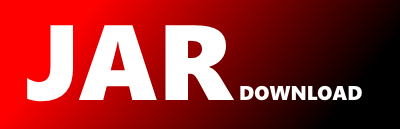
software.amazon.awscdk.services.appstream.CfnApplication Maven / Gradle / Ivy
Show all versions of appstream Show documentation
package software.amazon.awscdk.services.appstream;
/**
* A CloudFormation `AWS::AppStream::Application`.
*
* This resource creates an application. Applications store the details about how to launch applications on streaming instances. This is only supported for Elastic fleets.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnApplication cfnApplication = CfnApplication.Builder.create(this, "MyCfnApplication")
* .appBlockArn("appBlockArn")
* .iconS3Location(S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build())
* .instanceFamilies(List.of("instanceFamilies"))
* .launchPath("launchPath")
* .name("name")
* .platforms(List.of("platforms"))
* // the properties below are optional
* .attributesToDelete(List.of("attributesToDelete"))
* .description("description")
* .displayName("displayName")
* .launchParameters("launchParameters")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .workingDirectory("workingDirectory")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.50.0 (build d1830a4)", date = "2022-01-09T19:25:18.626Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnApplication")
public class CfnApplication extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnApplication(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnApplication(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.appstream.CfnApplication.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::AppStream::Application`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnApplication(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnApplicationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The ARN of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrArn() {
return software.amazon.jsii.Kernel.get(this, "attrArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The time when the application was created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreatedTime() {
return software.amazon.jsii.Kernel.get(this, "attrCreatedTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The tags of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The app block ARN with which the application should be associated.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAppBlockArn() {
return software.amazon.jsii.Kernel.get(this, "appBlockArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The app block ARN with which the application should be associated.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAppBlockArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "appBlockArn", java.util.Objects.requireNonNull(value, "appBlockArn is required"));
}
/**
* The icon S3 location of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getIconS3Location() {
return software.amazon.jsii.Kernel.get(this, "iconS3Location", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* The icon S3 location of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setIconS3Location(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "iconS3Location", java.util.Objects.requireNonNull(value, "iconS3Location is required"));
}
/**
* The icon S3 location of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setIconS3Location(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnApplication.S3LocationProperty value) {
software.amazon.jsii.Kernel.set(this, "iconS3Location", java.util.Objects.requireNonNull(value, "iconS3Location is required"));
}
/**
* The instance families the application supports.
*
* Allowed Values : GENERAL_PURPOSE
| GRAPHICS_G4
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getInstanceFamilies() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "instanceFamilies", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* The instance families the application supports.
*
* Allowed Values : GENERAL_PURPOSE
| GRAPHICS_G4
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setInstanceFamilies(final @org.jetbrains.annotations.NotNull java.util.List value) {
software.amazon.jsii.Kernel.set(this, "instanceFamilies", java.util.Objects.requireNonNull(value, "instanceFamilies is required"));
}
/**
* The launch path of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getLaunchPath() {
return software.amazon.jsii.Kernel.get(this, "launchPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The launch path of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLaunchPath(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "launchPath", java.util.Objects.requireNonNull(value, "launchPath is required"));
}
/**
* The name of the application.
*
* This name is visible to users when a name is not specified in the DisplayName property.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getName() {
return software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the application.
*
* This name is visible to users when a name is not specified in the DisplayName property.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "name", java.util.Objects.requireNonNull(value, "name is required"));
}
/**
* The platforms the application supports.
*
* Allowed Values : WINDOWS_SERVER_2019
| AMAZON_LINUX2
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getPlatforms() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "platforms", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* The platforms the application supports.
*
* Allowed Values : WINDOWS_SERVER_2019
| AMAZON_LINUX2
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlatforms(final @org.jetbrains.annotations.NotNull java.util.List value) {
software.amazon.jsii.Kernel.set(this, "platforms", java.util.Objects.requireNonNull(value, "platforms is required"));
}
/**
* A list of attributes to delete from an application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.util.List getAttributesToDelete() {
return java.util.Optional.ofNullable((java.util.List)(software.amazon.jsii.Kernel.get(this, "attributesToDelete", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))))).map(java.util.Collections::unmodifiableList).orElse(null);
}
/**
* A list of attributes to delete from an application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAttributesToDelete(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "attributesToDelete", value);
}
/**
* The description of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* The display name of the application.
*
* This name is visible to users in the application catalog.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDisplayName() {
return software.amazon.jsii.Kernel.get(this, "displayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The display name of the application.
