.Proxy(ScriptDetailsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ScriptDetailsProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The run path for the script.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getExecutablePath();
/**
* The S3 object location of the script.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getScriptS3Location();
/**
* The run timeout, in seconds, for the script.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getTimeoutInSeconds();
/**
* The parameters used in the run path for the script.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getExecutableParameters() {
return null;
}
/**
* @return a {@link Builder} of {@link ScriptDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ScriptDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String executablePath;
java.lang.Object scriptS3Location;
java.lang.Number timeoutInSeconds;
java.lang.String executableParameters;
/**
* Sets the value of {@link ScriptDetailsProperty#getExecutablePath}
* @param executablePath The run path for the script. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executablePath(java.lang.String executablePath) {
this.executablePath = executablePath;
return this;
}
/**
* Sets the value of {@link ScriptDetailsProperty#getScriptS3Location}
* @param scriptS3Location The S3 object location of the script. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scriptS3Location(software.amazon.awscdk.core.IResolvable scriptS3Location) {
this.scriptS3Location = scriptS3Location;
return this;
}
/**
* Sets the value of {@link ScriptDetailsProperty#getScriptS3Location}
* @param scriptS3Location The S3 object location of the script. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scriptS3Location(software.amazon.awscdk.services.appstream.CfnAppBlock.S3LocationProperty scriptS3Location) {
this.scriptS3Location = scriptS3Location;
return this;
}
/**
* Sets the value of {@link ScriptDetailsProperty#getTimeoutInSeconds}
* @param timeoutInSeconds The run timeout, in seconds, for the script. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timeoutInSeconds(java.lang.Number timeoutInSeconds) {
this.timeoutInSeconds = timeoutInSeconds;
return this;
}
/**
* Sets the value of {@link ScriptDetailsProperty#getExecutableParameters}
* @param executableParameters The parameters used in the run path for the script.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executableParameters(java.lang.String executableParameters) {
this.executableParameters = executableParameters;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ScriptDetailsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ScriptDetailsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ScriptDetailsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ScriptDetailsProperty {
private final java.lang.String executablePath;
private final java.lang.Object scriptS3Location;
private final java.lang.Number timeoutInSeconds;
private final java.lang.String executableParameters;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.executablePath = software.amazon.jsii.Kernel.get(this, "executablePath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.scriptS3Location = software.amazon.jsii.Kernel.get(this, "scriptS3Location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.timeoutInSeconds = software.amazon.jsii.Kernel.get(this, "timeoutInSeconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.executableParameters = software.amazon.jsii.Kernel.get(this, "executableParameters", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.executablePath = java.util.Objects.requireNonNull(builder.executablePath, "executablePath is required");
this.scriptS3Location = java.util.Objects.requireNonNull(builder.scriptS3Location, "scriptS3Location is required");
this.timeoutInSeconds = java.util.Objects.requireNonNull(builder.timeoutInSeconds, "timeoutInSeconds is required");
this.executableParameters = builder.executableParameters;
}
@Override
public final java.lang.String getExecutablePath() {
return this.executablePath;
}
@Override
public final java.lang.Object getScriptS3Location() {
return this.scriptS3Location;
}
@Override
public final java.lang.Number getTimeoutInSeconds() {
return this.timeoutInSeconds;
}
@Override
public final java.lang.String getExecutableParameters() {
return this.executableParameters;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("executablePath", om.valueToTree(this.getExecutablePath()));
data.set("scriptS3Location", om.valueToTree(this.getScriptS3Location()));
data.set("timeoutInSeconds", om.valueToTree(this.getTimeoutInSeconds()));
if (this.getExecutableParameters() != null) {
data.set("executableParameters", om.valueToTree(this.getExecutableParameters()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appstream.CfnAppBlock.ScriptDetailsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ScriptDetailsProperty.Jsii$Proxy that = (ScriptDetailsProperty.Jsii$Proxy) o;
if (!executablePath.equals(that.executablePath)) return false;
if (!scriptS3Location.equals(that.scriptS3Location)) return false;
if (!timeoutInSeconds.equals(that.timeoutInSeconds)) return false;
return this.executableParameters != null ? this.executableParameters.equals(that.executableParameters) : that.executableParameters == null;
}
@Override
public final int hashCode() {
int result = this.executablePath.hashCode();
result = 31 * result + (this.scriptS3Location.hashCode());
result = 31 * result + (this.timeoutInSeconds.hashCode());
result = 31 * result + (this.executableParameters != null ? this.executableParameters.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.appstream.CfnAppBlock}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.appstream.CfnAppBlockProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.appstream.CfnAppBlockProps.Builder();
}
/**
* The name of the app block.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*
* @return {@code this}
* @param name The name of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(final java.lang.String name) {
this.props.name(name);
return this;
}
/**
* The setup script details of the app block.
*
* @return {@code this}
* @param setupScriptDetails The setup script details of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder setupScriptDetails(final software.amazon.awscdk.core.IResolvable setupScriptDetails) {
this.props.setupScriptDetails(setupScriptDetails);
return this;
}
/**
* The setup script details of the app block.
*
* @return {@code this}
* @param setupScriptDetails The setup script details of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder setupScriptDetails(final software.amazon.awscdk.services.appstream.CfnAppBlock.ScriptDetailsProperty setupScriptDetails) {
this.props.setupScriptDetails(setupScriptDetails);
return this;
}
/**
* The source S3 location of the app block.
*
* @return {@code this}
* @param sourceS3Location The source S3 location of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceS3Location(final software.amazon.awscdk.core.IResolvable sourceS3Location) {
this.props.sourceS3Location(sourceS3Location);
return this;
}
/**
* The source S3 location of the app block.
*
* @return {@code this}
* @param sourceS3Location The source S3 location of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceS3Location(final software.amazon.awscdk.services.appstream.CfnAppBlock.S3LocationProperty sourceS3Location) {
this.props.sourceS3Location(sourceS3Location);
return this;
}
/**
* The description of the app block.
*
* @return {@code this}
* @param description The description of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The display name of the app block.
*
* @return {@code this}
* @param displayName The display name of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder displayName(final java.lang.String displayName) {
this.props.displayName(displayName);
return this;
}
/**
* The tags of the app block.
*
* @return {@code this}
* @param tags The tags of the app block. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.appstream.CfnAppBlock}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.appstream.CfnAppBlock build() {
return new software.amazon.awscdk.services.appstream.CfnAppBlock(
this.scope,
this.id,
this.props.build()
);
}
}
}