*
* This name is visible to users in the application catalog.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDisplayName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "displayName", value);
}
/**
* The launch parameters of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getLaunchParameters() {
return software.amazon.jsii.Kernel.get(this, "launchParameters", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The launch parameters of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLaunchParameters(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "launchParameters", value);
}
/**
* The working directory of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getWorkingDirectory() {
return software.amazon.jsii.Kernel.get(this, "workingDirectory", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The working directory of the application.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setWorkingDirectory(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "workingDirectory", value);
}
/**
* The S3 location of the application icon.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* S3LocationProperty s3LocationProperty = S3LocationProperty.builder()
* .s3Bucket("s3Bucket")
* .s3Key("s3Key")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnApplication.S3LocationProperty")
@software.amazon.jsii.Jsii.Proxy(S3LocationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface S3LocationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The S3 bucket of the S3 object.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getS3Bucket();
/**
* The S3 key of the S3 object.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getS3Key();
/**
* @return a {@link Builder} of {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String s3Bucket;
java.lang.String s3Key;
/**
* Sets the value of {@link S3LocationProperty#getS3Bucket}
* @param s3Bucket The S3 bucket of the S3 object. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3Bucket(java.lang.String s3Bucket) {
this.s3Bucket = s3Bucket;
return this;
}
/**
* Sets the value of {@link S3LocationProperty#getS3Key}
* @param s3Key The S3 key of the S3 object. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder s3Key(java.lang.String s3Key) {
this.s3Key = s3Key;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link S3LocationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public S3LocationProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link S3LocationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements S3LocationProperty {
private final java.lang.String s3Bucket;
private final java.lang.String s3Key;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.s3Bucket = software.amazon.jsii.Kernel.get(this, "s3Bucket", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.s3Key = software.amazon.jsii.Kernel.get(this, "s3Key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.s3Bucket = java.util.Objects.requireNonNull(builder.s3Bucket, "s3Bucket is required");
this.s3Key = java.util.Objects.requireNonNull(builder.s3Key, "s3Key is required");
}
@Override
public final java.lang.String getS3Bucket() {
return this.s3Bucket;
}
@Override
public final java.lang.String getS3Key() {
return this.s3Key;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("s3Bucket", om.valueToTree(this.getS3Bucket()));
data.set("s3Key", om.valueToTree(this.getS3Key()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appstream.CfnApplication.S3LocationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
S3LocationProperty.Jsii$Proxy that = (S3LocationProperty.Jsii$Proxy) o;
if (!s3Bucket.equals(that.s3Bucket)) return false;
return this.s3Key.equals(that.s3Key);
}
@Override
public final int hashCode() {
int result = this.s3Bucket.hashCode();
result = 31 * result + (this.s3Key.hashCode());
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.appstream.CfnApplication}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.appstream.CfnApplicationProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.appstream.CfnApplicationProps.Builder();
}
/**
* The app block ARN with which the application should be associated.
*
* @return {@code this}
* @param appBlockArn The app block ARN with which the application should be associated. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appBlockArn(final java.lang.String appBlockArn) {
this.props.appBlockArn(appBlockArn);
return this;
}
/**
* The icon S3 location of the application.
*
* @return {@code this}
* @param iconS3Location The icon S3 location of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iconS3Location(final software.amazon.awscdk.core.IResolvable iconS3Location) {
this.props.iconS3Location(iconS3Location);
return this;
}
/**
* The icon S3 location of the application.
*
* @return {@code this}
* @param iconS3Location The icon S3 location of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iconS3Location(final software.amazon.awscdk.services.appstream.CfnApplication.S3LocationProperty iconS3Location) {
this.props.iconS3Location(iconS3Location);
return this;
}
/**
* The instance families the application supports.
*
* Allowed Values : GENERAL_PURPOSE
| GRAPHICS_G4
*
* @return {@code this}
* @param instanceFamilies The instance families the application supports. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceFamilies(final java.util.List instanceFamilies) {
this.props.instanceFamilies(instanceFamilies);
return this;
}
/**
* The launch path of the application.
*
* @return {@code this}
* @param launchPath The launch path of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder launchPath(final java.lang.String launchPath) {
this.props.launchPath(launchPath);
return this;
}
/**
* The name of the application.
*
* This name is visible to users when a name is not specified in the DisplayName property.
*
* Pattern : ^[a-zA-Z0-9][a-zA-Z0-9_.-]{0,100}$
*
* @return {@code this}
* @param name The name of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(final java.lang.String name) {
this.props.name(name);
return this;
}
/**
* The platforms the application supports.
*
* Allowed Values : WINDOWS_SERVER_2019
| AMAZON_LINUX2
*
* @return {@code this}
* @param platforms The platforms the application supports. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder platforms(final java.util.List platforms) {
this.props.platforms(platforms);
return this;
}
/**
* A list of attributes to delete from an application.
*
* @return {@code this}
* @param attributesToDelete A list of attributes to delete from an application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder attributesToDelete(final java.util.List attributesToDelete) {
this.props.attributesToDelete(attributesToDelete);
return this;
}
/**
* The description of the application.
*
* @return {@code this}
* @param description The description of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The display name of the application.
*
* This name is visible to users in the application catalog.
*
* @return {@code this}
* @param displayName The display name of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder displayName(final java.lang.String displayName) {
this.props.displayName(displayName);
return this;
}
/**
* The launch parameters of the application.
*
* @return {@code this}
* @param launchParameters The launch parameters of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder launchParameters(final java.lang.String launchParameters) {
this.props.launchParameters(launchParameters);
return this;
}
/**
* The tags of the application.
*
* @return {@code this}
* @param tags The tags of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* The working directory of the application.
*
* @return {@code this}
* @param workingDirectory The working directory of the application. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workingDirectory(final java.lang.String workingDirectory) {
this.props.workingDirectory(workingDirectory);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.appstream.CfnApplication}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.appstream.CfnApplication build() {
return new software.amazon.awscdk.services.appstream.CfnApplication(
this.scope,
this.id,
this.props.build()
);
}
}
}